有许多数据类型无法轻松保存到平面文件,例如列表和词典。 如果希望文件是人类可读的,可能希望以巧妙的方式将它们保存为文本文件。JSON适用于Python词典。
但是,如果只想将它们导入Python,则可以序列化它们。 所有这些意味着将对象转换为字节序列或字节流。
1、导入pickle包,从文件中打开以前的pickle数据结构并加载它。
# Import pickle package
import pickle
# Open pickle file and load data: d
with open('data.pkl', 'rb') as file:
d = pickle.load(file)
# Print d
print(d)
# Print datatype of d
print(type(d))
{'Airline': '8', 'Aug': '85', 'Mar': '84.4', 'June': '69.4'}
<class 'dict'>
2、导入Excel电子表格
该excel电子表格包含两个工作表‘2002’和‘2004’
使用pandas导入Excel电子表格以及在任何已加载的.xlsx文件中列出工作表的名称
# Import pandas
import pandas as pd
# Assign spreadsheet filename: file
file = 'battledeath.xlsx'
# Load spreadsheet: xl
xl = pd.ExcelFile(file)
print(xl)
# Print sheet names
print(xl.sheet_names)
<pandas.io.excel.ExcelFile object at 0x7f78e91bb2b0>
['2002', '2004']
将加载的.xlsx文件的任何给定工作表导入为DataFrame。 可以通过指定工作表的名称或索引来完成此操作。
# Load a sheet into a DataFrame by name: df1
df1 = xl.parse('2004')
# Print the head of the DataFrame df1
print(df1.head())
# Load a sheet into a DataFrame by index: df2
df2 = xl.parse(0)
# Print the head of the DataFrame df2
print(df2.head())
War(country) 2004
0 Afghanistan 9.451028
1 Albania 0.130354
2 Algeria 3.407277
3 Andorra 0.000000
4 Angola 2.597931
War, age-adjusted mortality due to 2002
0 Afghanistan 36.083990
1 Albania 0.128908
2 Algeria 18.314120
3 Andorra 0.000000
4 Angola 18.964560
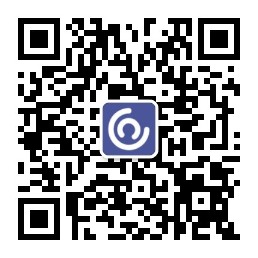
自定义电子表格导入
和以前一样,你将使用方法parse()。 但是,这一次,将添加其他参数skiprows:跳过行,names:命名列,parse_cols:指定要解析的列。 可以根据需要将所有这些参数分配给包含特定行号,字符串和列号的列表
# Parse the first sheet and rename the columns: df1
df1 = xl.parse(0, skiprows=[0], names=['Country','AAM due to War (2002)'])
# Print the head of the DataFrame df1
print(df1.head())
# Parse the first column of the second sheet and rename the column: df2
df2 = xl.parse(1, parse_cols=[0], skiprows=[0], names=['Country'])
# Print the head of the DataFrame df2
print(df2.head())
Country AAM due to War (2002)
0 Albania 0.128908
1 Algeria 18.314120
2 Andorra 0.000000
3 Angola 18.964560
4 Antigua and Barbuda 0.000000
Country
0 Albania
1 Algeria
2 Andorra
3 Angola
4 Antigua and Barbuda
3、导入SAS文件
sas是统计分析文件。用于数据流统计。
import pandas as pd
import matplotlib.pyplot as plt
# Import sas7bdat package
from sas7bdat import SAS7BDAT
# Save file to a DataFrame: df_sas
with SAS7BDAT('sales.sas7bdat') as file:
df_sas = SAS7BDAT.to_data_frame(file)
# Print head of DataFrame
print(df_sas.head())
# Plot histogram of DataFrame features (pandas and pyplot already imported)
pd.DataFrame.hist(df_sas[['P']])
plt.ylabel('count')
plt.show()
YEAR P S
0 1950.0 12.9 181.899994
1 1951.0 11.9 245.000000
2 1952.0 10.7 250.199997
3 1953.0 11.3 265.899994
4 1954.0 11.2 248.500000
4、导入Stata文件
# Import pandas
import pandas as pd
