一、接收参数(postman发送)
1.form表单
@RequestParam("name") String name
会把传递过来的Form表单中的name对应到formData方法的name参数上
该注解不能接收json传参
该注解表示name字段是必须入参的,否则会报错
@RequestParam(value = "name", required = false) String name
required = false表示必须入参
@RequestParam(value = "name", defaultValue = "admin") String name
defaultValue = "admin"表示当name入参为空的时候给它一个默认值admin
/** * 测试接收form表单、URL的数据。不能接收Json数据 * */ @RequestMapping(value = "/test1", method = RequestMethod.POST) public String formData(@RequestParam("name") String name , @RequestParam("age") int age){ String result = "receive name = "+name+" age = "+age; System.out.println(result); return result; }
2.URL
代码跟1.form表单中的代码一样
3.动态接收URL中的数据
@PathVariable将URL中的占位符参数绑定到控制器处理方法的入参
此种情况下,url求情中一定要带占位符pageNo,pageSize的值,不然访问失败
即访问时一定要用 http://localhost:8088/sid/test2/2/20
如果用 http://localhost:8088/sid/test2 则访问失败
/** * 测试动态接收URL中的数据 * */ @RequestMapping(value = "/test2/{pageNo}/{pageSize}", method = RequestMethod.POST) public String urlData(@PathVariable int pageNo , @PathVariable int pageSize){ String result = "receive pageNo = "+pageNo+" pageSize = "+pageSize; System.out.println(result); return result; }
4.json
@RequestBody 接收Json格式的数据需要加这个注解。该注解不能接收URL、Form表单传参
/** * 测试接收json数据 * */ @RequestMapping(value = "/jsonData", method = RequestMethod.POST) public String jsonData(@RequestBody TestModel tm){ String result = "receive name = "+tm.getName()+" age = "+tm.getAge(); System.out.println(result); return result; }
5.@RequestMapping注解详细介绍
1.处理多个URL
@RestController @RequestMapping("/home") public class IndexController { @RequestMapping(value = { "", "/page", "page*", "view/*,**/msg" }) String indexMultipleMapping() { return "Hello from index multiple mapping."; } }
这些 URL 都会由 indexMultipleMapping() 来处理:
localhost:8080/home
localhost:8080/home/
localhost:8080/home/page
localhost:8080/home/pageabc
localhost:8080/home/view/
localhost:8080/home/view/view
2.HTTP的各种方法
如POST方法
@RequestMapping(value = "/test1", method = RequestMethod.POST)
3.produces、consumes
produces 指定返回的内容类型,仅当request请求头header中的(Accept)类型中包含该指定类型才返回。结合@ResponseBody使用
---------------------
---------------------
@Controller @RequestMapping(value = "/t") public class TestController { //方法仅处理request请求中Accept头中包含了"text/html"的请求 @ResponseBody @RequestMapping(value = "/produces",produces = {"text/html"}) public String testProduces(String name) { return "test requestMapping produces attribute! "+name; } }
方法仅处理request请求中Accept头中包含了"text/html"的请求
比如用postman构建一个Accept=“application/json”的请求,请求会失败
扫描二维码关注公众号,回复:
4656293 查看本文章
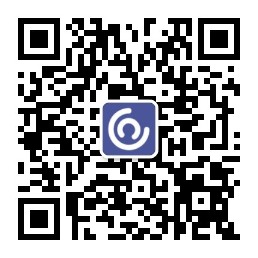
comsumes 指定处理请求的提交内容类型(Content-Type),例如application/json, text/html。结合@RequestBody使用
@Controller @RequestMapping(value = "/t") public class TestController { //方法仅处理request Content-Type为"application/json"类型的请求 @ResponseBody @RequestMapping(value = "/consumes",consumes = {"application/json"}) public String testConsumes(@RequestBody String name) { return "test requestMapping consumes attribute! "+name; } }
方法仅处理request Content-Type为"application/json"类型的请求。
如果用postman构建一个Content-Type=“application/x-www-form-urlencoded”的请求,该方法不处理
4.headers
根据请求中的消息头内容缩小请求映射的范围
例如:
只处理header中testHeader = sid的请求
//方法仅处理header中testHeader = sid的请求 @ResponseBody @RequestMapping(value = "/header",headers = {"testHeader = sid"}) public String testHeader(String name) { return "test requestMapping headers attribute! "+name; }
构建一个header钟不带testHeader=sid的请求,会失败
必须要header中带testHeader=sid的请求的请求才处理
5.结合params属性处理请求参数
例如:
请求参数name=sid的时候由getParams方法处理
请求参数name=lee的时候由getParamsDifferent方法处理
@Controller @RequestMapping(value = "/t") public class TestController { @RequestMapping(value = "/params", params = { "name=sid" }) @ResponseBody public String getParams(@RequestParam("name") String name) { return "getParams method do " + name; } @RequestMapping(value = "/params", params = { "name=lee" }) @ResponseBody public String getParamsDifferent(@RequestParam("name") String name) { return "getParamsDifferent method do " + name; } }