转
https://blog.csdn.net/GoForwardToStep/article/details/77887777一、简述
之前写了一篇 Qt 之 自定义窗口标题栏 ,用重写了窗口的标题栏,今天为此篇的续篇,对自定义窗口再进行拓展,因为进行了自定义窗口标题栏,去掉了窗口原有的边框,所以鼠标放置窗口边框对窗口进行拉伸的功能也就没有了,所以需要自己实现。
其实窗口拉伸功能在 Qt 之 简单截图功能(三)实现可拖拽选中区域 文章中已经有类似的实现,但也有一些差别,下面就窗口拉伸功能进行讲述。
注意:
在阅读此篇文章前可以先阅读 Qt 之 自定义窗口标题栏 文章,需要了解这篇文章中的一些代码,下面讲述的将不包括以上代码,只讲述实现窗口拉伸的代码。
二、代码之路
画了一个简单的示意图,其实窗口拉伸也就这8块区域,当鼠标移动进入这8块区域时,需要判断当前进入了哪一块区域,然后显示什么样式。我们在Qt助手中找到如下四个样式,同时后面也有相应的解释说明。下面就一步一步分析如何实现窗口拉伸功能。
1、计算窗口上这8个区域Rect
什么时候需要计算呢?
(1)在窗口第一次show完之后需要计算(在showEvent事件中调用)。
(2)在每次窗口拉伸完时,也就是鼠标左键松开时计算一次。因为窗口的大小发生了变化(虽然在窗口拉伸的过程中,窗口大小也会变化,但是不需要再计算)。
#define STRETCH_RECT_HEIGHT 4 // 拉伸小矩形的高度;
#define STRETCH_RECT_WIDTH 4 // 拉伸小矩形的宽度;
// 计算拉伸区域Rect位置;
// 以下8个Rect对应上图中8个区域;
void BaseWindow::calculateCurrentStrechRect()
{
// 四个角Rect;
m_leftTopRect = QRect(0, 0, STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
m_leftBottomRect = QRect(0, this->height() - STRETCH_RECT_HEIGHT, STRETCH_RECT_WIDTH, STRETCH_RECT_WIDTH);
m_rightTopRect = QRect(this->width() - STRETCH_RECT_WIDTH, 0, STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
m_rightBottomRect = QRect(this->width() - STRETCH_RECT_WIDTH, this->height() - STRETCH_RECT_HEIGHT, STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT);
// 四条边Rect;
m_topBorderRect = QRect(STRETCH_RECT_WIDTH, 0, this->width() - STRETCH_RECT_WIDTH * 2, STRETCH_RECT_HEIGHT);
m_rightBorderRect = QRect(this->width() - STRETCH_RECT_WIDTH, STRETCH_RECT_HEIGHT, STRETCH_RECT_WIDTH, this->height() - STRETCH_RECT_HEIGHT * 2);
m_bottomBorderRect = QRect(STRETCH_RECT_WIDTH, this->height() - STRETCH_RECT_HEIGHT, this->width() - STRETCH_RECT_WIDTH * 2, STRETCH_RECT_HEIGHT);
m_leftBorderRect = QRect(0, STRETCH_RECT_HEIGHT, STRETCH_RECT_WIDTH, this->height() - STRETCH_RECT_HEIGHT * 2);
}
// 在窗口第一次show完之后需要计算拉伸区域Rect位置;
void BaseWindow::showEvent(QShowEvent *event)
{
calculateCurrentStrechRect();
return __super::showEvent(event);
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
2、根据当前鼠标的位置显示不同的样式
// 定义当前鼠标所处状态;
enum WindowStretchRectState
{
NO_SELECT = 0, // 鼠标未进入下方矩形区域;
LEFT_TOP_RECT, // 鼠标在左上角区域;
TOP_BORDER, // 鼠标在上边框区域;
RIGHT_TOP_RECT, // 鼠标在右上角区域;
RIGHT_BORDER, // 鼠标在右边框区域;
RIGHT_BOTTOM_RECT, // 鼠标在右下角区域;
BOTTOM_BORDER, // 鼠标在下边框区域;
LEFT_BOTTOM_RECT, // 鼠标在左下角区域;
LEFT_BORDER // 鼠标在左边框区域;
};
// 根据当前鼠标位置,定位鼠标在具体哪一块拉伸区域;
WindowStretchRectState BaseWindow::getCurrentStretchState(QPoint cursorPos)
{
WindowStretchRectState stretchState;
if (m_leftTopRect.contains(cursorPos))
{
stretchState = LEFT_TOP_RECT;
}
else if (m_rightTopRect.contains(cursorPos))
{
stretchState = RIGHT_TOP_RECT;
}
else if (m_rightBottomRect.contains(cursorPos))
{
stretchState = RIGHT_BOTTOM_RECT;
}
else if (m_leftBottomRect.contains(cursorPos))
{
stretchState = LEFT_BOTTOM_RECT;
}
else if (m_topBorderRect.contains(cursorPos))
