If you want to preview pdf in the winform, you need to make sure Adobe PDF Reader is installed on your computer. Then you can add control "Adobe PDF Reader" by the following steps.
1.Choose "Tools" and click "Choose Toolbox items…"
2.Click "COM Components" and choose "Adobe PDF Reader", then click "OK"
3.Drag "Adobe PDF Reader" to winform
Then try the code:

static byte[] filestream; private void Form1_Load(object sender, EventArgs e) { Image i = Image.FromFile(@"D:\1.png"); filestream = ConvertToBinary(@"D:\a.pdf"); DataGridViewRow dr = new DataGridViewRow(); dr.CreateCells(dataGridView1); dr.Cells[1].Value = i; dr.Cells[2].Value = filestream; dataGridView1.Rows.Add(dr); dataGridView1.AllowUserToAddRows = false; } public static byte[] ConvertToBinary(string Path) { FileStream stream = new FileInfo(Path).OpenRead(); byte[] buffer = new byte[stream.Length]; stream.Read(buffer, 0, Convert.ToInt32(stream.Length)); return buffer; } private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e) { int CIndex = e.ColumnIndex; if (CIndex == 0) { string savepath = @"D:\new.pdf"; if (System.IO.File.Exists(savepath)) { System.IO.File.Delete(savepath); } FileStream fs = new FileStream(savepath, FileMode.CreateNew); BinaryWriter bw = new BinaryWriter(fs); bw.Write(filestream, 0, filestream.Length); bw.Close(); fs.Close(); axAcroPDF.LoadFile(savepath); } }
Otherwise, if you want to preview it with other softwares, such as the browser, modify the code as follows.

private void dataGridView1_CellContentClick(object sender, DataGridViewCellEventArgs e) { int CIndex = e.ColumnIndex; if (CIndex == 0) { string savepath = @"D:\new.pdf"; if (System.IO.File.Exists(savepath)) { System.IO.File.Delete(savepath); } FileStream fs = new FileStream(savepath, FileMode.CreateNew); BinaryWriter bw = new BinaryWriter(fs); bw.Write(filestream, 0, filestream.Length); bw.Close(); fs.Close(); // modify this Process.Start(savepath); } }
The result of winform like as follows,
扫描二维码关注公众号,回复:
4100435 查看本文章
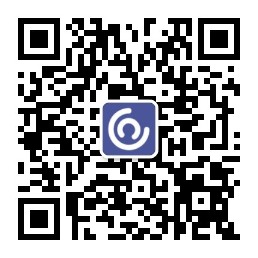