之前不知道abc怎么和它的全排列做比较,这里使用的方法是通过字符映射到数字,再对数字进行求和。abc和cba的和是一样的。
第一种:滑动窗口
For each window
representing a substring of s2
of length len(s1)
, we want to check if the count of the window is equal to the count of s1
. Here, the count of a string is the list of: [the number of a
's it has, the number of b
's,... , the number of z
's.]
We can maintain the window by deleting the value of s2[i - len(s1)] when it gets larger than len(s1)
. After, we only need to check if it is equal to the target. Working with list values of [0, 1,..., 25] instead of 'a'-'z' makes it easier to count later.
def checkInclusion(self, s1, s2):
A = [ord(x) - ord('a') for x in s1]
B = [ord(x) - ord('a') for x in s2]
target = [0] * 26
for x in A:
target[x] += 1
window = [0] * 26
for i, x in enumerate(B):
window[x] += 1
if i >= len(A):
window[B[i - len(A)]] -= 1
if window == target:
return True
return False
from collections import Counter
class Solution:
def checkInclusion(self, s1, s2):
"""
:type s1: str
:type s2: str
:rtype: bool
"""
d1, d2 = Counter(s1), Counter(s2[:len(s1)])
for start in range(len(s1), len(s2)):
if d1 == d2: return True
d2[s2[start]] += 1
d2[s2[start-len(s1)]] -= 1
if d2[s2[start-len(s1)]] == 0:
del d2[s2[start-len(s1)]]
return d1 == d2
最后一种:
虽然超过时间限制,但是这个思路很简单——用i定位长的字符长,然后取(i+短的字符串)这么多个长度,和短的字符串做比较。
ctr1 = collections.Counter(s1)
i = 0
while i < len(s2) - len(s1) + 1:
if s2[i] in ctr1:
ctr2 = collections.Counter(s2[i: i+len(s1)])
if ctr1 == ctr2: return True
i += 1
return False
补充知识:
key = sum(map(hash, s1))
now = sum(map(hash, s2[:ls1]))
这是干嘛的??(O_O)?
扫描二维码关注公众号,回复:
3940151 查看本文章
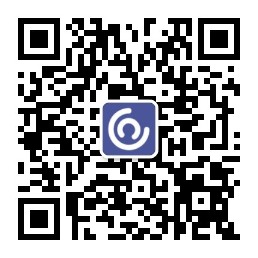