版权声明:本文以学习为主,欢迎转载,但必须在文章页面明显位置给出原文连接。 如果文中有不妥或者错误的地方还望高手的你指出,以免误人子弟。 https://blog.csdn.net/u014116780/article/details/84391900
@梦想橡皮擦 是你的博客自动评论“谢谢博主分享”把我带入了爬虫的世界, 仅以此篇博客表示敬意!
基础知识:
网络爬虫是一种高效地信息采集利器,利用它可以快速、准确地采集互联网上的各种数据资源,几乎已经成为大数据时代IT从业者的必修课。简单点说,网络爬虫就是获取网页并提取和保存信息的自动化过程,分为下列三个步骤:获取网页、提取信息、保存数据。
1.获取网页
使用requests发送GET请求获取网页的源代码。以获取百度为例:
import requests
response = requests.get('https://www.baidu.com')
print(response.text)
2.提取信息
Beautiful Soup是Python的一个HTML或XML解析库,速度快,容错能力强,可以方便、高效地从网页中提取数据。基本用法:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'lxml')
print(soup.prettify())
print(soup.title.string)
Beautiful Soup方法选择器:
find_all()查询符合条件的所有元素,返回所有匹配元素组成的列表。API如下:
find_all(name,attrs,recursive,text,**kwargs)
find()返回第一个匹配的元素。举个栗子:
from bs4 import BeautifulSoup
soup = BeautifulSoup(html, 'lxml')
print(soup.find('div', attrs={'class': 'article-list'}))
3.保存数据
使用Txt文档保存,兼容性好。
扫描二维码关注公众号,回复:
4481572 查看本文章
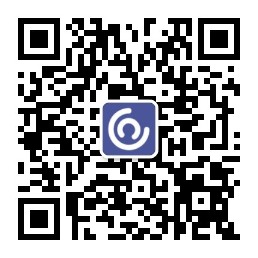
使用with as语法。在with控制块结束的时候,文件自动关闭。举个栗子:
with open(file_name, 'a') as file_object:
file_object.write("I love programming.\n")
file_object.write("I love playing basketball.\n")
分析页面:
要爬取的页面是一个博客页面:https://blog.csdn.net/hihell/article/list/1,这位博主曾在我的博客下面进行评论留言,让我甚是感动。爬取博客,只为学习,并无恶意。
使用Chrome的开发者工具(快捷键F12),看到这个页面的博文列表是一个article-list,下面有许多article-item-box,对应每一篇博文。
每一篇博文下面有各种信息:标题、链接、摘要、发表日期、阅读量、评论量等。
需要做的就是获取到这个页面,然后提取各种信息,转存为txt即可。
编写代码:
获取网页使用requests ,提取信息使用Beautiful Soup,存储使用txt就可以了。
# coding: utf-8
import re
import requests
from bs4 import BeautifulSoup
def get_blog_info():
headers = {'User-Agent': 'Mozilla/5.0 (X11; Linux x86_64) '
'AppleWebKit/537.36 (KHTML, like Gecko) '
'Ubuntu Chromium/44.0.2403.89 '
'Chrome/44.0.2403.89 '
'Safari/537.36'}
html = get_page(blog_url)
soup = BeautifulSoup(html, 'lxml')
article_list = soup.find('div', attrs={'class': 'article-list'})
article_item = article_list.find_all('div', attrs={'class': 'article-item-box csdn-tracking-statistics'})
for ai in article_item:
title = ai.a.text.replace(ai.a.span.text, "")
link = ai.a['href']
date = ai.find('div', attrs={'class': 'info-box d-flex align-content-center'}).span.text
read_num = ai.find('div', attrs={'class': 'info-box d-flex align-content-center'}).find_all('span', attrs={
'class': 'read-num'})[0].text
comment_num = ai.find('div', attrs={'class': 'info-box d-flex align-content-center'}).find_all('span', attrs={
'class': 'read-num'})[1].text
print(title.strip())
print(link)
print(date)
print(read_num)
print(comment_num)
write_to_file(title)
write_to_file(link)
write_to_file('\t' + date)
write_to_file('\t' + read_num)
write_to_file('\t' + comment_num)
def get_page(url):
try:
headers = {'User-Agent': 'Mozilla/5.0 (X11; Linux x86_64) '
'AppleWebKit/537.36 (KHTML, like Gecko) '
'Ubuntu Chromium/44.0.2403.89 '
'Chrome/44.0.2403.89 '
'Safari/537.36'}
response = requests.get(blog_url, headers=headers, timeout=10)
return response.text
except:
return ""
def write_to_file(content):
with open('article.txt', 'a', encoding='utf-8') as f:
f.write(content)
if __name__ == '__main__':
blog_url = "https://blog.csdn.net/hihell/article/list/1"
get_blog_info()