转载自:http://blog.csdn.net/wsl211511/article/details/51605655
Java 反射是 Java 被视为动态(或准动态)语言的一个关键性质。这个机制允许程序在运 行时透过 Reflection APIs 取得任何一个已知名称的class 的内部信息,包括其 modifiers( 诸如 public, static 等 )、superclass (例如 Object)、 实现之 interfaces(例如 Cloneable),也包括 fields 和 methods 的所有信息,并可于运行时改变 fields 内容或唤起 methods。
Java 反射机制容许程序在运行时加载、探知、使用编译期间完全未知的 classes。 换言之,Java 可以加载一个运行时才得知名称的 class,获得其完整结构。
JDK 中提供的 Reflection API
Java 反射相关的 API 在包 java.lang.reflect 中,JDK 1.6.0的 reflect 包如下图:
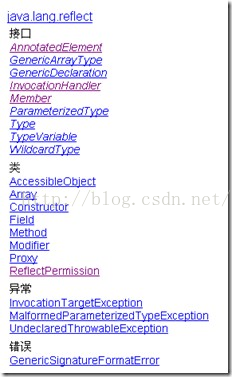
Member 接口 该接口可以获取有关类成员(域或者方法)后者构造函数的信息。
AccessibleObject 类
该类是域(field)对象、方法(method)对象、构造函数(constructor)对象 的基础类。它提供了将反射的对象标记为在使用时取消默认 Java 语言访问控 制检查的能力。
Array 类 提供动态地生成和访问 JAVA 数组的方法。
Constructor 类 提供一个类的构造函数的信息以及访问类的构造函数的接口。
Field 类 提供一个类的域的信息以及访问类的域的接口。
Method 类 提供一个类的方法的信息以及访问类的方法的接口。
Modifier类 提供了 static 方法和常量,对类和成员访问修饰符进行解码。
Proxy 类 提供动态地生成代理类和类实例的静态方法。
JAVA 反射机制提供了什么功能
Java 反射机制提供如下功能: 在运行时判断任意一个对象所属的类 在运行时构造任意一个类的对象
在运行时判段任意一个类所具有的成员变量和方法 在运行时调用任一个对象的方法
在运行时创建新类对象
在使用 Java 的反射功能时,基本首先都要获取类的 Class 对象,再通过 Class 对象获取 其他的对象。
这里首先定义用于测试的类:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<span style= "font-size:18px;" >classType{ publicintpubIntField; publicString pubStringField; privateintprvIntField; publicType(){ Log( "Default Constructor" ); } Type(intarg1, String arg2){ pubIntField = arg1; pubStringField = arg2; 13 Log( "Constructor with parameters" ); 15 } publicvoidsetIntField(intval) { this .prvIntField = val; 19 } publicintgetIntField() { returnprvIntField; } privatevoidLog(String msg){ System.out.println( "Type:" + msg); } }</span> |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<span style= "font-size:18px;" > classExtendTypeextendsType{ publicintpubIntExtendField; publicString pubStringExtendField; privateintprvIntExtendField; <span style= "white-space:pre" > </span>publicExtendType(){ } Log( "Default Constructor" ); } ExtendType(intarg1, String arg2){ pubIntExtendField = arg1; pubStringExtendField = arg2; Log( "Constructor with parameters" ); } publicvoidsetIntExtendField(intfield7) { this .prvIntExtendField = field7; } publicintgetIntExtendField() { returnprvIntExtendField; } privatevoidLog(String msg){ System.out.println( "ExtendType:" + msg); }</span> |
1、获取类的 Class 对象
Class 类的实例表示正在运行的 Java 应用程序中的类和接口。获取类的 Class 对象有多种方式:
调用 Boolean var1 = true; getClass
运 用 .class语法
运用 static method Class.forName()
1 2 |
Class<?> classType2 = var1.getClass(); System.out.println(classType2); |
输出:class java.lang.Boolean
Class<?> classType4 = Boolean.class; System.out.println(classType4);
输出:class java.lang.Boolean
Class<?> classType5 = Class.forName(“java.lang.Boolean”); System.out.println(classType5);
输出:class java.lang.Boolean
运用 Class<?> classType3 = Boolean.TYPE
primitive wrapper classes 的TYPE 语法
这里 返 回 的 是 原 生 类 型 ,和Boolean.class返回的不同
System.out.println(classType3);
输出:boolean
2、获取类的 Fields
可以通过反射机制得到某个类的某个属性,然后改变对应于这个类的某个实例的该属性值。JAVA 的 Class<T>类提供了几个方法获取类的属性。
public Field getField(String name)
返回一个 Field 对象,它反映此 Class 对象所表示的类或接口的指定公共成员字段
public Field[] getFields()
返回一个包含某些 Field 对象的数组,这些对象反映此 Class 对象所表 示的类或接口的所有可访问公共字段
public Field getDeclared Field(String name)
返回一个 Field 对象,该对象反映此 Class 对象所表示的类或接口的指定已声明字段
public Field[] getDeclared Fields()
返回 Field 对象的一个数组,这些对象反映此 Class 对象所表示的类或 接口所声明的所有字段
