一、if判断:
1 语法一: 2 if 条件: 3 # 条件成立时执行的子代码块 4 代码1 5 代码2 6 代码3

1 示例: 2 sex='female' 3 age=18 4 is_beautiful=True 5 6 if sex == 'female' and age > 16 and age < 20 and is_beautiful: 7 print('开始表白。。。') 8 9 print('other code1...') 10 print('other code2...') 11 print('other code3...')
1 语法二: 2 if 条件: 3 # 条件成立时执行的子代码块 4 代码1 5 代码2 6 代码3 7 else: 8 # 条件不成立时执行的子代码块 9 代码1 10 代码2 11 代码3

1 sex='female' 2 age=38 3 is_beautiful=True 4 5 if sex == 'female' and age > 16 and age < 20 and is_beautiful: 6 print('开始表白。。。') 7 else: 8 print('阿姨好。。。') 9 10 11 print('other code1...') 12 print('other code2...') 13 print('other code3...')
1 语法三: 2 if 条件1: 3 if 条件2: 4 代码1 5 代码2 6 代码3

1 sex='female' 2 age=18 3 is_beautiful=True 4 is_successful=True 5 height=1.70 6 7 8 if sex == 'female' and age > 16 and age < 20 and is_beautiful \ 9 and height > 1.60 and height < 1.80: 10 print('开始表白。。。') 11 if is_successful: 12 print('在一起。。。') 13 else: 14 print('什么爱情不爱情的,爱nmlgb的爱情,爱nmlg啊.') 15 else: 16 print('阿姨好。。。') 17 18 19 print('other code1...') 20 print('other code2...') 21 print('other code3...')
1 语法四: 2 if 条件1: 3 代码1 4 代码2 5 代码3 6 elif 条件2: 7 代码1 8 代码2 9 代码3 10 elif 条件3: 11 代码1 12 代码2 13 代码3 14 ....... 15 else: 16 代码1 17 代码2 18 代码3

1 示例: 2 如果成绩 >= 90,那么:优秀 3 4 如果成绩 >= 80且 < 90, 那么:良好 5 6 如果成绩 >= 70且 < 80, 那么:普通 7 8 其他情况:很差 9 ''' 10 11 score = input('please input your score: ') # score='100' 12 score = int(score) 13 14 if score >= 90: 15 print('优秀') 16 elif score >= 80: 17 print('良好') 18 elif score >= 70: 19 print('普通') 20 else: 21 print('很差')
二、while循环
1 语法: 2 while 条件: 3 代码1 4 代码2 5 代码3

1 while True: 2 name=input('please input your name: ') 3 pwd=input('please input your password: ') 4 5 if name == 'egon' and pwd == '123': 6 print('login successful') 7 else: 8 print('username or password error')
1 结束while循环的两种方式 2 3 方式一:条件改为False, 4 在条件改为False时不会立即结束掉循环,而是要等到下一次循环判断条件时才会生效 5 6 tag=True 7 while tag: 8 name=input('please input your name: ') 9 pwd=input('please input your password: ') 10 11 if name == 'egon' and pwd == '123': 12 print('login successful') 13 tag=False 14 else: 15 print('username or password error') 16 17 print('===>')
1 方式二:while+break 2 break一定要放在循环体内,一旦循环体执行到break就会立即结束本层循环 3 4 while True: 5 name=input('please input your name: ') 6 pwd=input('please input your password: ') 7 8 if name == 'egon' and pwd == '123': 9 print('login successful') 10 break 11 else: 12 print('username or password error') 13 14 print('===>>>>>') 15 print('===>>>>>')
2.1、while+continue:结束本次循环,直接进入下一次循环
1 # 示例一 2 count=1 3 while count < 6: #count=6 4 if count == 4: 5 count += 1 6 continue 7 8 print(count) 9 count+=1 10 11 # 示例二: 12 while True: 13 name=input('please input your name: ') 14 pwd=input('please input your password: ') 15 16 if name == 'egon' and pwd == '123': 17 print('login successful') 18 break 19 else: 20 print('username or password error') 21 # continue # 此处加continue无用
2.2、了解知识
while + else: while 条件: 代码1 代码2 代码3 else: 在循环结束后,并且在循环没有被break打断过的情况下,才会执行else的代码 tag=True while tag: print(1) print(2) print(3) # tag=False break else: print('else的代码')
2.3、while嵌套
1 #语法 2 while 条件1: 3 while 条件2: 4 代码1 5 代码2 6 代码3 7 8 示例一: 9 while True: 10 name=input('please input your name: ') 11 pwd=input('please input your password: ') 12 13 if name == 'egon' and pwd == '123': 14 print('login successful') 15 while True: 16 print(""" 17 0 退出 18 1 取款 19 2 转账 20 3 查询 21 """) 22 choice=input('请输入您要执行的操作:') #choice='1' 23 if choice == '0': 24 break 25 elif choice == '1': 26 print('取款。。。') 27 elif choice == '2': 28 print('转账。。。') 29 elif choice == '3': 30 print('查询') 31 else: 32 print('输入指令错误,请重新输入') 33 break 34 else: 35 print('username or password error')

