版权声明:欢迎转载请注明转自方辰昱的博客https://blog.csdn.net/viafcccy https://blog.csdn.net/viafcccy/article/details/84888406
strstr_while模型
看这篇https://blog.csdn.net/viafcccy/article/details/84886885
两头堵模型
将一个这种形式的“ zxcv ”求出多少个空格并且去除
这里要用到一个isspace()
若参数c为空格字符,则返回TRUE,否则返回NULL(0)。就是这么简单
思路大概是这样
通过指针从两端遍历达到目的
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
#include <ctype.h>
int getCount(char * str,int * pCount)
{
char *p = str;
int ncount = 0;
int i,j = 0;
i = 0;
j = strlen(p);
while(isspace(p[i]) && p[i] != '\0')
{
i++;
}
while(isspace(p[j-1]) && p[j-1] != '\0')
{
j--;
}
ncount = j - i ;
*pCount = ncount;
return 0;
}
int trimSpace(char * str,char * newstr)
{
char * p = str;
int ncount = 0;
int i,j = 0;
i = 0;
j = strlen(p)-1;
while(isspace(p[i]) && p[i] != '\0')
{
i++;
}
while(isspace(p[j]) && p[j] != '\0')
{
j--;
}
ncount = j - i + 1;
strncpy(newstr , str + i , ncount);
newstr[ncount] = '\0';
return 0;
}
int main()
{
char *p = " abcdefg ";
char buf[1024] = {0};
int num = 0;
getCount(p , &num);
trimSpace(p , buf);
printf("nount:%d\n",num);
printf("buf:%s\n",buf);
system("pause");
return 0;
}
这里又有一个容易宕机的误区就是
扫描二维码关注公众号,回复:
4505681 查看本文章
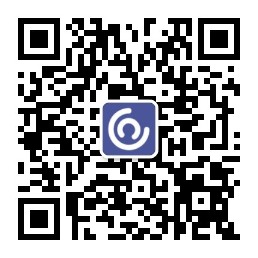
原因是此时对字符串指直接操作 但是字符串是在全局区受到操作系统保护不能通过我们修改 就会宕机
所以要注意 是否可以修改
字符串反转(逆序)
方法1指针
#include<stdio.h>
#include<string.h>
void main()
{
char buf[] = "abcdefg";
int length = strlen(buf);
char *p1 = buf;
char *p2 = buf + length - 1;//指向末尾
while(p1 < p2)
{
char c = *p1;
*p1 = *p2;
*p2 = c;
++p1;//直接自增过的值去执行
--p2;
}
printf("%s\n",buf);
}
进行封装
#include<stdio.h>
#include<string.h>
int inverse(char * buf)
{
char * p = buf;
int length = strlen(p);
char *p1 = p;
char *p2 = p + length - 1;//指向末尾
while(p1 < p2)
{
char c = *p1;
*p1 = *p2;
*p2 = c;
++p1;//直接自增过的值去执行
--p2;
}
return 0;
}
void main()
{
char buf[] = "abcdefg";
inverse(buf);
printf("%s\n",buf);
}
方法2递归逆序
原理就是利用函数参数出入栈的规律
#include<stdio.h>
#include<string.h>
int inverse(char * buf)
{
char *p = buf;
if (p ==NULL)
{
return -1;
}
if(*p == '\0')
{
return 0;
}
inverse(p+1);
printf("%c",*p);
return 0;
}
void main()
{
char buf[] = "abcdefg";
int a;
a = inverse(buf);
if(a != 0)
{
printf("erro:%d",a);
}
}
理解递归调用主要依靠两个模型
1.函数参数出入栈区https://blog.csdn.net/viafcccy/article/details/84583386
2.函数调用模型https://blog.csdn.net/viafcccy/article/details/84673184
memset()函数主要作用就是初始化数组
下面我们来写一个函数利用全局变量储存已经逆序排列的字符串
#include<stdio.h>
#include<string.h>
char g_buf[128];
int inverse(char * buf)
{
char *p = buf;
if (p ==NULL)
{
return -1;
}
if(*p == '\0')
{
return 0;
}
inverse(p+1);
//snprintf()
strncat(g_buf,p,1);
return 0;
}
void main()
{
char buf[] = "abcd";
int a ;
memset(g_buf,0,sizeof(g_buf));
a = inverse(buf);
if(a != 0)
{
printf("erro:%d",a);
}
printf("g_buf:%s",g_buf);
}
但是在多线程编程的时候如果使用全局变量可能会造成资源竞争问题会导致乱序
因此下面使用局部变量进行储存逆序的字符串
#include<stdio.h>
#include<string.h>
char g_buf[128];
int inverse(char * buf,char * bufresult)
{
char *p = buf;
if (p ==NULL||bufresult == NULL)
{
return -1;
}
if(*p == '\0')
{
return 0;
}
inverse(p+1,bufresult);
strncat(bufresult,p,1);
return 0;
}
void main()
{
char buf[] = "abcd";
char mybuf[1024] = {0};
int a ;
a = inverse(buf,mybuf);
if(a != 0)
{
printf("erro:%d",a);
}
printf("g_buf:%s",mybuf);
}
也memset等于这一句
这里也就是警示我们对于使用不完的数组要初始化
但是为什会输出的是512个烫 和 abcddcba
也就是说输出了1024+8个字节已经超过了规定的buf1024个字节
更多的可以看一下这篇帖子 https://bbs.csdn.net/topics/391828185?locationNum=4