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 |
<span style= "font-size:18px;" >Class<?> classType = ExtendType. class ; // 使用 getFields 获取属性 Field[] fields = classType.getFields(); for (Field f : fields) { System.out.println(f); } System.out.println(); // 使用 getDeclaredFields 获取属性 fields = classType.getDeclaredFie lds(); for (Field f : fields) { System.out.println(f); } </span> |
输出:
public int com.quincy.ExtendType.pubIntExtendField
public java.lang.String com.quincy.ExtendType.pubStringExtendField public int com.quincy.Type.pubIntField
public java.lang.String com.quincy.Type.pubStringField
public int com.quincy.ExtendType.pubIntExtendField
public java.lang.String com.quincy.ExtendType.pubStringExtendField private int com.quincy.ExtendType.prvIntExtendField
可见 getFields和 getDeclaredFields区别:
1 2 3 4 5 6 7 8 |
<span style= "font-size:18px;" > public MethodgetMethod(String name, Class<?>... parameterTy pes) public Method[] getMethods( ) public MethodgetDeclared Method(Stringname,Class< ?>... parameterTy pes) public Method[] getDeclared Methods()</span> |
getFields返回的是申明为 public的属性,包括父类中定义,
getDeclaredFields返回的是指定类定义的所有定义的属性,不包括父类的。
3、获取类的 Method
通过反射机制得到某个类的某个方法,然后调用对应于这个类的某个实例的该方法
Class<T>类提供了几个方法获取类的方法。
1 2 3 4 5 6 7 |
<span style= "font-size:18px;" > public MethodgetMethod(String name, Class<?>... parameterTy pes) public Method[] getMethods( ) public MethodgetDeclared Method(Stringname,Class< ?>... parameterTy pes) public Method[] getDeclared Methods()</span> |
返回一个 Method 对象,它反映此 Class 对象所表示的类或接口的指定公共成员方法;
返回一个包含某些 Method 对象的数组,这些对象反映此Class 对象所表 示的类或接口(包括那些由该类或接口声明的以及从超类和超接口继承的那 些的类或接口)的公共 member 方法;
返回一个 Method 对象,该对象反映此Class 对象所表示的类或接口的指 定已声明方法;
返回 Method对象的一个数组,这些对象反映此 Class 对象表示的类或接 口声明的所有方法,包括公共、保护、默认(包)访问和私有方法,但不包 括继承的方法。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<span style= "font-size:18px;" > // 使用 getMethods 获取函数 Class<?> classType = ExtendType. class ; Method[] methods = classType.getMethods(); for (Method m : methods) 5 { System.out.println(m); } System.out.println(); // 使用 getDeclaredMethods 获取函数 methods = classType.getDeclaredMethods(); for (Method m : methods) { System.out.println(m); }</span> |
输出:
public void com.quincy.ExtendType.setIntExtendField(int) public int com.quincy.ExtendType.getIntExtendField() public void com.quincy.Type.setIntField(int)
public int com.quincy.Type.getIntField()
public final native void java.lang.Object.wait(long) throws java.lang.InterruptedException
public final void java.lang.Object.wait() throws java.lang.InterruptedException
public final void java.lang.Object.wait(long,int) throws java.lang.InterruptedException
public boolean java.lang.Object.equals(java.lang.Object) public java.lang.String java.lang.Object.toString() public native int java.lang.Object.hashCode()
public final native java.lang.Class java.lang.Object.getClass() public final native void java.lang.Object.notify()
public final native void java.lang.Object.notifyAll() private void com.quincy.ExtendType.Log(java.lang.String) public void com.quincy.ExtendType.setIntExtendField(int) public int com.quincy.ExtendType.getIntExtendField()
4、获取类的 Constructor
通过反射机制得到某个类的构造器,然后调用该构造器创建该类的一个实例Class<T>类提供了几个方法获取类的构造器。
public Constructor< T>
