刚刚写了一个链表的头插法和尾插法函数,测试后发现先用头插法插入10 个数 从1到10 打印链表长为10,
尾插法在插入5个数后在打印链表长为5,我一想不对啊,应该是15 才对啊,原来头插法和尾插法各创建了一个链表,头插法的链表在尾插法开始后在内存中丢失。因为我在头插法后没有清除链表所有导致这次错误。把两个代码放出来大家吸取点经验。
void CreateListHead(LinkList *L, int n)
179.{
180. LinkList p;
181. int i;
182. srand(time(0)); /* 初始化随机数种子 */
183. *L = (LinkList)malloc(sizeof(Node));
184. (*L)->next = NULL; /* 先建立一个带头结点的单链表 */
185. for (i=0; i<n; i++)
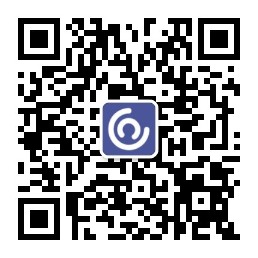
186. {
187. p = (LinkList)malloc(sizeof(Node)); /* 生成新结点 */
188. p->data = rand()%100+1; /* 随机生成100以内的数字 */
189. p->next = (*L)->next;
190. (*L)->next = p; /* 插入到表头 */
191. }
192.}
./* 随机产生n个元素的值,建立带表头结点的单链线性表L(尾插法) */
195.void CreateListTail(LinkList *L, int n)
196.{
197. LinkList p,r;
198. int i;
199. srand(time(0)); /* 初始化随机数种子 */
200. *L = (LinkList)malloc(sizeof(Node)); /* L为整个线性表 */
201. r=*L; /* r为指向尾部的结点 */
202. for (i=0; i<n; i++)
203. {
204. p = (Node *)malloc(sizeof(Node)); /* 生成新结点 */
205. p->data = rand()%100+1; /* 随机生成100以内的数字 */
206. r->next=p; /* 将表尾终端结点的指针指向新结点 */
207. r = p; /* 将当前的新结点定义为表尾终端结点 */
208. }
209. r->next = NULL; /* 表示当前链表结束 */
210.}
///////////////////////////////////////////////////////////////////////////////////////////////////
在插入链表前应该查看链表是否为空
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
#define OK 1
#define ERROR 0
#define TRUE 1
#define FALSE 0
typedef struct Node{
int data;
struct Node *next;
}Node,*PNode;
void init_list(PNode *l)
{
(*l)=(PNode)malloc(sizeof(Node));
if(!(*l))
{
perror("malloc()");
exit(1);
}
(*l)->next=NULL;
}
int list_empty(PNode l)
{
if(l->next)
return FALSE;
else
return TRUE;
}
void insert_Hlist(PNode *l,int num)/*头插法*/
{
PNode q,p=NULL;
int i;
p=*l;
while(p->next) /*判断是否为空*/
p=p->next;
for(i=0;i<num;i++)
{
q=(PNode)malloc(sizeof(Node));
q->data=i+1;
q->next=p->next;
p->next=q;
}
// printf("(*l)->next=%p",(*l)->next);
}
void insert_Tlist(PNode *l,int num) /*尾插法*/
{
PNode p;
PNode ptrav = NULL;
int i;
ptrav = *l;
while(ptrav->next) /*判断是否为空*/
ptrav = ptrav->next;
for(i=0;i<num;i++)
{
p=(PNode)malloc(sizeof(Node));
p->data=9999-i;
ptrav->next=p;
ptrav=p;
}
ptrav->next=NULL;
}
int clear_list(PNode *l)
{
PNode q,p;
p=(*l)->next;
while(p)
{
q=p->next;
free(q);
p=q;
}
(*l)->next=NULL;
return OK;
}
int list_insert(PNode *l,int i,int e)
{
int j;
PNode p,s;
p=*l;
j=1;
while(p&&j<i)
{
p=p->next;
++j;
}
if(!p||j>i)
return ERROR;
s=(PNode)malloc(sizeof(Node));
s->data=e;
s->next=p->next;
p->next=s;
return OK;
}
void show_list(PNode l)
{
PNode p=l->next;
while(p)
{
printf("%d\t",p->data);
p=p->next;
}
printf("\n");
}
void listDelete(PNode *l,int i,int *e)
{
int j;
PNode p,q;
p=*l;
j=1;
while(p->next&&j<i)
{
p=p->next;
++j;
}
if(!(p->next)||j>i)
exit(1);
q=p->next;
// printf("q=%p\n",q);
*e=q->data;
free(q);
}
int length_list(PNode l)
{
int i=0;
PNode p=l->next;
while(p)
{
i++;
p=p->next;
}
return i;
}
int main()
{
PNode p;
init_list(&p);
int i;
/*
for(i=0;i<5;i++)
list_insert(&p,1,i);
insert_Hlist(&p,1000);
show_list(p);
clear_list(&p);
*/
printf("\n\n\n\n\n\n\n");
insert_Hlist(&p,10);
printf("the length of list=%d\n",length_list(p));
// show_list(p);
//list_insert(&p,8,8888);
insert_Tlist(&p,5);
insert_Hlist(&p,5);
printf("the length of list=%d\n",length_list(p));
listDelete(&p,3,&i);
show_list(p);
return 0;
}