版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_27786919/article/details/82905137
最近在做微信公众号支付,看文档做的,感觉踩了不少坑,为了避免后人采坑,所以写下此文档
1:前往微信公众号商户平台,拿到要做支付必要的各种id和密钥。
2:引入微信sdk,在项目中的pom文件中加入下面的依赖(或者直接将下载的sdk demo放入项目)
<dependency>
<groupId>com.github.wxpay</groupId>
<artifactId>wxpay-sdk</artifactId>
<version>0.0.3</version>
</dependency>
3:域名管理,实现主备域名自动切换实现类
public class WXPayDomainSimpleImpl implements IWXPayDomain {
private WXPayDomainSimpleImpl(){}
private static class WxpayDomainHolder{
private static IWXPayDomain holder = new WXPayDomainSimpleImpl();
}
public static IWXPayDomain instance(){
return WxpayDomainHolder.holder;
}
public synchronized void report(final String domain, long elapsedTimeMillis, final Exception ex) {
DomainStatics info = domainData.get(domain);
if(info == null){
info = new DomainStatics(domain);
domainData.put(domain, info);
}
if(ex == null){ //success
if(info.succCount >= 2){ //continue succ, clear error count
info.connectTimeoutCount = info.dnsErrorCount = info.otherErrorCount = 0;
}else{
++info.succCount;
}
}else if(ex instanceof ConnectTimeoutException){
info.succCount = info.dnsErrorCount = 0;
++info.connectTimeoutCount;
}else if(ex instanceof UnknownHostException){
info.succCount = 0;
++info.dnsErrorCount;
}else{
info.succCount = 0;
++info.otherErrorCount;
}
}
public synchronized DomainInfo getDomain(final WXPayConfig config) {
DomainStatics primaryDomain = domainData.get(WXPayConstants.DOMAIN_API);
if(primaryDomain == null ||
primaryDomain.isGood()) {
return new DomainInfo(WXPayConstants.DOMAIN_API, true);
}
long now = System.currentTimeMillis();
if(switchToAlternateDomainTime == 0){ //first switch
switchToAlternateDomainTime = now;
return new DomainInfo(WXPayConstants.DOMAIN_API2, false);
}else if(now - switchToAlternateDomainTime < MIN_SWITCH_PRIMARY_MSEC){
DomainStatics alternateDomain = domainData.get(WXPayConstants.DOMAIN_API2);
if(alternateDomain == null ||
alternateDomain.isGood() ||
alternateDomain.badCount() < primaryDomain.badCount()){
return new DomainInfo(WXPayConstants.DOMAIN_API2, false);
}else{
return new DomainInfo(WXPayConstants.DOMAIN_API, true);
}
}else{ //force switch back
switchToAlternateDomainTime = 0;
primaryDomain.resetCount();
DomainStatics alternateDomain = domainData.get(WXPayConstants.DOMAIN_API2);
if(alternateDomain != null)
alternateDomain.resetCount();
return new DomainInfo(WXPayConstants.DOMAIN_API, true);
}
}
static class DomainStatics {
final String domain;
int succCount = 0;
int connectTimeoutCount = 0;
int dnsErrorCount =0;
int otherErrorCount = 0;
DomainStatics(String domain) {
this.domain = domain;
}
void resetCount(){
succCount = connectTimeoutCount = dnsErrorCount = otherErrorCount = 0;
}
boolean isGood(){ return connectTimeoutCount <= 2 && dnsErrorCount <= 2; }
int badCount(){
return connectTimeoutCount + dnsErrorCount * 5 + otherErrorCount / 4;
}
}
private final int MIN_SWITCH_PRIMARY_MSEC = 3 * 60 * 1000; //3 minutes
private long switchToAlternateDomainTime = 0;
private Map<String, DomainStatics> domainData = new HashMap<String, DomainStatics>();
}
4:配置代码如下
public class JSAPIConfig extends WXPayConfig {
private byte[] certData;
public JSAPIConfig() throws Exception {
//引入证书路径
File file = ResourceUtils.getFile(""填写你的证书路径");
InputStream certStream = new FileInputStream(file);
this.certData = new byte[(int) file.length()];
certStream.read(this.certData);
certStream.close();
}
public String getAppID() {
return "填写你的AppID";
}
public String getMchID() {
return "填写你的getMchID";
}
public String getKey() {
return "填写你的getKey";
}
public InputStream getCertStream() {
ByteArrayInputStream certBis = new ByteArrayInputStream(this.certData);
return certBis;
}
public IWXPayDomain getWXPayDomain() {
return WXPayDomainSimpleImpl.instance();
}
public String getPrimaryDomain() {
return "api.mch.weixin.qq.com";
}
public int getHttpConnectTimeoutMs() {
return 80000;
}
public int getHttpReadTimeoutMs() {
return 100000;
}
}
4:服务器端生成签名后的订单信息返回给前端的controller代码:
@Login
@RequestMapping("UnifiedOrder")
@ApiOperation("公众号用户充值")
public R UnifiedOrder(@ApiIgnore @RequestAttribute("userId") Integer userId, @RequestBody Map<String,Object> params, HttpSession httpSession,HttpServletRequest httpServletRequest) throws Exception {
String trxamt = (String) params.get("trxamt");//充值金额 单位:元
String amount = Tools.yuanToCent(trxamt);//将元转为分
String body = (String) params.get("body");//支付信息 如购买流量-微信充值