返回一个 Constructor 对象,它反映此 Class对象所表示的类的指定 公共构造方法
1 2 3 4 5 6 7 |
<span style= "font-size:18px;" >getConstruct or(Class<?>. .. parameterTyp es) public Constructor< ?>[] getConstruct ors()</span> |
返回一个包含某些 Constructor 对象的数组,这些对象反映此 Class
对象所表示的类的所有公共构造方法
1 2 3 |
<span style= "font-size:18px;" > public Constructor< T> getDeclaredC onstructor(Class<?>... parameterTyp es)</span> |
返回一个 Constructor 对象,该对象反映此 Class对象所表示的类或 接口的指定构造方法
1 2 3 4 5 |
<span style= "font-size:18px;" > public Constructor< ?>[] getDeclaredC onstructors( )</span> |
返回 Constructor 对象的一个数组,这些对象反映此 Class 对象表示 的类声明的所有构造方法。它们是公共、保护、默认(包)访问和私有构造方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<span style= "font-size:18px;" > // 使用 getConstructors 获取构造器 Constructor<?>[] constructors = classType.getConstructors(); for (Constructor<?> m : constructors){ System.out.println(m); } System.out.println(); // 使用 getDeclaredConstructors 获取构造器 constructors = classType.getDeclaredConstructors(); for (Constructor<?> m : constructors) { System.out.println(m); } 输出: publiccom.quincy.ExtendType() publiccom.quincy.ExtendType() com.quincy.ExtendType( int ,java.lang.String)<span style= "font-family: Arial, Helvetica, sans-serif; background-color: rgb(255, 255, 255);" > </span></span> |
5、新建类的实例
通过反射机制创建新类的实例,有几种方法可以创建新类的实例,调用无自变量
①、调用类的 Class对象的 newInstance方法,该方法会调用对象的默认ctor构造器,如果没有默认构造器,会调用失败.
1 |
<span style= "font-size:18px;" >Class<?> classType = ExtendType. class ; Object inst = classType.newInstance(); System.out.println(inst);</span> |
输出:
Type:Default Constructor ExtendType:Default Constructor com.quincy.ExtendType@d80be3
②、调用默认 Constructor 对象的 newInstance 方法
1 2 3 |
<span style= "font-size:18px;" >Class<?> classType = ExtendType. class ; Constructor<?> constructor1 = classType.getConstructor(); Object inst = constructor1.newInstance(); System.out.println(inst);</span> |
输出:
Type:Default Constructor ExtendType:Default Constructor com.quincy.ExtendType@1006d75
③、调用带参数 Constructor 对象的 newInstance 方法
1 2 3 |
<span style= "font-size:18px;" >Constructor<?> constructor2 = classType.getDeclaredConstructor( int . class , String. class ); Object inst = constructor2.newInstance( 1 , "123" ); System.out.println(inst);</span> |
输出:
Type:Default Constructor ExtendType:Constructor with parameters com.quincy.ExtendType@15e83f9
6、调用类的函数
通过反射获取类 Method 对象,调用 Field 的 Invoke 方法调用函数。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
<span style= "font-size:18px;" >Class<?> classType = ExtendType. class ; Object inst = classType.newInstance(); Method logMethod = classType.<STRONG>getDeclaredMethod</STRONG>( "Log" , String. class ); logMethod.invoke(inst, "test" ); 输出: Type:Default Constructor ExtendType:Default Constructor <FONT color=#ff0000>Class com.quincy.ClassT can not accessa member ofclasscom.quincy.ExtendType with modifiers "private" </FONT> <FONT color=#ff0000>上面失败是由于没有权限调用 private 函数,这里需要设置 Accessible 为 true ;</FONT> Class<?> classType = ExtendType. class ; Object inst = classType.newInstance(); Method logMethod = classType.getDeclaredMethod( "Log" , String. class ); <FONT color=#ff0000>logMethod.setAccessible( true );</FONT> logMethod.invoke(inst, "test" );</span> |
7、设置/获取类的属性值
通过反射获取类的 Field 对象,调用 Field 方法设置或获取值
1 2 3 4 5 6 7 |
