前言
断言是写自动化测试基本最重要的一步,一个用例没有断言,就失去了自动化测试的意义了。什么是断言呢?
简单来讲就是实际结果和期望结果去对比,符合预期那就测试pass,不符合预期那就测试 failed
assert
pytest允许您使用标准Python断言来验证Python测试中的期望和值。例如,你可以写下
# content of test_assert1.py
def f(): return 3 def test_function(): assert f() == 4
断言f()函数的返回值,接下来会看到断言失败,因为返回的值是3,判断等于4,所以失败了
$ pytest test_assert1.py
=========================== test session starts ============================
platform linux -- Python 3.x.y, pytest-3.x.y, py-1.x.y, pluggy-0.x.y
rootdir: $REGENDOC_TMPDIR, inifile:
collected 1 item
test_assert1.py F [100%]
================================= FAILURES =================================
______________________________ test_function _______________________________ def test_function(): > assert f() == 4 E assert 3 == 4 E + where 3 = f() test_assert1.py:5: AssertionError ========================= 1 failed in 0.12 seconds =========================
从报错信息可以看到断言失败原因:E assert 3 == 4
异常信息
接下来再看一个案例,如果想在异常的时候,输出一些提示信息,这样报错后,就方便查看是什么原因了
def f():
return 3 def test_function(): a = f() assert a % 2 == 0, "判断a为偶数,当前a的值为:%s"%a
运行结果
================================== FAILURES ===================================
________________________________ test_function ________________________________ def test_function(): a = f() > assert a % 2 == 0, "判断a为偶数,当前a的值为:%s"%a E AssertionError: 判断a为偶数,当前a的值为:3 E assert (3 % 2) == 0 test_03.py:9: AssertionError ========================== 1 failed in 0.18 seconds ===========================
这样当断言失败的时候,会给出自己写的失败原因了E AssertionError: 判断a为偶数,当前a的值为:3
异常断言
为了写关于引发异常的断言,可以使用pytest.raises作为上下文管理器,如下
# content of test_assert1.py
import pytest
def test_zero_division(): with pytest.raises(ZeroDivisionError): 1 / 0
运行结果
============================= test session starts =============================
platform win32 -- Python 3.6.0, pytest-3.6.3, py-1.5.4, pluggy-0.6.0 rootdir: D:\YOYO\canshuhua, inifile: plugins: metadata-1.7.0, html-1.19.0 collected 1 item test_assert1.py. ========================== 1 passed in 0.31 seconds ===========================
如果我们要断言它抛的异常是不是预期的,比如执行:1/0,预期结果是抛异常:ZeroDivisionError: division by zero,那我们要断言这个异常,通常是断言异常的type和value值了。
这里1/0的异常类型是ZeroDivisionError,异常的value值是division by zero,于是用例可以这样设计
# content of test_assert1.py
import pytest
def test_zero_division(): '''断言异常''' with pytest.raises(ZeroDivisionError) as excinfo: 1 / 0 # 断言异常类型type assert excinfo.type == ZeroDivisionError # 断言异常value值 assert "division by zero" in str(excinfo.value)
excinfo 是一个异常信息实例,它是围绕实际引发的异常的包装器。主要属性是.type、 .value 和 .traceback
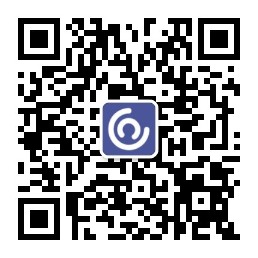
注意:断言type的时候,异常类型是不需要加引号的,断言value值的时候需转str
在上下文管理器窗体中,可以使用关键字参数消息指定自定义失败消息:
with pytest.raises(ZeroDivisionError, message="Expecting ZeroDivisionError"):
pass
结果:Failed: Expecting ZeroDivisionError
常用断言
pytest里面断言实际上就是python里面的assert断言方法,常用的有以下几种
- assert xx 判断xx为真
- assert not xx 判断xx不为真
- assert a in b 判断b包含a
- assert a == b 判断a等于b
- assert a != b 判断a不等于b
import pytest
def is_true(a): if a > 0: return True else: return False def test_01(): '''断言xx为真''' a = 5 b = -1 assert is_true(a) assert not is_true(b) def test_02(): '''断言b 包含 a''' a = "hello" b = "hello world" assert a in b def test_03(): '''断言相等''' a = "yoyo" b = "yoyo" assert a == b def test_04(): '''断言不等于''' a = 5 b = 6 assert a != b if __name__ == "__main__": pytest.main(["-s", "test_01.py"])
前言
断言是写自动化测试基本最重要的一步,一个用例没有断言,就失去了自动化测试的意义了。什么是断言呢?