{
stretchState = TOP_BORDER;
}
else if (m_rightBorderRect.contains(cursorPos))
{
stretchState = RIGHT_BORDER;
}
else if (m_bottomBorderRect.contains(cursorPos))
{
stretchState = BOTTOM_BORDER;
}
else if (m_leftBorderRect.contains(cursorPos))
{
stretchState = LEFT_BORDER;
}
else
{
stretchState = NO_SELECT;
}
return stretchState;
}
// 根据getCurrentStretchState返回状态进行更新鼠标样式;
void BaseWindow::updateMouseStyle(WindowStretchRectState stretchState)
{
switch (stretchState)
{
case NO_SELECT:
setCursor(Qt::ArrowCursor);
break;
case LEFT_TOP_RECT:
case RIGHT_BOTTOM_RECT:
setCursor(Qt::SizeFDiagCursor);
break;
case TOP_BORDER:
case BOTTOM_BORDER:
setCursor(Qt::SizeVerCursor);
break;
case RIGHT_TOP_RECT:
case LEFT_BOTTOM_RECT:
setCursor(Qt::SizeBDiagCursor);
break;
case LEFT_BORDER:
case RIGHT_BORDER:
setCursor(Qt::SizeHorCursor);
break;
default:
setCursor(Qt::ArrowCursor);
break;
}
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
3、通过重写鼠标事件完成窗口拉伸的操作
主要是重写mouseMoveEvent、mousePressEvent、mouseReleaseEvent这三个事件。
这三个事件具体的操作请看代码中的注释。
扫描二维码关注公众号,回复:
57059 查看本文章
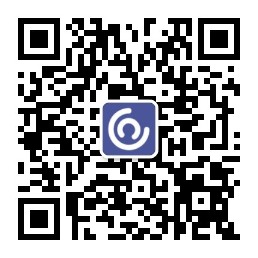
// 重写mouseMoveEvent事件,用于获取当前鼠标的位置,将位置传递给getCurrentStretchState方法,得到当前鼠标的状态,然后调用updateMouseStyle对鼠标的样式进行更新;
void BaseWindow::mouseMoveEvent(QMouseEvent *event)
{
// 如果窗口最大化是不能拉伸的;
// 也不用更新鼠标样式;
if (m_isWindowMax)
{
return __super::mouseMoveEvent(event);
}
// 如果当前鼠标未按下,则根据当前鼠标的位置更新鼠标的状态及样式;
if (!m_isMousePressed)
{
QPoint cursorPos = event->pos();
// 根据当前鼠标的位置显示不同的样式;
m_stretchRectState = getCurrentStretchState(cursorPos);
updateMouseStyle(m_stretchRectState);
}
// 如果当前鼠标左键已经按下,则记录下第二个点的位置,并更新窗口的大小;
else
{
m_endPoint = this->mapToGlobal(event->pos());
updateWindowSize();
}
return __super::mouseMoveEvent(event);
}
void BaseWindow::mousePressEvent(QMouseEvent *event)
{
// 当前鼠标进入了以上指定的8个区域,并且是左键按下时才开始进行窗口拉伸;
if (m_stretchRectState != NO_SELECT && event->button() == Qt::LeftButton)
{
m_isMousePressed = true;
// 记录下当前鼠标位置,为后面计算拉伸位置;
m_startPoint = this->mapToGlobal(event->pos());
// 保存下拉伸前的窗口位置及大小;
m_windowRectBeforeStretch = this->geometry();
}
return __super::mousePressEvent(event);
}
void BaseWindow::mouseReleaseEvent(QMouseEvent *event)
{
// 鼠标松开后意味之窗口拉伸结束,置标志位,并且重新计算用于拉伸的8个区域Rect;
m_isMousePressed = false;
calculateCurrentStrechRect();
return __super::mouseReleaseEvent(event);
}
// 拉伸窗口过程中,根据记录的坐标更新窗口大小;
void BaseWindow::updateWindowSize()
{
// 拉伸时要注意设置窗口最小值;
QRect windowRect = m_windowRectBeforeStretch;
int delValue_X = m_startPoint.x() - m_endPoint.x();
int delValue_Y = m_startPoint.y() - m_endPoint.y();
if (m_stretchRectState == LEFT_BORDER)
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setX(topLeftPoint.x() - delValue_X);
windowRect.setTopLeft(topLeftPoint);
}
else if (m_stretchRectState == RIGHT_BORDER)
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setX(bottomRightPoint.x() - delValue_X);