<span style= "font-size:18px;" >Class<?> classType = ExtendType. class ; Object inst = classType.newInstance(); Field intField = classType.getField( "pubIntExtendField" ); intField.<STRONG>setInt</STRONG>(inst, 100 ); intvalue = intField.<STRONG>getInt</STRONG>(inst); </span> |
动态创建代理类
代理模式:代理模式的作用=为其他对象提供一种代理以控制对这个对象的访问。 代理模式的角色:
抽象角色:声明真实对象和代理对象的共同接口 代理角色:代理角色内部包含有真实对象的引用,从而可以操作真实对象。真实角色:代理角色所代表的真实对象,是我们最终要引用的对象。 动态代理:
java.lang.re flect.Proxy
Proxy提供用于创建动态代理类和实例的静态方法,它还是由这些方法创建 的所有动态代理类的超InvocationHandler是代理实例的调用处理程序 实现的接口,每个代理实例都具有一个关联的调用处理程序。对代理实例调用方法时,将对方法调用进行编码并将其指派到 它的调用处理程序的 invoke 方法。
动态 Proxy 是这样的一种类:
它是在运行生成的类,在生成时你必须提供一组 Interface给它,然后该 class 就宣称 它实现了这些interface。你可以把该 class 的实例当作这些 interface 中的任何一个 来用。当然,这个 DynamicProxy其实就是一个 Proxy,它不会替你作实质性的工作,在生成它的实例时你必须提供一个 handler,由它接管实际的工作。
在使用动态代理类时,我们必须实现 InvocationHandler接口
步骤:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 |
<span style= "font-size:18px;" > //1、定义抽象角色 public interface Subject { public void Request(); } //2、定义真实角色 public class RealSubject implements Subject { @Override public void Request() { // TODO Auto-generated method stub System.out.println("RealSubject"); } } //3、定义代理角色 public class DynamicSubject implements InvocationHandler { private Objectsub; public DynamicSubject(Object obj){ this .sub = obj; } @Override public Object invoke(Object proxy, Method method, Object[] args) throws Throwable{ // TODO Auto-generated method stub System.out.println("Method:"+ method + ",Args:" + args); method.invoke(sub, args); return null ; } } //4、通过 Proxy.newProxyInstance 构建代理对象 RealSubject realSub = new RealSubject(); InvocationHandler handler = new DynamicSubject(realSub); Class<?> classType = handler.getClass(); Subject sub = (Subject)Proxy.newProxyInstance(classType.getClassLoader(), realSub.getClass().getInterfaces(), handler); System.out.println(sub.getClass()); //5、通过调用代理对象的方法去调用真实角色的方法。 sub.Request();</span> |
输出:
class $Proxy0 新建的代理对象,它实现指定的接口
Method:public abstract void DynamicProxy.Subject.Request(),Args:null
RealSubject 调用的真实对象的方法
本篇文章依旧采用小demo来说明,因为我始终觉的,案例驱动是最好的,要不然只看理论的话,看了也不懂,不过建议大家在看完文章之后,在回过头去看看理论,会有更好的理解。
下面开始正文。
【案例1】通过一个对象获得完整的包名和类名
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
<span style= "font-size:18px;" >packageReflect; /** * 通过一个对象获得完整的包名和类名 * */ classDemo{ //other codes... } classhello{ publicstaticvoidmain(String[] args) { Demo demo=newDemo(); System.out.println(demo.getClass().getName()); } } </span> |
【运行结果】:Reflect.Demo
添加一句:所有类的对象其实都是Class的实例。
【案例2】实例化Class类对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
<span style= "font-size:18px;" >packageReflect; classDemo{ //other codes... } classhello{ publicstaticvoidmain(String[] args) { Class<?> demo1= null ; Class<?> demo2= null ; Class<?> demo3= null ; try { //一般尽量采用这种形式 demo1=Class.forName( "Reflect.Demo" ); } catch (Exception e){ e.printStackTrace(); } demo2=newDemo().getClass(); demo3=Demo. class ; System.out.println( "类名称 " +demo1.getName()); System.out.println( "类名称 " +demo2.getName()); System.out.println( "类名称 " +demo3.getName()); } } </span> |
【运行结果】:
类名称 Reflect.Demo
类名称 Reflect.Demo
类名称 Reflect.Demo
