1、注解@Document
1.1、@Document源码
@Persistent
@Inherited
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.TYPE})
public @interface Document {
//索引库名称
String indexName();
//类型
String type() default "";
//
boolean useServerConfiguration() default false;
//默认分片数5
short shards() default 5;
//默认副本数1
short replicas() default 1;
//刷新间隔
String refreshInterval() default "1s";
//索引文件存储类型
String indexStoreType() default "fs";
//是否创建索引
boolean createIndex() default true;
}
1.2、@Document注解使用
@Document注解作用在类上,标记实体类为文档对象,常用属性如下:
(1)indexName:对应索引库名称;
(2)type:对应在索引库中的类型;
(3)shards:分片数
(4)replicas:副本数;
2、注解@Field
2.1、@Field源码
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.FIELD})
@Documented
@Inherited
public @interface Field {
//自动检测属性类型
FieldType type() default FieldType.Auto;
//
boolean index() default true;
//时间类型的字段格式化
DateFormat format() default DateFormat.none;
//
String pattern() default "";
//默认情况下不存储
boolean store() default false;
//
boolean fielddata() default false;
//指定字段使用搜索时的分词
String searchAnalyzer() default "";
//
String analyzer() default "";
//如果某个字段需要被忽略
String[] ignoreFields() default {};
//
boolean includeInParent() default false;
}
2.2、枚举类FieldType
【FieldType源码】
public enum FieldType {
Text,
Integer,
Long,
Date,
Float,
Double,
Boolean,
Object,
Auto,
Nested,
Ip,
Attachment,
Keyword;
private FieldType() {
}
}
2.3、@Field注解使用
@Field作用在成员变量,标记为文档的字段,并制定映射属性;
(1)@Id:作用在成员变量,标记一个字段为id主键;一般id字段或是域不需要存储也不需要分词;
(2)type:字段的类型,取值是枚举,FieldType;
(3)index:是否索引,布尔值类型,默认是true;
扫描二维码关注公众号,回复:
4392987 查看本文章
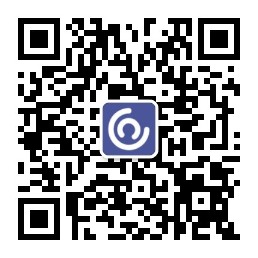
(4)store:是否存储,布尔值类型,默认值是false;
(5)analyzer:分词器名称
3、实体类代码
@Document(indexName = "item",type = "docs",shards = 1,replicas = 0)
public class Item {
@Id
private Long id;
@Field(type = FieldType.Text,analyzer = "ik_max_word")
private String title;
@Field(type=FieldType.Keyword)
private String category;
@Field(type=FieldType.Keyword)
private String brand;
@Field(type=FieldType.Double)
private Double price;
@Field(index = false,type = FieldType.Keyword)
private String images;
}
【注意】(1)@Field(index=true)表示是否索引,如果是索引表示该字段(或者叫域)能能够搜索。
(2)@Field(analyzer="ik_max_word",searchAnalyzer="ik_max_word")表示是否分词,如果是分词就会按照分词的单词搜索,如果不是分词就按照整体搜索。
(3)@Field(store=true)是否存储,也就是页面上显示。