import math
import numpy as np
import matplotlib.pyplot as plt
xy1 = [1,2,2,2]
xy2 = [2,2,2,0,1,2]
xy3 = [2,3,4,5,2,3,4,5]
def get_len(x1,x2,y1,y2):
diff_x = (x1-x2)**2
diff_y = (y1-y2)**2
length = np.sqrt(diff_x+diff_y)
return length
def rotate(angle,valuex,valuey):
valuex = np.array(valuex)
valuey = np.array(valuey)
rotatex = math.cos(angle)*valuex - math.sin(angle)*valuey
rotatey = math.cos(angle)*valuey + math.sin(angle)*valuex
rotatex = rotatex.tolist()
rotatey = rotatey.tolist()
return rotatex, rotatey
x1,y1 = rotate(90,xy1[0:2],xy1[2:4])
x2,y2 = rotate(90,xy2[0:3],xy2[3:6])
x3,y3 = rotate(90,xy3[0:4],xy3[4:8])
############################################
#测试形状是否发生改变
len1 = get_len(xy2[0],xy2[2],xy2[3],xy2[5])
len2 = get_len(x2[0],x2[2],y2[0],y2[2])
print(len1)
print(len2)
#############################################
plt.plot(xy1[0:2],xy1[2:4])
plt.plot(xy2[0:3],xy2[3:6])
plt.plot(xy3[0:4],xy3[4:8])
plt.plot(x1,y1)
plt.plot(x2,y2)
plt.plot(x3,y3)
plt.xlim(-6,6)
plt.ylim(-6,6)
plt.show()
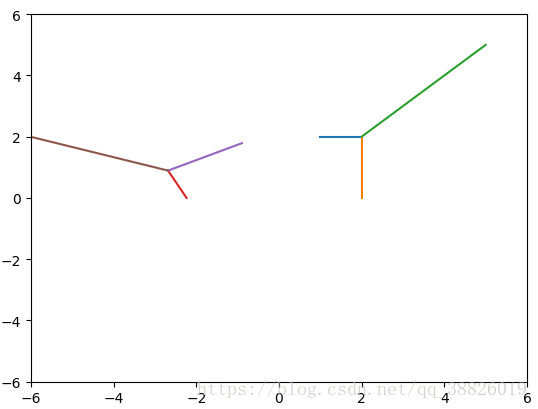
import math
import numpy as np
import matplotlib.pyplot as plt
xy1 = [1,2,2,2]
xy2 = [2,2,2,0,1,2]
xy3 = [2,3,4,5,2,3,4,5]
def get_len(x1,x2,y1,y2):
diff_x = (x1-x2)**2
diff_y = (y1-y2)**2
length = np.sqrt(diff_x+diff_y)
return length
def rotate(angle,valuex,valuey):
valuex = np.array(valuex)
valuey = np.array(valuey)
rotatex = math.cos(angle)*valuex - math.sin(angle)*valuey
rotatey = math.cos(angle)*valuey + math.sin(angle)*valuex
rotatex = rotatex.tolist()
rotatey = rotatey.tolist()
return rotatex, rotatey
x1,y1 = rotate(90,xy1[0:2],xy1[2:4])
x2,y2 = rotate(90,xy2[0:3],xy2[3:6])
x3,y3 = rotate(90,xy3[0:4],xy3[4:8])
x = x1 + x2 + x3
y = y1 + y2 + y3
x1 = np.array(x1)
x2 = np.array(x2)
x3 = np.array(x3)
y1 = np.array(y1)
y2 = np.array(y2)
y3 = np.array(y3)
x1 = x1-min(x)
x2 = x2-min(x)
x3 = x3-min(x)
y1 = y1-min(y)
y2 = y2-min(y)
y3 = y3-min(y)
print(x1)
# ############################################
#测试形状是否发生改变
len1 = get_len(xy2[0],xy2[2],xy2[3],xy2[5])
len2 = get_len(x2[0],x2[2],y2[0],y2[2])
print(len1)
print(len2)
# #############################################
plt.plot(xy1[0:2],xy1[2:4])
plt.plot(xy2[0:3],xy2[3:6])
plt.plot(xy3[0:4],xy3[4:8])
plt.plot(x1,y1)
plt.plot(x2,y2)
plt.plot(x3,y3)
plt.xlim(0,6)
plt.ylim(0,6)
plt.show()