【案例3】通过Class实例化其他类的对象 通过无参构造实例化对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<span style= "font-size:18px;" > package Reflect; class Person{ public String getName(){ return name; } public void setName(String name) { this .name = name; } public int getAge() { return age; } public void setAge(intage) { this .age = age; } @Override public String toString(){ return "[" + this .name+ " " + this .age+ "]" ; } private String name; private int age; } class hello{ public static void main(String[] args) { } Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } Person per= null ; try { per=(Person)demo.newInstance(); } catch (InstantiationException e) { // TODO Auto-generated catch block e.printStackTrace(); } catch (IllegalAccessException e) { // TODO Auto-generated catch block e.printStackTrace(); } per.setName( "Rollen" ); per.setAge( 20 ); System.out.println(per); }</span> |
【运行结果】:
[Rollen20]
但是注意一下,当我们把 Person中的默认的无参构造函数取消的时候,比如自己定义只定义一个有参数 的构造函数之后,会出现错误:
比如我定义了一个构造函数:
1 2 3 4 |
<span style= "font-size:18px;" > public Person(String name,intage) { this .age=age; this .name=name; }</span> |
然后继续运行上面的程序,会出现:
1 2 3 4 5 6 |
<span style= "font-size:18px;" >java.lang.InstantiationException:Reflect.Person atjava.lang.Class.newInstance0(Class.java: 340 ) atjava.lang.Class.newInstance(Class.java: 308 ) atReflect.hello.main(hello.java: 39 ) Exceptioninthread "main" java.lang.NullPointerException atReflect.hello.main(hello.java: 47 )</span> |
所以大家以后再编写使用 Class 实例化其他类的对象的时候,一定要自己定义无参的构造函数
【案例】通过 Class 调用其他类中的构造函数 (也可以通过这种方式通过 Class 创建其他类的对象)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
<span style= "font-size:18px;" > package Reflect; import java.lang.reflect.Constructor; class Person{ public Person() { } publicPerson(String name){ this .name=name; } public Person(intage){ this .age=age; } public Person(String name,intage) { } this .age=age; this .name=name; } publicString getName() { returnname; } publicintgetAge() { returnage; } @Override publicString toString(){ return "[" + this .name+ " " + this .age+ "]" ; } private String name; private int age; class hello{ public static void main(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } Person per1= null ; Person per2= null ; Person per3= null ; Person per4= null ; //取得全部的构造函数 Constructor<?> cons[]=demo.getConstructors(); try { per1=(Person)cons[ 0 ].newInstance(); per2=(Person)cons[ 1 ].newInstance( "Rollen" ); per3=(Person)cons[ 2 ].newInstance( 20 ); per4=(Person)cons[ 3 ].newInstance( "Rollen" , 20 ); } catch (Exception e){ e.printStackTrace(); } System.out.println(per1); System.out.println(per2); System.out.println(per3); System.out.println(per4); }</span> |
【运行结果】:
[null0]
[Rollen0]
[null20]
[Rollen20]
【案例】 返回一个类实现的接口:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 |
<span style= "font-size:18px;" > package Reflect; interfaceChina{ publicstaticfinalString name= "Rollen" ; publicstatic int age= 20 ; publicvoidsayChina(); publicvoidsayHello(String name,intage); } class Person implements China{ public Person() { } publicPerson(String sex){ this .sex=sex; } publicString getSex(){ returnsex; } publicvoidsetSex(String sex) { this .sex = sex; 22 } @Override publicvoidsayChina(){ System.out.println( "hello ,china" ); } @Override publicvoidsayHello(String name,intage){ System.out.println(name+ " " +age); } privateString sex; } class hello{ publicstaticvoidmain(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } } //保存所有的接口 Class<?> intes[]=demo.getInterfaces(); for (inti = 0 ; i < intes.length; i++) { System.out.println( "实现的接口 " +intes[i].getName()); } }</span> |
