2018-12-4更新
增添了memset函数对标记数组mark重置
---------------------------------------------------------------------------------------------------------------------------------------------------
- 说明
- 基于邻接表实现的图
一、说明
1、以下代码实现的图,顶点为0到n-1(n为顶点数)(整数),边权为大于0的整数。有向图。
2、isEdge除了有判断是否存在边的作用外,还有定位的作用。
3、注意各文件包含相应的头文件。
4、以下代码仅供参考。
二、基于邻接表实现的图
1、Link.h
2、List.h
3、LList.h
4、LList.cpp
参考实验一 线性表的物理实现(顺序表+单向链表)
5、Queue.h
6、AQueue.h
7、AQueue.cpp
参考队列的物理实现(顺序队列+链式队列)
扫描二维码关注公众号,回复:
4993149 查看本文章
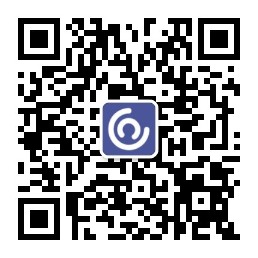
8、Edge.h
#include<iostream>
using namespace std;
#ifndef _Edge
#define _Edge
namespace wangzhe
{
class Edge
{
private:
int vert,wt;//顶点、权值
public:
Edge()
{
vert=-1,wt=-1;
}
Edge(int v,int w)
{
vert=v,wt=w;
}
int vertex()
{
return vert;
}
int weight()
{
return wt;
}
};
}
#endif
9、Graph.h
参考实验五 图的物理实现(邻接矩阵)
10、Graphl.h
#include<iostream>
using namespace std;
#include"Graph.h"
#include"Edge.h"
#include"List.h"
#ifndef _Graphl
#define _Graphl
namespace wangzhe
{
class Graphl:public Graph
{
private:
List<Edge>** vertex;//表头集合
int numVertex,numEdge;//顶点数,边数
int *mark;//标记数组
public:
Graphl();
~Graphl();
void Init(int n);
void clear();
int n();
int e();
int first(int v);
int next(int v,int w);
bool setEdge(int v1,int v2,int wght);
bool delEdge(int v1,int v2);
bool isEdge(int i,int j);
int weight(int v1,int v2);
int getMark(int v);
void setMark(int v,int val);
void memset();//重置mark数组
void BFS(Graph* G,int start,Queue<int>* Q);
void DFS(Graph *G,int v);
void print();
};
}
#endif
11、Graphl.cpp
#include<iostream>
#define UNVISITED 0
#define VISITED 1
using namespace std;
#include"Graphl.h"
#include"Edge.h"
#include"LList.h"
#include"LList.cpp"
namespace wangzhe
{
Graphl::Graphl()
{
}
Graphl::~Graphl()
{
clear();
}
void Graphl::Init(int n)
{
numVertex=n;
numEdge=0;
mark=new int[n];
for(int i=0;i<n;i++) mark[i]=UNVISITED;
vertex=(List<Edge>**) new List<Edge>*[n];
for(int i=0;i<n;i++) vertex[i]=new LList<Edge>(1010);
}
void Graphl::clear()
{
delete [] mark;
mark=NULL;
for(int i=0;i<numVertex;i++)
delete [] vertex[i];
delete vertex;
vertex=NULL;
}
int Graphl::n()
{
return numVertex;
}
int Graphl::e()
{
return numEdge;
}
int Graphl::first(int v)
{
if(vertex[v]->length()==0)
return numVertex;
vertex[v]->moveToStart();
Edge it=vertex[v]->getValue();
return it.vertex();
}
int Graphl::next(int v,int w)
{
if(isEdge(v,w))//定位
if(vertex[v]->currPos()+1<vertex[v]->length())
{
vertex[v]->next();
Edge it=vertex[v]->getValue();
return it.vertex();
}
return n();
}
bool Graphl::setEdge(int v1,int v2,int wght)
{
if(v1<0||v2<0||v1>=numVertex||v2>=numVertex||v1==v2)
{
cout<<"Illegal vertex\n";
return false;
}
if(wght<=0)
{
cout<<"Illegal weight\n";
return false;
}
Edge currEdge(v2,wght);
if(isEdge(v1,v2))
{
vertex[v1]->remove();
vertex[v1]->insert(currEdge);
}
else
{
numEdge++;
for(vertex[v1]->moveToStart();
vertex[v1]->currPos()<vertex[v1]->length();
vertex[v1]->next())
{
Edge temp=vertex[v1]->getValue();
if(temp.vertex()>v2) break;
}
vertex[v1]->insert(currEdge);
}
return true;
}
bool Graphl::delEdge(int v1,int v2)
{
if(v1<0||v2<0||v1>=numVertex||v2>=numVertex||v1==v2)
{
cout<<"Illegal vertex\n";
return false;
}
if(isEdge(v1,v2))
{
vertex[v1]->remove();
numEdge--;
}
return true;
}
bool Graphl::isEdge(int i,int j)
{
for(vertex[i]->moveToStart();vertex[i]->currPos()<vertex[i]->length();
vertex[i]->next())
{
Edge temp=vertex[i]->getValue();
if(temp.vertex()==j) return true;
}
return false;
}
int Graphl::weight(int v1,int v2)
{
Edge curr;
if(isEdge(v1,v2))
{
curr=vertex[v1]->getValue();
return curr.weight();
}
else return 0;
}
int Graphl::getMark(int v)
{
return mark[v];
}
void Graphl::setMark(int v,int val)
{
mark[v]=val;
}
void Graphm::memset()
{
for(int i=0;i<numVertex;i++)
mark[i]=UNVISITED;
}
void Graphl::BFS(Graph* G,int start,Queue<int>* Q)
{
Q->enqueue(start);
G->setMark(start,VISITED);
while(Q->length())
{
int v=Q->dequeue();
cout<<v<<' ';//previsit
for(int w=G->first(v);w<G->n();w=G->next(v,w))
{
if(G->getMark(w)==UNVISITED)
{
G->setMark(w,VISITED);
Q->enqueue(w);
}
}
}
}
void Graphl::DFS(Graph* G,int v)
{
cout<<v<<' ';//previsit
G->setMark(v,VISITED);
for(int w=G->first(v);w<G->n();w=G->next(v,w))
{
if(G->getMark(w)==UNVISITED)
DFS(G,w);
}
}
void Graphl::print()
{
for(int i=0;i<numVertex;i++)
{
cout<<i<<' ';
for(vertex[i]->moveToStart();
vertex[i]->currPos()<vertex[i]->length();
vertex[i]->next())
{
Edge it=vertex[i]->getValue();
cout<<"->"<<it.vertex()<<' ';
}
cout<<endl;
}
}
}
12、main.cpp
参考实验五 图的物理实现(邻接矩阵)