Given an array of integers A
sorted in non-decreasing order, return an array of the squares of each number, also in sorted non-decreasing order.
Example 1:
Input: [-4,-1,0,3,10] Output: [0,1,9,16,100]
Example 2:
Input: [-7,-3,2,3,11] Output: [4,9,9,49,121]
Note:
1 <= A.length <= 10000
-10000 <= A[i] <= 10000
A
is sorted in non-decreasing order.
题目理解:
给定一个数组A,已经按照递增顺序排好了,求一个目标数组,其中的元素都是A中元素的平方,也要按照递增顺序排列
解题思路:
这里主要需要考虑的问题是目标数组的排序。如果A中的数字都不为负的话,那么直接按照原来数组的顺序存入每个数字的平方就可以。但是数组中会有负数。
扫描二维码关注公众号,回复:
5497820 查看本文章
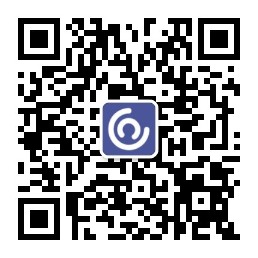
这里可以先找到A中绝对值最小的一个数,从这个数开始,它两边的数的平方一定都是递增的,因此我们采用两个指针,left和right,两个指针都从绝对值最小的数字开始,如果left指向的数的绝对值小,那么就往目标数组里面存入left的平方,然后将left左移,否则存入right的平方,然后将right右移,这样产生的目标数组,就已经按照递增顺序排列好了
class Solution {
public int[] sortedSquares(int[] A) {
int pos = 0, len = A.length;
for(int i = 0; i < len; i++){
if(Math.abs(A[i]) < Math.abs(A[pos]))
pos = i;
if(A[i] > 0)
break;
}
int left = pos, right = pos + 1, it = 0;
int[] res = new int[len];
while(it < len){
if(left == -1){
res[it++] = A[right] * A[right];
right++;
}
else if(right == len){
res[it++] = A[left] * A[left];
left--;
}
else{
if(Math.abs(A[left]) < Math.abs(A[right])){
res[it++] = A[left] * A[left];
left--;
}
else{
res[it++] = A[right] * A[right];
right++;
}
}
}
return res;
}
}