【运行结果】:
实现的接口 Reflect.China
(注意,以下几个例子,都会用到这个例子的 Person类,所以为节省篇幅,此处不再粘贴 Person的代 码部分,只粘贴主类 hello的代码)
【案例】:取得其他类中的父类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<span style= "font-size:18px;" > class hello{ public static void main(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } //取得父类 Class<?> temp=demo.getSuperclass(); System.out.println( "继承的父类为: " +temp.getName()); } } </span> |
【运行结果】
继承的父类为: java.lang.Object
【案例】:获得其他类中的全部构造函数 这个例子需要在程序开头添加importjava.lang.reflect.*;然后将主类编写为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
<span style= "font-size:18px;" > class hello{ public static void main(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } Constructor<?>cons[]=demo.getConstructors(); for (inti = 0 ; i < cons.length; i++) { System.out.println( "构造方法: " +cons[i]); } } }</span> |
【运行结果】:
构造方法:public Reflect.Person()
构造方法:publicReflect.Person(java.lang.String)
但是细心的读者会发现,上面的构造函数没有 public或者 private这一类的修饰符下面这个例子我们就来获取修饰符
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<span style= "font-size:18px;" > class hello{ //构造方法 } publicstaticvoidmain(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } Constructor<?>cons[]=demo.getConstructors(); for (inti = 0 ; i < cons.length; i++) { Class<?> p[]=cons[i].getParameterTypes(); System.out.print( "构造方法: " ); intmo=cons[i].getModifiers(); System.out.print(Modifier.toString(mo)+ " " ); System.out.print(cons[i].getName()); System.out.print( "(" ); for (intj= 0 ;j<p.length;++j){ System.out.print(p[j].getName()+ " arg" +i); if (j<p.length- 1 ){ System.out.print( "," ); } } System.out.println( "){}" ); } }</span> |
【运行结果】:
构造方法: publicReflect.Person(){}
构造方法: publicReflect.Person(java.lang.Stringarg1){}
有时候一个方法可能还有异常。下面看看:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
<span style= "font-size:18px;" > class hello{ publicstaticvoidmain(String[] args) { Class<?> demo= null ; try { demo=Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } Method method[]=demo.getMethods(); for (inti= 0 ;i<method.length;++i){ Class<?> returnType=method[i].getReturnType(); Class<?> para[]=method[i].getParameterTypes(); inttemp=method[i].getModifiers(); System.out.print(Modifier.toString(temp)+ " " ); System.out.print(returnType.getName()+ " " ); System.out.print(method[i].getName()+ " " ); System.out.print( "(" ); for (intj= 0 ;j<para.length;++j){ System.out.print(para[j].getName()+ " " + "arg" +j); if (j<para.length- 1 ){ System.out.print( "," ); } } Class<?> exce[]=method[i].getExceptionTypes(); if (exce.length> 0 ){ System.out.print( ") throws " ); for (intk= 0 ;k<exce.length;++k){ System.out.print(exce[k].getName()+ " " ); if (k<exce.length- 1 ){ System.out.print( "," ); } } } } else { System.out.print( ")" ) } System.out.println(); } }</span> |
【运行结果】:
1 2 3 4 5 6 7 8 9 10 |
