百度百科:
Neo4j是一个高性能的,NOSQL图形数据库,它将结构化数据存储在网络上而不是表中。它是一个
嵌入式的、基于
磁盘的、具备完全的事务特性的Java持久化引擎,但是它将结构化数据存储在网络(从数学角度叫做图)上而不是表中。Neo4j也可以被看作是一个高性能的图引擎,该引擎具有成熟数据库的所有特性。程序员工作在一个面向对象的、灵活的网络结构下而不是严格、静态的表中——但是他们可以享受到具备完全的事务特性、企业级的数据库的所有好处。
Neo4j因其嵌入式、高性能、轻量级等优势,越来越受到关注.
查询语句:
不是SQL,而是Cypher
Cypher关键点
- () 表示节点
- [] 表示关系
- {} 表示属性
- > 表示关系的方向
示例:
//查询a的一个叫duchong的朋友 match (a)-[:Friend]->b where b.name ='duchong' return b
一、安装Neo4j
社区版下载地址:
https://neo4j.com/artifact.php?name=neo4j-community-3.4.10-windows.zip
配置环境变量
NEO4J_HOME
Path
%NEO4J_HOME%\bin
配置完成,cmd
neo4j.bat console
启动完成 浏览器访问地址为:
localhost:7474
初始用户名和密码均为neo4j
扫描二维码关注公众号,回复:
4542587 查看本文章
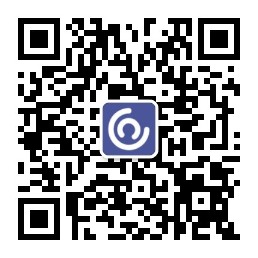
二、springboot整合
添加依赖
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-neo4j</artifactId> </dependency>
properties配置
#neo4j
spring.data.neo4j.uri=http://localhost:7474
spring.data.neo4j.username=neo4j
spring.data.neo4j.password=123qwe
User节点
package com.dc.sb.web.neo4j; import org.neo4j.ogm.annotation.GraphId; import org.neo4j.ogm.annotation.NodeEntity; import org.neo4j.ogm.annotation.Property; /** * 节点实体类型-别名为User * @author DUCHONG * @since 2018-12-17 11:32 **/ @NodeEntity(label = "User") public class UserNode { //图形id @GraphId private Long nodeId; //属性 @Property private String userId; //属性 @Property private String userName; //属性 @Property private int age; public Long getNodeId() { return nodeId; } public void setNodeId(Long nodeId) { this.nodeId = nodeId; } public String getUserId() { return userId; } public void setUserId(String userId) { this.userId = userId; } public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
UserRelation节点
package com.dc.sb.web.neo4j; import org.neo4j.ogm.annotation.EndNode; import org.neo4j.ogm.annotation.GraphId; import org.neo4j.ogm.annotation.RelationshipEntity; import org.neo4j.ogm.annotation.StartNode; /** * 关系节点类型 * @author DUCHONG * @since 2018-12-17 11:39 **/ @RelationshipEntity(type = "UserRelation") public class UserRelation { @GraphId private Long id; //开始节点 @StartNode private UserNode startNode; //结束节点 @EndNode private EndNode endNode; public Long getId() { return id; } public void setId(Long id) { this.id = id; } public UserNode getStartNode() { return startNode; } public void setStartNode(UserNode startNode) { this.startNode = startNode; } public EndNode getEndNode() { return endNode; } public void setEndNode(EndNode endNode) { this.endNode = endNode; } }
UserRepository
package com.dc.sb.web.neo4j.dao; import com.dc.sb.web.neo4j.UserNode; import org.springframework.data.neo4j.annotation.Query; import org.springframework.data.neo4j.repository.GraphRepository; import org.springframework.data.repository.query.Param; import org.springframework.stereotype.Component; import java.util.List; /** * @author DUCHONG * @since 2018-12-17 11:42 **/ @Component public interface UserRepository extends GraphRepository<UserNode> { @Query("MATCH (n: User) RETURN n") List<UserNode> getUserNodeList(); @Query("CREATE (n: User{age:{age},userName:{userName}}) RETURN n") List<UserNode> addUserNodeList(@Param("userName") String userName, @Param("age") int age); }
UserReloationReposiroty
package com.dc.sb.web.neo4j.dao; import com.dc.sb.web.neo4j.UserRelation; import org.springframework.data.neo4j.annotation.Query; import org.springframework.data.neo4j.repository.GraphRepository; import org.springframework.data.repository.query.Param; import org.springframework.stereotype.Component; import java.util.List; /** * 用户关系DO * @author DUCHONG * @since 2018-12-17 13:53 **/ @Component public interface UserRelationRepository extends GraphRepository<UserRelation> { @Query("match p= (n:User)<- [r: UserRelation]-> (n1:User) wheren.userId=(firstUserId) and n1.userId =(secondUserId) return p") List<UserRelation> findUserRelationByEachId (@Param(" firstUserId") String firstUserId, @Param ("secondUserId") String secondUserId) ; @Query ("match(fu:User) ,(su:User) where fu. userId=[firstUserId]and su.userId=[ secondUserId, create p= (fu)- [r:UserRelation]-> (su) return p") List<UserRelation> addUserRelation (@Param(" firstUserId") String firstUserId, @Param("secondUserId") String secondUserId) ; }
Service
package com.dc.sb.web.neo4j.service; import com.dc.sb.web.neo4j.UserNode; import com.dc.sb.web.neo4j.dao.UserRepository; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Service; /** * @author DUCHONG * @since 2018-12-17 14:02 **/ @Service public class Neo4jUserService { @Autowired private UserRepository userRepository; public void addUserNode(UserNode userNode){ userRepository.addUserNodeList(userNode.getUserName(),userNode.getAge()); } }
Controller
package com.dc.sb.web.neo4j.controller; import com.dc.sb.web.neo4j.UserNode; import com.dc.sb.web.neo4j.service.Neo4jUserService; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; /** * @author DUCHONG * @since 2018-12-17 14:14 **/ @RestController public class Neo4jController { @Autowired private Neo4jUserService userService; @RequestMapping("/addUserNode") public String addUserNode(){ UserNode userNode=new UserNode(); userNode.setUserName("duchong"); userNode.setAge(20); userService.addUserNode(userNode); return "add success "; } }
浏览器访问localhost:8080/addUserNode
查看Neo4j 数据库
完整代码已托管到GitHub ---》欢迎fork