package com.cxy; import java.util.HashMap; import java.util.Map; import java.util.concurrent.CountDownLatch; import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.Semaphore; /** * Created by Administrator on 2019/4/10. */ public class CxyDemo { // 请求总数 public static int clientTotal = 5000; // 同时并发执行的线程数 public static int threadTotal = 200; private static Map<Integer, Integer> map = new HashMap<>(); public static void main(String[] args) throws Exception { ExecutorService executorService = Executors.newCachedThreadPool(); final Semaphore semaphore = new Semaphore(threadTotal); final CountDownLatch countDownLatch = new CountDownLatch(clientTotal); for (int i = 0; i < clientTotal; i++) { final int count = i; executorService.execute(() -> { try { semaphore.acquire(); update(count); semaphore.release(); } catch (Exception e) { // log.error("exception" , e); } countDownLatch.countDown(); }); } countDownLatch.await(); executorService.shutdown(); System.out.println(map.size()); //log.info("size:{}" , map.size()); } private static void update(int i) { map.put(i, i); } }
hashmap不是一个线程安全的类,上面就是对其进行测试
执行结果:
再执行一次:
可见不是线程安全的,
package com.cxy; import java.util.HashMap; import java.util.Map; import java.util.concurrent.*; /** * Created by Administrator on 2019/4/10. */ public class CxyDemo { // 请求总数 public static int clientTotal = 5000; // 同时并发执行的线程数 public static int threadTotal = 200; //private static Map<Integer, Integer> map = new HashMap<>(); private static ConcurrentHashMap<Integer, Integer> map = new ConcurrentHashMap<>(); public static void main(String[] args) throws Exception { ExecutorService executorService = Executors.newCachedThreadPool(); final Semaphore semaphore = new Semaphore(threadTotal); final CountDownLatch countDownLatch = new CountDownLatch(clientTotal); for (int i = 0; i < clientTotal; i++) { final int count = i; executorService.execute(() -> { try { semaphore.acquire(); update(count); semaphore.release(); } catch (Exception e) { // log.error("exception" , e); } countDownLatch.countDown(); }); } countDownLatch.await(); executorService.shutdown(); System.out.println(map.size()); //log.info("size:{}" , map.size()); } private static void update(int i) { map.put(i, i); } }
测试concurrentHashmap,可以知道是线程安全的
package com.cxy; import java.util.HashMap; import java.util.Hashtable; import java.util.Map; import java.util.concurrent.*; /** * Created by Administrator on 2019/4/10. */ public class CxyDemo { // 请求总数 public static int clientTotal = 5000; // 同时并发执行的线程数 public static int threadTotal = 200; //private static Map<Integer, Integer> map = new HashMap<>(); // private static ConcurrentHashMap<Integer, Integer> map = new ConcurrentHashMap<>(); private static Map<Integer, Integer> map = new Hashtable<>(); public static void main(String[] args) throws Exception { ExecutorService executorService = Executors.newCachedThreadPool(); final Semaphore semaphore = new Semaphore(threadTotal); final CountDownLatch countDownLatch = new CountDownLatch(clientTotal); for (int i = 0; i < clientTotal; i++) { final int count = i; executorService.execute(() -> { try { semaphore.acquire(); update(count); semaphore.release(); } catch (Exception e) { // log.error("exception" , e); } countDownLatch.countDown(); }); } countDownLatch.await(); executorService.shutdown(); System.out.println(map.size()); //log.info("size:{}" , map.size()); } private static void update(int i) { map.put(i, i); } }
hashtable也是线程安全:
总结:通过semaphore来模拟线程数,然后通过countdownlatch线程计数器来计算执行的线程,这样可以来模拟高并发测试
扫描二维码关注公众号,回复:
5834654 查看本文章
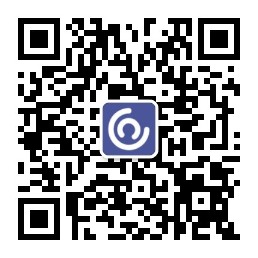