<span style= "font-size:18px;" > public java.lang.StringgetSex() public void setSex(java.lang.Stringarg0)publicvoidsayChina () public void sayHello (java.lang.String arg0, int arg1) public final native void wait ( long arg0) throwsjava.lang.InterruptedException public final void wait()throwsjava.lang.InterruptedException public final void wait(longarg0,intarg1)throwsjava.lang.InterruptedException public boolean equals(java.lang.Objectarg0)publicjava.lang.StringtoString() public native int hashCode() public final native java.lang.ClassgetClass () public final native void notify () public final native void notifyAll()</span> |
【案例】接下来让我们取得其他类的全部属性吧,最后我讲这些整理在一起,也就是通过class取得一个 类的全部框架
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
<span style= "font-size:18px;" > class hello { public static void main(String[] args) { Class<?> demo = null ; try { demo = Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } System.out.println("=============== 本 类 属 性 ========================"); // 取得本类的全部属性 Field[] field = demo.getDeclaredFields(); for ( int i = 0 ; i < field.length; i++) { 14 // 权限修饰符 int mo = field[i].getModifiers(); String priv = Modifier.toString(mo); // 属性类型 Class<?> type = field[i].getType(); System.out.println(priv + " " +type.getName() + " " + field[i].getName() + ";" ); 21 } System.out.println("=============== 实 现 的 接 口 或 者 父 类 的 属 性 ========================"); // 取得实现的接口或者父类的属性 Field[] filed1 = demo.getFields(); for (intj = 0 ; j < filed1.length; j++) { 27 // 权限修饰符 int mo = filed1[j].getModifiers(); String priv = Modifier.toString(mo); // 属性类型 Class<?> type = filed1[j].getType(); System.out.println(priv + " " +type.getName() + " " + filed1[j].getName() + ";" ) } }</span> |
【运行结果】:
===============本类属性========================
private java.lang.String sex;
===============实现的接口或者父类的属性========================
public static final java.lang.String name; public static final int age;
【案例】其实还可以通过反射调用其他类中的方法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
<span style= "font-size:18px;" > class hello { public static void main(String[] args) { Class<?> demo = null ; try { demo = Class.forName( "Reflect.Person" ); } catch (Exception e) { e.printStackTrace(); } try { //调用 Person 类中的 sayChina 方法 Method method=demo.getMethod( "sayChina" ); method.invoke(demo.newInstance()); //调用 Person 的 sayHello 方法 method=demo.getMethod( "sayHello" , String. class , int . class ); } } catch (Exception e) { e.printStackTrace(); } }</span> |
【运行结果】:
hello,chinaRollen20
动态代理
【案例】首先来看看如何获得类加载器:
1 2 3 4 5 6 7 8 |
<span style= "font-size:18px;" > class test{ } class hello{ public static void main(String[] args) { test t=newtest(); System.out.println( "类加载器" +t.getClass().getClassLoader().getClass().getName()); } }</span> |
【程序输出】:
类加载器 sun.misc.Launcher$AppClassLoader
其实在java中有三种类类加载器。
1)BootstrapClassLoader此加载器采用c++编写,一般开发中很少见。
2)ExtensionClassLoader用来进行扩展类的加载,一般对应的是jre\lib\ext目录中的类3)AppClassLoader 加载 classpath 指定的类,是最常用的加载器。同时也是java 中默认的加载器。 如果想要完成动态代理,首先需要定义一个 InvocationHandler接口的子类,已完成代理的具体操作。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
