参考官方文档:https://spring.io/guides/gs/accessing-data-mysql
环境:IDEA、Java8、maven、springboot
实践内容:使用MySQL访问数据
pom.xml主要的依赖
在mysql的命令行中创建数据库db_example
create database db_example;
在mysql命令行中创建新的连接数据库用户springuser和密码springpassword
create user 'springuser'@'localhost' identified by 'springpassword';
在mysql命令行中给这个用户添加权限
grant all on db_example.* to 'springuser'@'localhost';
创建application.properties文件
spring.jpa.hibernate.ddl-auto可以是none,update,create,create-drop,请参阅Hibernate文档,了解详细信息。
- none这是默认设置MySQL,不会更改数据库结构。
- update Hibernate根据给定的Entity结构更改数据库。
- create 每次都创建数据库,但不要在关闭时删除它。
- create-drop创建数据库,然后在SessionFactory关闭时删除它。
我们在这里开始是create因为我们还没有数据库结构。第一次运行后,我们可以将其切换到update或none根据程序要求。update当您想对数据库结构进行一些更改时使用。
在数据库处于生产状态之后,您可以none通过连接到Spring应用程序的MySQL用户进行此操作并撤消所有权限,然后只给他SELECT,UPDATE,INSERT,DELETE,这是一种很好的安全做法。
创建实体类User,Hibernate将自动转换为表的实体类。
package hello;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity // This tells Hibernate to make a table out of this class
public class User {
@Id
@GeneratedValue(strategy=GenerationType.AUTO)
private Integer id;
private String name;
private String email;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
创建存储库,UserRepository接口,继承CrudRepository接口。这是存储库接口,这将由Spring在一个bean中自动实现。
package hello;
import org.springframework.data.repository.CrudRepository;
import hello.User;
// This will be AUTO IMPLEMENTED by Spring into a Bean called userRepository
// CRUD refers Create, Read, Update, Delete
public interface UserRepository extends CrudRepository<User, Integer> {
}
创建控制器,UserController
package hello;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import hello.User;
import hello.UserRepository;
@Controller // This means that this class is a Controller
@RequestMapping(path="/demo") // This means URL's start with /demo (after Application path)
public class MainController {
@Autowired // This means to get the bean called userRepository
// Which is auto-generated by Spring, we will use it to handle the data
private UserRepository userRepository;
@GetMapping(path="/add") // Map ONLY GET Requests
public @ResponseBody String addNewUser (@RequestParam String name
, @RequestParam String email) {
// @ResponseBody means the returned String is the response, not a view name
// @RequestParam means it is a parameter from the GET or POST request
User n = new User();
n.setName(name);
n.setEmail(email);
userRepository.save(n);
return "Saved";
}
@GetMapping(path="/all")
public @ResponseBody Iterable<User> getAllUsers() {
// This returns a JSON or XML with the users
return userRepository.findAll();
}
}
上面的示例没有明确指定GETvs. PUT,POST等等,因为它@GetMapping是一个快捷方式@RequestMapping(method=GET)。@RequestMapping默认情况下映射所有HTTP操作。使用@RequestMapping(method=GET)
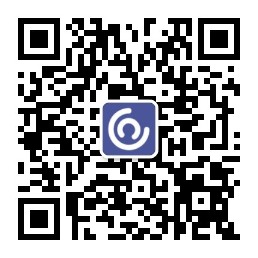
测试运行
浏览器访问:
localhost:8080/demo/add?name=First&[email protected]
添加一条记录
localhost:8080/demo/all
查询所有记录
以上实在开发环境的配置,如果在生产环境可以做一些安全性的配置更改,如下:
- 针对数据库:
revoke all on db_example.* from 'springuser'@'localhost';
移除springuser的所有权限,只给CRUD权限:
grant select, insert, delete, update on db_example.* to 'springuser'@'localhost';
- 针对application.properties配置文件:
spring.jpa.hibernate.ddl-auto=none
可以看到,本项目只有少量的配置。对数据库的操作已经封装的很好,只要在控制器调用封装的CRUD方法即可。
ps:虚心求教。如果内容有误欢迎指出,如果内容帮助了你欢迎留下痕迹。