Reverse digits of an integer.
Example1: x = 123, return 321
Example2: x = -123, return -321
Here are some good questions to ask before coding. Bonus points for you if you have already thought through this!
If the integer's last digit is 0, what should the output be? ie, cases such as 10, 100.
Did you notice that the reversed integer might overflow? Assume the input is a 32-bit integer, then the reverse of 1000000003 overflows. How should you handle such cases?
For the purpose of this problem, assume that your function returns 0 when the reversed integer overflows.
思路分析:这题比較简单,可是有一个tricky的地方,就是怎样处理溢出的情况。
首先要搞清楚,不管32位机器还是64位机器,java定义int为32位,所以Integer.MAX_VAULE和Integer_MIN_VALUE各自是2^31-1和-2^31,32位把第一位当成符号位。easy得出前面的计算结果(考虑进位的规律或者等比数列求和)。所以这题处理溢出有两个地方要注意,第一对于Integer.MIN_VALUE要单独处理,不要直接取绝对值,否则直接溢出。应该返回0;第二就是要推断是否有
res * 10 + posx % 10 > Integer.MAX_VALUE
可是不能直接这么写,直接这样写从左向右运行code也会溢出,能够换一种写法写成推断是否有
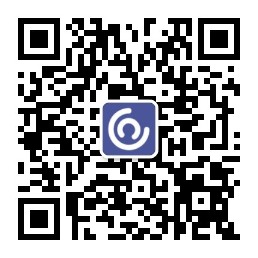
res > (Integer.MAX_VALUE - posx % 10) / 10假设是直接返回0.最后当x位负数时。返回结果的相反数。
AC Code
public class Solution { public int reverse(int x) { //1259 if(x == Integer.MIN_VALUE){ return 0;//Integer.MIN_VALUE is -2^31, the abs of which is larger than Integer.MAX_VALUE(2^31 - 1) } int posx = Math.abs(x); int res = 0; while(posx != 0){ int rem = posx % 10; //see whether res * 10 + posx % 10 > Integer.MAX_VALUE if yes, overflow //note that we move the item except res in the right to the left if(res > (Integer.MAX_VALUE - rem) / 10){ return 0; } res = res * 10 + rem; posx /= 10; } return x>0?res:-res; } }