<span style= "font-size:18px;" > package Reflect; import java.lang.reflect.*; //定义项目接口 interface Subject { public String say(String name,intage); 7 } // 定义真实项目 class RealSubject implements Subject { } @Override public String say(String name,intage) { returnname + " " + age; } class MyInvocationHandler implements InvocationHandler { private Object obj = null ; public Object bind(Object obj) { this .obj = obj; return Proxy.newProxyInstance(obj.getClass().getClassLoader(), } .getClass().getInterfaces(), this ); } @Override public Object invoke(Object proxy, Method method,Object[] args) throwsThrowable{ Object temp = method.invoke( this .obj, args); return temp; } class hello { public static void main(String[] args) { MyInvocationHandler demo =newMyInvocationHandler(); Subject sub = (Subject)demo.bind(newRealSubject()); String info = sub.say( "Rollen" , 20 ); System.out.println(info); } }</span> |
【运行结果】: Rollen20
类的生命周期
在一个类编译完成之后,下一步就需要开始使用类,如果要使用一个类,肯定离不开 JVM。在程序执行中JVM 通过装载,链接,初始化这3个步骤完成。
类的装载是通过类加载器完成的,加载器将.class文件的二进制文件装入JVM的方法区,并且在堆区创 建描述这个类的 java.lang.Class 对象。用来封装数据。但是同一个类只会被类装载器装载以前
链接就是把二进制数据组装为可以运行的状态。
链接分为校验,准备,解析这3个阶段校验一般用来确认此二进制文件是否适合当前的 JVM(版本),准备就是为静态成员分配内存空间,。并设置默认值
解析指的是转换常量池中的代码作为直接引用的过程,直到所有的符号引用都可以被运行程序使用(建立完整的对应关系)
完成之后,类型也就完成了初始化,初始化之后类的对象就可以正常使用了,直到一个对象不再使用之后,将被垃圾回收。释放空间。
当没有任何引用指向Class对象时就会被卸载,结束类的生命周期
将反射用于工厂模式 先来看看,如果不用反射的时候,的工厂模式吧:
http://www.cnblogs.com/rollenholt/archive/2011/08/18/2144851.html
反射的用途
Reflection is commonly used by programs which require the ability to examine or modify the runtime behavior of applications running in the Java virtual machine.This is a relatively advanced feature and should be used only bydevelopers who have a strong graspof the fundamentals of the language.With that caveat in mind, reflection isa powerful technique and can enableapplications toperform operations which would otherwise be impossible.
反射被广泛地用于那些需要在运行时检测或修改程序行为的程序中。这是一个相对高级
的特性,只有那些语言基础非常扎实的开发者才应该使用它。如果能把这句警示时刻放在心里,那么反射机制就会成为一项强大的技术,可以让应用程序做一些几乎不可能做到的事情。
反射的缺点
Reflection is powerful, but should not be used indiscriminately. If it is possible to performan operation without using reflection, then it ispreferable to avoid using it. The following concerns should be kept in mind when accessing code via reflection.
尽管反射非常强大,但也不能滥用。如果一个功能可以不用反射完成,那么最好就不用。
在我们使用反射技术时,下面几条内容应该牢记于心:
性能第一
Because reflection involves types that are dynamically resolved, certain Java virtual machine optimizations can not be performed.Consequently, reflective operations have slower performance than their non-reflective counterparts, and should be avoided in sections of code which are called frequently inperformance-sensitive applications.
反射包括了一些动态类型,所以 JVM 无法对这些代码进行优化。因此,反射操作的效
率要比那些非反射操作低得多。我们应该避免在经常被 执行的代码或对性能要求很高的程 序中使用反射。
安全限制
Reflection requires a runtime permission which may not be presentwhen running under a security manager. This is in an important consideration for code which has to run in a restrictedsecurity context, such as in an Applet.
使用反射技术要求程序必须在一个没有安全限制的环境中运行。如果一个程序必须在有
安全限制的环境中运行,如 Applet,那么这就是个问题了。
内部暴露
Since reflection allows code to perform operations that would be illegal in non-reflective code, such as accessingprivatefields and methods, the use of reflection can result in
unexpected side-effects, which may render code dysfunctional and may destroy portability. Reflective code breaks abstractions and thereforemay change behavior with upgradesof the platform.
由于反射允许代码执行一些在正常情况下不被允许的操作(比如访问私有的属性和方
法),所以使用反射可能会导致意料之外的副作用--代码有功能上的错误,降低可移植性。反射代码破坏了抽象性,因此当平台发生改变的时候,代码的行为就有可能也随着变化。
业务思想
JAVA中反射机制很实用。在不断地项目实战中,大家或许才体会到反射的真正强大之处,从很多小的demo中,个人在学习阶段时,几乎没啥感觉,就是个面熟。参考很多资料,个人的总结不到之处,欢迎交流指正。