easy
101. Symmetric Tree
Given a binary tree, check whether it is a mirror of itself (ie,
symmetric around its center).
Note: Bonus points if you could solve it both recursively and
iteratively.
Recursive and non-recursive solutions in Java:
Recursive–400ms:
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public boolean isSymmetric(TreeNode root) {
return root==null || isSymmetricHelp(root.left, root.right);
}
private boolean isSymmetricHelp(TreeNode left, TreeNode right){
if(left==null || right==null)
return left==right;
if(left.val!=right.val)
return false;
return isSymmetricHelp(left.left, right.right) && isSymmetricHelp(left.right, right.left);
}
}
Non-recursive(use Stack)–460ms:
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public boolean isSymmetric(TreeNode root) {
if(root==null) return true;
Stack<TreeNode> stack = new Stack<TreeNode>();
TreeNode left, right;
if(root.left!=null){
if(root.right==null) return false;
stack.push(root.left);
stack.push(root.right);
}
else if(root.right!=null){
return false;
}
while(!stack.empty()){
if(stack.size()%2!=0) return false;
right = stack.pop();
left = stack.pop();
if(right.val!=left.val) return false;
if(left.left!=null){
if(right.right==null) return false;
stack.push(left.left);
stack.push(right.right);
}
else if(right.right!=null){
return false;
}
if(left.right!=null){
if(right.left==null) return false;
stack.push(left.right);
stack.push(right.left);
}
else if(right.left!=null){
return false;
}
}
return true;
}
}
1ms recursive Java Solution, easy to understand:
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public boolean isSymmetric(TreeNode root) {
if(root==null) return true;
return isMirror(root.left,root.right);
}
public boolean isMirror(TreeNode p, TreeNode q) {
if(p==null && q==null) return true;
if(p==null || q==null) return false;
return (p.val==q.val) && isMirror(p.left,q.right) && isMirror(p.right,q.left);
}
}
medium
102. Binary Tree Level Order Traversal
Given a binary tree, return the level order traversal of its nodes’ values. (ie, from left to right, level by level).
Java solution with a queue used:
public class Solution {
public List<List<Integer>> levelOrder(TreeNode root) {
Queue<TreeNode> queue = new LinkedList<TreeNode>();
List<List<Integer>> wrapList = new LinkedList<List<Integer>>();
if(root == null) return wrapList;
queue.offer(root);
while(!queue.isEmpty()){
int levelNum = queue.size();
List<Integer> subList = new LinkedList<Integer>();
for(int i=0; i<levelNum; i++) {
if(queue.peek().left != null) queue.offer(queue.peek().left);
if(queue.peek().right != null) queue.offer(queue.peek().right);
subList.add(queue.poll().val);
}
wrapList.add(subList);
}
return wrapList;
}
}
Nothing special. Just wanna provide a different way from BFS.:
class Solution {
public List<List<Integer>> levelOrder(TreeNode root) {
List<List<Integer>> res = new ArrayList<List<Integer>>();
levelHelper(res, root, 0);
return res;
}
public void levelHelper(List<List<Integer>> res, TreeNode root, int height) {
if (root == null) return;
if (height >= res.size()) {
res.add(new LinkedList<Integer>());
}
res.get(height).add(root.val);
levelHelper(res, root.left, height+1);
levelHelper(res, root.right, height+1);
}
}
200. Number of Islands
Given a 2d grid map of '1’s (land) and '0’s (water), count the number of islands. An island is surrounded by water and is formed by connecting adjacent lands horizontally or vertically. You may assume all four edges of the grid are all surrounded by water.
Very concise Java AC solution:
public class Solution {
private int n;
private int m;
public int numIslands(char[][] grid) {
int count = 0;
n = grid.length;
if (n == 0) return 0;
m = grid[0].length;
for (int i = 0; i < n; i++){
for (int j = 0; j < m; j++)
if (grid[i][j] == '1') {
DFSMarking(grid, i, j);
++count;
}
}
return count;
}
private void DFSMarking(char[][] grid, int i, int j) {
if (i < 0 || j < 0 || i >= n || j >= m || grid[i][j] != '1') return;
grid[i][j] = '0';
DFSMarking(grid, i + 1, j);
DFSMarking(grid, i - 1, j);
DFSMarking(grid, i, j + 1);
DFSMarking(grid, i, j - 1);
}
}
207. Course Schedule
There are a total of n courses you have to take, labeled from 0 to n-1.
Some courses may have prerequisites, for example to take course 0 you have to first take course 1, which is expressed as a pair: [0,1]
Given the total number of courses and a list of prerequisite pairs, is it possible for you to finish all courses?
Note:
- The input prerequisites is a graph represented by a list of edges, not adjacency matrices. Read more about how a graph is represented.
- You may assume that there are no duplicate edges in the input prerequisites.
Easy BFS Topological sort, Java:
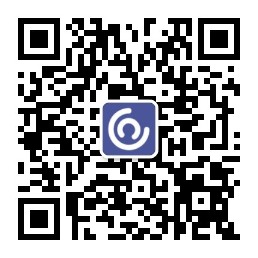
class Solution {
public boolean canFinish(int numCourses, int[][] prerequisites) {
int[][] matrix = new int[numCourses][numCourses]; // i -> j
int[] indegree = new int[numCourses];
for (int i=0; i<prerequisites.length; i++) {
int ready = prerequisites[i][0];
int pre = prerequisites[i][1];
if (matrix[pre][ready] == 0)
indegree[ready]++; //duplicate case
matrix[pre][ready] = 1;
}
int count = 0;
Queue<Integer> queue = new LinkedList();
for (int i=0; i<indegree.length; i++) {
if (indegree[i] == 0) queue.offer(i);
}
while (!queue.isEmpty()) {
int course = queue.poll();
count++;
for (int i=0; i<numCourses; i++) {
if (matrix[course][i] != 0) {
if (--indegree[i] == 0)
queue.offer(i);
}
}
}
return count == numCourses;
}
}
279. Perfect Squares
Given a positive integer n, find the least number of perfect square numbers (for example, 1, 4, 9, 16, …) which sum to n.