1 # 示范二: 2 tag=True 3 while tag: 4 name=input('please input your name: ') 5 pwd=input('please input your password: ') 6 7 if name == 'egon' and pwd == '123': 8 print('login successful') 9 while tag: 10 print(""" 11 0 退出 12 1 取款 13 2 转账 14 3 查询 15 """) 16 choice=input('请输入您要执行的操作:') #choice='1' 17 if choice == '0': 18 tag=False 19 elif choice == '1': 20 print('取款。。。') 21 elif choice == '2': 22 print('转账。。。') 23 elif choice == '3': 24 print('查询') 25 else: 26 print('输入指令错误,请重新输入') 27 else: 28 print('username or password error')
2.4、while练习
1 #1. 使用while循环输出1 2 3 4 5 6 8 9 10 2 #2. 求1-100的所有数的和 3 #3. 输出 1-100 内的所有奇数 4 #4. 输出 1-100 内的所有偶数 5 #5. 求1-2+3-4+5 ... 99的所有数的和 6 #6. 用户登陆(三次机会重试) 7 #7:猜年龄游戏 8 要求: 9 允许用户最多尝试3次,3次都没猜对的话,就直接退出,如果猜对了,打印恭喜信息并退出 10 #8:猜年龄游戏升级版 11 要求: 12 允许用户最多尝试3次 13 每尝试3次后,如果还没猜对,就问用户是否还想继续玩,如果回答Y或y, 就继续让其猜3次,以此往复,如果回答N或n,就退出程序 14 如何猜对了,就直接退出

1 #题一 2 count=1 3 while count <= 10: 4 if count == 7: 5 count+=1 6 continue 7 print(count) 8 count+=1 9 10 #2. 求1-100的所有数的和 11 count=1 12 while count <= 10: 13 if count != 7: 14 print(count) 15 count+=1 16 res=0 17 count=1 18 while count<=100: 19 res+=count 20 count+=1 21 print(res) 22 23 #3. 输出 1-100 内的所有奇数 24 count=1 25 while count<=100: 26 if count%2==1: 27 print(count) 28 count+=1 29 30 #4. 输出 1-100 内的所有偶数 31 count=1 32 while count<=100: 33 if count%2!=1: 34 print(count) 35 count+=1 36 37 #5. 求1-2+3-4+5 ... 99的所有数的和 38 res=0 39 count=1 40 while count<=99: 41 if count%2==1: 42 res-=count 43 else: 44 res+=count 45 count+=1 46 print(res) 47 48 #6. 用户登陆(三次机会重试) 49 count=1 50 while True: 51 username = input('请输入用户名:') 52 userpwd = input('请输入用户密码:') 53 if count!=3: 54 if username=='zhaokang' and userpwd=='123': 55 print('登陆成功!') 56 break 57 else: 58 break 59 count+=1 60 61 62 #7:猜年龄游戏 63 #要求: 64 # 允许用户最多尝试3次,3次都没猜对的话,就直接退出,如果猜对了,打印恭喜信息并退出 65 count=1 66 while True: 67 age=int(input('请输入年龄:')) 68 if count!=3: 69 if age==18: 70 print('猜对了') 71 break 72 else: 73 print('抱歉') 74 else: 75 break 76 count+=1 77 78 79 #8:猜年龄游戏升级版 80 #要求: 81 # 允许用户最多尝试3次 82 # 每尝试3次后,如果还没猜对,就问用户是否还想继续玩,如果回答Y或y, 就继续让其猜3次,以此往复,如果回答N或n,就退出程序 83 # 如何猜对了,就直接退出 84 85 age_of_oldboy=73 86 87 count=0 88 while True: 89 if count == 3: 90 choice=input('继续(Y/N?)>>: ') 91 if choice == 'Y' or choice == 'y': 92 count=0 93 else: 94 break 95 96 guess=int(input('>>: ')) 97 if guess == age_of_oldboy: 98 print('you got it') 99 break 100 count+=1
三、for循环
1 迭代式循环:for,语法如下 for i in range(10): 缩进的代码块 2 break与continue(同上)
3 循环嵌套

1 for i in range(1,10): 2 for j in range(1,i+1): 3 print('%s*%s=%s' %(i,j,i*j),end=' ') 4 print()
扫描二维码关注公众号,回复:
5608842 查看本文章
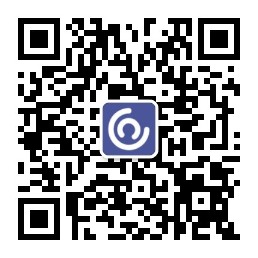