String openid = (String) httpSession.getAttribute("openid");//获取用户openid
openid = (openid == null || "".equals(openid)) ? (String) params.get("openid") : openid;
if (openid == null || "".equals(openid)) {
return R.error("opend获取失败");
}
//生成订单号
String orderId = System.currentTimeMillis() + "js" + userId;
Map<String, String> data = new HashMap<String, String>();
data.put("body", body);//支付信息
data.put("out_trade_no", orderId); //订单号
data.put("device_info", "WEB");//设备信息
data.put("fee_type", "CNY");//币种
data.put("total_fee", amount);//充值金额
data.put("spbill_create_ip", "127.0.0.14");//客户端IP
data.put("notify_url","127.0.0.14:8082/api/notify");//异步回调通知地址
data.put("trade_type", "JSAPI"); // 此处指定为扫码支付
data.put("openid", openid);//用户openid
Map res=wxPayService.dounifiedOrder(data,userId);//调取服务层统一下单
if (res.get("code").equals("1")){
logger.error(res.get("msg").toString());
return R.error();
}
return R.ok().put("data",res);
}
5:service中的下单方法
@Override
@Transactional
public Map<String, String> dounifiedOrder(Map<String, String> data, Integer userId){
Map<String, String> fail = new HashMap<>();
fail.put("code","1");
try {
JSAPIConfig config = new JSAPIConfig();
WXPay wxpay = new WXPay(config);
Map<String, String> map = wxpay.unifiedOrder(data);
System.out.println(map);
if (map == null) {
throw new Exception("返回数据错误");
}
if ("SUCCESS".equals(map.get("return_code"))) {
if ("SUCCESS".equals(map.get("result_code"))) {
//此处为下单成功关于订单处理代码
//订单入库
//创建支付信息
Map<String, String> resault = new HashMap<String, String>();
Long timeStamp=WXPayUtil.getCurrentTimestamp();
String prepay_id="prepay_id="+map.get("prepay_id");
String nonceStr =WXPayUtil.generateNonceStr();
resault.put("appId",WXConfig.getAppID());
resault.put("timeStamp",timeStamp.toString());
resault.put("nonceStr",nonceStr);
resault.put("package",prepay_id);
resault.put("signType","MD5");
String sign=WXPayUtil.generateSignature(resault, WXConfig.getKey());
resault.put("paySign",sign);
resault.put("code","0");
return resault;
} else {
throw new Exception("下单失败");
}
} else {
throw new Exception(map.get("return_msg").toString());
}
} catch (Exception e) {
e.printStackTrace();
fail.put("msg",e.getMessage());
return fail;
}
}
6:服务器端支付成功异步回调通知处理方法的controller代码:
扫描二维码关注公众号,回复:
5488421 查看本文章
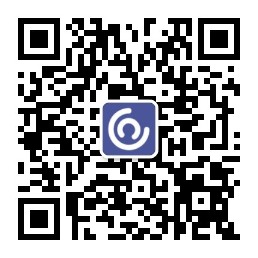
@RequestMapping("JSPayNotify")
@ApiOperation("微信公众号结果通知")
@Transactional
public String JSPayNotify(HttpServletRequest request , HttpServletResponse response) throws UnsupportedEncodingException {
System.out.println("接收到通知");
String notifyData = "";// 支付结果通知的xml格式数据
try {
InputStream is = request.getInputStream();
//将InputStream转换成String
BufferedReader reader = new BufferedReader(new InputStreamReader(is));
StringBuilder sb = new StringBuilder();
String line = null;
try {
while ((line = reader.readLine()) != null) {
sb.append(line + "\n"); }
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
notifyData=sb.toString();
System.err.println(notifyData);
String resault=wxPayService.notify(notifyData);
return resault;
}catch (Exception e){
logger.info("支付回到处理异常");
logger.error(notifyData.toString());
logger.error(e.getMessage());
return "<xml><return_code><![CDATA[FAIL]]></return_code> <return_msg><![CDATA[报文为空]]></return_msg></xml> ";
}
}
7:服务器端支付成功异步回调通知处理方法的service代码:
/**
* 支付结果通知
* @param notifyData 异步通知后的XML数据
* @return
*/
@Override
@Transactional
public String notify(String notifyData){
try{
JSAPIConfig config = new JSAPIConfig();
WXPay wxpay = new WXPay(config);
Map<String, String> notifyMap = WXPayUtil.xmlToMap(notifyData); // 转换成map
if (notifyMap == null) {
throw new Exception("返回数据错误");
}
if ("SUCCESS".equals(notifyMap.get("return_code"))) {
if("SUCCESS".equals(notifyMap.get("result_code"))){
if (wxpay.isPayResultNotifySignatureValid(notifyMap)) {
// 签名正确
// 进行处理。
// 注意特殊情况:订单已经退款,但收到了支付结果成功的通知,不应把商户侧订单状态从退款改成支付成功
return "<xml><return_code><![CDATA[FAIL]]></return_code><return_msg><![CDATA[报文为空]]></return_msg></xml> ";
}
}
} else {
// 签名错误,如果数据里没有sign字段,也认为是签名错误
throw new Exception("签名错误");
}
} else {
throw new Exception("下单失败");
}
} else {
throw new Exception(notifyMap.get("err_code_des"));
}
}catch (Exception e){
return "<xml><return_code><![CDATA[FAIL]]></return_code><return_msg><![CDATA[报文为空]]></return_msg></xml> ";
}
}
注:微信公众号支付签名验证错误
最新微信支付提供的SDK代码中,统一下单接口sign_type是置为 HMAC-SHA256 而不是使用默认的 MD5,而公众号内发起支付的签名只能使用 MD5,正是这种不一致导致了签名验证错误,将统一下单的签名类型改成MD5即可。