windowRect.setBottomRight(bottomRightPoint);
}
else if (m_stretchRectState == TOP_BORDER)
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setY(topLeftPoint.y() - delValue_Y);
windowRect.setTopLeft(topLeftPoint);
}
else if (m_stretchRectState == BOTTOM_BORDER)
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setY(bottomRightPoint.y() - delValue_Y);
windowRect.setBottomRight(bottomRightPoint);
}
else if (m_stretchRectState == LEFT_TOP_RECT)
{
QPoint topLeftPoint = windowRect.topLeft();
topLeftPoint.setX(topLeftPoint.x() - delValue_X);
topLeftPoint.setY(topLeftPoint.y() - delValue_Y);
windowRect.setTopLeft(topLeftPoint);
}
else if (m_stretchRectState == RIGHT_TOP_RECT)
{
QPoint topRightPoint = windowRect.topRight();
topRightPoint.setX(topRightPoint.x() - delValue_X);
topRightPoint.setY(topRightPoint.y() - delValue_Y);
windowRect.setTopRight(topRightPoint);
}
else if (m_stretchRectState == RIGHT_BOTTOM_RECT)
{
QPoint bottomRightPoint = windowRect.bottomRight();
bottomRightPoint.setX(bottomRightPoint.x() - delValue_X);
bottomRightPoint.setY(bottomRightPoint.y() - delValue_Y);
windowRect.setBottomRight(bottomRightPoint);
}
else if (m_stretchRectState == LEFT_BOTTOM_RECT)
{
QPoint bottomLeftPoint = windowRect.bottomLeft();
bottomLeftPoint.setX(bottomLeftPoint.x() - delValue_X);
bottomLeftPoint.setY(bottomLeftPoint.y() - delValue_Y);
windowRect.setBottomLeft(bottomLeftPoint);
}
// 避免宽或高为零窗口显示有误,这里给窗口设置最小拉伸高度、宽度;
if (windowRect.width() < m_windowMinWidth)
{
windowRect.setLeft(this->geometry().left());
windowRect.setWidth(m_windowMinWidth);
}
if (windowRect.height() < m_windowMinHeight)
{
windowRect.setTop(this->geometry().top());
windowRect.setHeight(m_windowMinHeight);
}
this->setGeometry(windowRect);
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
4、提供设置窗口是否支持拉伸功能的接口;
// 设置当前窗口是否支持拉伸;
// 此方法需要在调用完initTitleBar方法之后调用,因为m_titleBar在initTitleBar方法中创建;
void BaseWindow::setSupportStretch(bool isSupportStretch)
{
// 因为需要在鼠标未按下的情况下通过mouseMoveEvent事件捕捉鼠标位置,所以需要设置setMouseTracking为true(如果窗口支持拉伸);
m_isSupportStretch = isSupportStretch;
this->setMouseTracking(isSupportStretch);
// 这里对子控件也进行了设置,是因为如果不对子控件设置,当鼠标移动到子控件上时,不会发送mouseMoveEvent事件,也就获取不到当前鼠标位置,无法判断鼠标状态及显示样式了。
QList<QWidget*> widgetList = this->findChildren<QWidget*>();
for each (QWidget* widget in widgetList)
{
widget->setMouseTracking(isSupportStretch);
}
// 这里加了非空判断,防止m_titleBar未创建;
if (m_titleBar != NULL)
{
// m_titleBar同理,也需要对自己及子控件进行调用setMouseTracking进行设置,见上方注释;
m_titleBar->setSupportStretch(isSupportStretch);
}
}
// 返回当前窗口是否支持拉伸;
bool BaseWindow::getSupportStretch()
{
return m_isSupportStretch;
}
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
效果图:
尾
整体代码如上所示,这里只展示了窗口拉伸功能的代码,整个工程代码后续会上传CSDN上,有兴趣的小伙伴可以下载学习,参考。不过以上代码并不完美,实现了大致的功能,但是仍有小问题存在,后面会不断改进。