Summary of 4 different solutions (BFS, DP, static DP and mathematics):
1.Dynamic Programming: 440ms
class Solution {
public int numSquares(int n) {
if (n <= 0)
{
return 0;
}
// cntPerfectSquares[i] = the least number of perfect square numbers
// which sum to i. Note that cntPerfectSquares[0] is 0.
vector<int> cntPerfectSquares(n + 1, INT_MAX);
cntPerfectSquares[0] = 0;
for (int i = 1; i <= n; i++)
{
// For each i, it must be the sum of some number (i - j*j) and
// a perfect square number (j*j).
for (int j = 1; j*j <= i; j++)
{
cntPerfectSquares[i] =
min(cntPerfectSquares[i], cntPerfectSquares[i - j*j] + 1);
}
}
return cntPerfectSquares.back();
}
}
2.Static Dynamic Programming: 12ms
class Solution {
public int numSquares(int n) {
if (n <= 0)
{
return 0;
}
// cntPerfectSquares[i] = the least number of perfect square numbers
// which sum to i. Since cntPerfectSquares is a static vector, if
// cntPerfectSquares.size() > n, we have already calculated the result
// during previous function calls and we can just return the result now.
static vector<int> cntPerfectSquares({0});
// While cntPerfectSquares.size() <= n, we need to incrementally
// calculate the next result until we get the result for n.
while (cntPerfectSquares.size() <= n)
{
int m = cntPerfectSquares.size();
int cntSquares = INT_MAX;
for (int i = 1; i*i <= m; i++)
{
cntSquares = min(cntSquares, cntPerfectSquares[m - i*i] + 1);
}
cntPerfectSquares.push_back(cntSquares);
}
return cntPerfectSquares[n];
}
}
3.Mathematical Solution: 4ms
class Solution {
private int is_square(int n)
{
int sqrt_n = (int)(sqrt(n));
return (sqrt_n*sqrt_n == n);
}
// Based on Lagrange's Four Square theorem, there
// are only 4 possible results: 1, 2, 3, 4.
public int numSquares(int n)
{
// If n is a perfect square, return 1.
if(is_square(n))
{
return 1;
}
// The result is 4 if and only if n can be written in the
// form of 4^k*(8*m + 7). Please refer to
// Legendre's three-square theorem.
while ((n & 3) == 0) // n%4 == 0
{
n >>= 2;
}
if ((n & 7) == 7) // n%8 == 7
{
return 4;
}
// Check whether 2 is the result.
int sqrt_n = (int)(sqrt(n));
for(int i = 1; i <= sqrt_n; i++)
{
if (is_square(n - i*i))
{
return 2;
}
}
return 3;
}
}
4.Breadth-First Search: 80ms
class Solution {
public int numSquares(int n) {
if (n <= 0)
{
return 0;
}
// perfectSquares contain all perfect square numbers which
// are smaller than or equal to n.
vector<int> perfectSquares;
// cntPerfectSquares[i - 1] = the least number of perfect
// square numbers which sum to i.
vector<int> cntPerfectSquares(n);
// Get all the perfect square numbers which are smaller than
// or equal to n.
for (int i = 1; i*i <= n; i++)
{
perfectSquares.push_back(i*i);
cntPerfectSquares[i*i - 1] = 1;
}
// If n is a perfect square number, return 1 immediately.
if (perfectSquares.back() == n)
{
return 1;
}
// Consider a graph which consists of number 0, 1,...,n as
// its nodes. Node j is connected to node i via an edge if
// and only if either j = i + (a perfect square number) or
// i = j + (a perfect square number). Starting from node 0,
// do the breadth-first search. If we reach node n at step
// m, then the least number of perfect square numbers which
// sum to n is m. Here since we have already obtained the
// perfect square numbers, we have actually finished the
// search at step 1.
queue<int> searchQ;
for (auto& i : perfectSquares)
{
searchQ.push(i);
}
int currCntPerfectSquares = 1;
while (!searchQ.empty())
{
currCntPerfectSquares++;
int searchQSize = searchQ.size();
for (int i = 0; i < searchQSize; i++)
{
int tmp = searchQ.front();
// Check the neighbors of node tmp which are the sum
// of tmp and a perfect square number.
for (auto& j : perfectSquares)
{
if (tmp + j == n)
{
// We have reached node n.
return currCntPerfectSquares;
}
else if ((tmp + j < n) && (cntPerfectSquares[tmp + j - 1] == 0))
{
// If cntPerfectSquares[tmp + j - 1] > 0, this is not
// the first time that we visit this node and we should
// skip the node (tmp + j).
cntPerfectSquares[tmp + j - 1] = currCntPerfectSquares;
searchQ.push(tmp + j);
}
else if (tmp + j > n)
{
// We don't need to consider the nodes which are greater ]
// than n.
break;
}
}
searchQ.pop();
}
}
return 0;
}
}