简单来讲就是实际结果和期望结果去对比,符合预期那就测试pass,不符合预期那就测试 failed
assert
pytest允许您使用标准Python断言来验证Python测试中的期望和值。例如,你可以写下
# content of test_assert1.py
def f(): return 3 def test_function(): assert f() == 4
断言f()函数的返回值,接下来会看到断言失败,因为返回的值是3,判断等于4,所以失败了
$ pytest test_assert1.py
=========================== test session starts ============================
platform linux -- Python 3.x.y, pytest-3.x.y, py-1.x.y, pluggy-0.x.y
rootdir: $REGENDOC_TMPDIR, inifile:
collected 1 item
test_assert1.py F [100%]
================================= FAILURES =================================
______________________________ test_function _______________________________ def test_function(): > assert f() == 4 E assert 3 == 4 E + where 3 = f() test_assert1.py:5: AssertionError ========================= 1 failed in 0.12 seconds =========================
从报错信息可以看到断言失败原因:E assert 3 == 4
异常信息
接下来再看一个案例,如果想在异常的时候,输出一些提示信息,这样报错后,就方便查看是什么原因了
def f():
return 3 def test_function(): a = f() assert a % 2 == 0, "判断a为偶数,当前a的值为:%s"%a
运行结果
================================== FAILURES ===================================
________________________________ test_function ________________________________ def test_function(): a = f() > assert a % 2 == 0, "判断a为偶数,当前a的值为:%s"%a E AssertionError: 判断a为偶数,当前a的值为:3 E assert (3 % 2) == 0 test_03.py:9: AssertionError ========================== 1 failed in 0.18 seconds ===========================
这样当断言失败的时候,会给出自己写的失败原因了E AssertionError: 判断a为偶数,当前a的值为:3
异常断言
为了写关于引发异常的断言,可以使用pytest.raises作为上下文管理器,如下
# content of test_assert1.py
import pytest
def test_zero_division(): with pytest.raises(ZeroDivisionError): 1 / 0
运行结果
============================= test session starts =============================
platform win32 -- Python 3.6.0, pytest-3.6.3, py-1.5.4, pluggy-0.6.0 rootdir: D:\YOYO\canshuhua, inifile: plugins: metadata-1.7.0, html-1.19.0 collected 1 item test_assert1.py. ========================== 1 passed in 0.31 seconds ===========================
如果我们要断言它抛的异常是不是预期的,比如执行:1/0,预期结果是抛异常:ZeroDivisionError: division by zero,那我们要断言这个异常,通常是断言异常的type和value值了。
这里1/0的异常类型是ZeroDivisionError,异常的value值是division by zero,于是用例可以这样设计
# content of test_assert1.py
import pytest
def test_zero_division(): '''断言异常''' with pytest.raises(ZeroDivisionError) as excinfo: 1 / 0 # 断言异常类型type assert excinfo.type == ZeroDivisionError # 断言异常value值 assert "division by zero" in str(excinfo.value)
excinfo 是一个异常信息实例,它是围绕实际引发的异常的包装器。主要属性是.type、 .value 和 .traceback
注意:断言type的时候,异常类型是不需要加引号的,断言value值的时候需转str
在上下文管理器窗体中,可以使用关键字参数消息指定自定义失败消息:
with pytest.raises(ZeroDivisionError, message="Expecting ZeroDivisionError"):
pass
结果:Failed: Expecting ZeroDivisionError
常用断言
pytest里面断言实际上就是python里面的assert断言方法,常用的有以下几种
- assert xx 判断xx为真
- assert not xx 判断xx不为真
- assert a in b 判断b包含a
- assert a == b 判断a等于b
- assert a != b 判断a不等于b
import pytest
def is_true(a): if a > 0: return True else: return False def test_01(): '''断言xx为真''' a = 5 b = -1 assert is_true(a) assert not is_true(b) def test_02(): '''断言b 包含 a''' a = "hello" b = "hello world" assert a in b def test_03(): '''断言相等''' a = "yoyo" b = "yoyo" assert a == b def test_04(): '''断言不等于''' a = 5 b = 6 assert a != b if __name__ == "__main__": pytest.main(["-s", "test_01.py"])