665:Non-decreasing Array
Given an array with n integers, your task is to check if it could become non-decreasing by modifying at most 1 element.
We define an array is non-decreasing if array[i] <= array[i + 1] holds for every i (1 <= i < n).
Example 1:
Input: [4,2,3]
Output: True
Explanation: You could modify the first 4 to 1 to get a non-decreasing array.
Example 2:
Input: [4,2,1]
Output: False
Explanation: You can’t get a non-decreasing array by modify at most one element.
Note: The n belongs to [1, 10,000].
class Solution {
public:
bool checkPossibility(vector<int>& nums) {
int index = -1;
int countnum = 0;
bool flag1;
bool flag2;
for(int i = 0; i<nums.size()-1; i++)
{
if(nums[i+1]<nums[i])
{
if(countnum == 0)
{
index = i + 1;
}
countnum ++;
}
}
if(index == -1)
return true;
else if(countnum>1)
return false;
else
{
if(index+1 > nums.size()-1)
{
flag1 = true;
}
else if(nums[index+1]>=nums[index-1])
{
flag1 = true;
}
else
{
flag1 = false;
}
if(index-2<0)
{
flag2 = true;
}
else if(nums[index]>nums[index-2])
{
flag2 = true;
}
else
{
flag2 = false;
}
}
return flag1||flag2;
}
};
605: Can Place Flowers
Suppose you have a long flowerbed in which some of the plots are planted and some are not. However, flowers cannot be planted in adjacent plots - they would compete for water and both would die.
Given a flowerbed (represented as an array containing 0 and 1, where 0 means empty and 1 means not empty), and a number n, return if n new flowers can be planted in it without violating the no-adjacent-flowers rule.
Example 1:
Input: flowerbed = [1,0,0,0,1], n = 1
Output: True
Example 2:
Input: flowerbed = [1,0,0,0,1], n = 2
Output: False
Note:
The input array won’t violate no-adjacent-flowers rule.
The input array size is in the range of [1, 20000].
n is a non-negative integer which won’t exceed the input array size.
首先需要找到关键点:v[i]==0的点,再根据i点的左右值把数组分为以下四种讨论:
1.[0] i=0
2.[0,0,…] i=0
3.[…0,0] i=size()-1
4.[…0,0,0…] 普通情况
可得出如下代码:
# 605: Can Place Flowers
class Solution {
public:
bool canPlaceFlowers(vector<int>& flowerbed, int n) {
int countnum = 0;
if(n == 0)
return true;
for(int i = 0; i<flowerbed.size(); i++)
{
if(flowerbed[i] == 0)
{
if(i-1 < 0 && i+1 >flowerbed.size()-1) //[0]
{
flowerbed[i] = 1;
countnum++;
}
if(i==flowerbed.size()-1 && i-1 >=0 && flowerbed[i-1] == 0) //i-1存在且v(i-1)==0 [...0,0]
{
flowerbed[i] = 1;
countnum++;
}
if(i==0 && i+1<=flowerbed.size()-1 && flowerbed[i+1] == 0) // [0,0....]
{
flowerbed[i] = 1;
countnum++;
}
if(i-1 >= 0 && i+1 <=flowerbed.size()-1 && flowerbed[i-1] == 0 && flowerbed[i+1] == 0) // [...0,0,0...]
{
flowerbed[i] = 1;
countnum++;
}
}
}
if(countnum>=n)
{
return true;
}
else
{
return false;
}
}
};
实际上,上述1,2,3,三种情况可以通过一些技巧转换未普通情况:当i索引(v[i]==0)位于数组的最左边,最右边或者数组中只有一个元素时,通过人工添加i索引处左值(v[i-1])或者右值(v[i+1])来将其转换为普通情况:
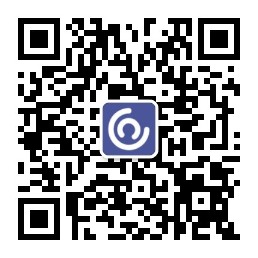
if(flowerbed[i] == 0)
int next = (i == flowerbed.length - 1) ? 0 : flowerbed[i + 1];
int prev = (i == 0) ? 0 : flowerbed[i - 1];
同时在for循环中加上count<n节省时间:
public class Solution {
public boolean canPlaceFlowers(int[] flowerbed, int n) {
int count = 0;
for(int i = 0; i < flowerbed.length && count < n; i++) {
if(flowerbed[i] == 0) {
//get next and prev flower bed slot values. If i lies at the ends the next and prev are considered as 0.
int next = (i == flowerbed.length - 1) ? 0 : flowerbed[i + 1];
int prev = (i == 0) ? 0 : flowerbed[i - 1];
if(next == 0 && prev == 0) {
flowerbed[i] = 1;
count++;
}
}
}
return count == n;
}
}
tip:
①:找关键点,根据关键点来对可能出现的情况进行讨论,比如665中的i与i-1的情况。
②:关键点转换,比如605中,对于数组头与数组尾的情况,通过对pre与next赋值来转换为一般情况