# Load Stata file into a pandas DataFrame: df
df = pd.read_stata('disarea.dta')
# Print the head of the DataFrame df
print(df.head())
# Plot histogram of one column of the DataFrame
pd.DataFrame.hist(df[['disa10']])
plt.xlabel('Extent of disease')
plt.ylabel('Number of countries')
plt.show()
<script.py> output:
wbcode country disa1 disa2 disa3 disa4 disa5 disa6 \
0 AFG Afghanistan 0.00 0.00 0.76 0.73 0.0 0.00
1 AGO Angola 0.32 0.02 0.56 0.00 0.0 0.00
2 ALB Albania 0.00 0.00 0.02 0.00 0.0 0.00
3 ARE United Arab Emirates 0.00 0.00 0.00 0.00 0.0 0.00
4 ARG Argentina 0.00 0.24 0.24 0.00 0.0 0.23
disa7 disa8 ... disa16 disa17 disa18 disa19 disa20 disa21 \
0 0.00 0.0 ... 0.0 0.0 0.0 0.00 0.00 0.0
1 0.56 0.0 ... 0.0 0.4 0.0 0.61 0.00 0.0
2 0.00 0.0 ... 0.0 0.0 0.0 0.00 0.00 0.0
3 0.00 0.0 ... 0.0 0.0 0.0 0.00 0.00 0.0
4 0.00 0.0 ... 0.0 0.0 0.0 0.00 0.05 0.0
disa22 disa23 disa24 disa25
0 0.00 0.02 0.00 0.00
1 0.99 0.98 0.61 0.00
2 0.00 0.00 0.00 0.16
3 0.00 0.00 0.00 0.00
4 0.00 0.01 0.00 0.11
[5 rows x 27 columns]
5、使用h5py导入HDF5文件
# Import packages
import numpy as np
import h5py
# Assign filename: file
file = 'LIGO_data.hdf5'
# Load file: data
data = h5py.File(file, 'r')
# Print the datatype of the loaded file
print(type(data))
# Print the keys of the file
for key in data.keys():
print(key)
<class 'h5py._hl.files.File'>
meta
quality
strain
从HDF5文件中提取数据
# Get the HDF5 group: group
group = data['strain']
# Check out keys of group
for key in group.keys():
print(key)
# Set variable equal to time series data: strain
strain = data['strain']['Strain'].value
# Set number of time points to sample: num_samples
num_samples = 10000
# Set time vector
time = np.arange(0, 1, 1/num_samples)
# Plot data
plt.plot(time, strain[:num_samples])
plt.xlabel('GPS Time (s)')
plt.ylabel('strain')
plt.show()
<script.py> output:
Strain
6、加载.mat文件
# Import package
import scipy.io
import matplotlib.pyplot as plt
import numpy as np
# Load MATLAB file: mat
mat = scipy.io.loadmat('albeck_gene_expression.mat')
# Print the datatype type of mat
print(type(mat))
# Print the keys of the MATLAB dictionary
print(mat.keys())
# Print the type of the value corresponding to the key 'CYratioCyt'
print(type(mat['CYratioCyt']))
# Print the shape of the value corresponding to the key 'CYratioCyt'
print(np.shape(mat['CYratioCyt']))
# Subset the array and plot it
data = mat['CYratioCyt'][25, 5:]
fig = plt.figure()
plt.plot(data)
plt.xlabel('time (min.)')
plt.ylabel('normalized fluorescence (measure of expression)')
plt.show()
<script.py> output:
<class 'dict'>
dict_keys(['__globals__', '__version__', '__header__', 'cfpCyt', 'rfpCyt', 'cfpNuc', 'rfpNuc', 'CYratioCyt', 'yfpCyt', 'yfpNuc'])
<class 'numpy.ndarray'>
(200, 137)