- 组合模式允许你将对象组合成树形结构来表现”部分-整体“的层次结构,使得客户以一致的方式处理单个对象以及对象的组合。
- 组合模式实现的最关键的地方是——简单对象和复合对象必须实现相同的接口。这就是组合模式能够将组合对象和简单对象进行一致处理的原因。
- 组合部件(Component):它是一个抽象角色,为要组合的对象提供统一的接口。
- 叶子(Leaf):在组合中表示子节点对象,叶子节点不能有子节点。
- 合成部件(Composite):定义有枝节点的行为,用来存储部件,实现在Component接口中的有关操作,如增加(Add)和删除(Remove)。
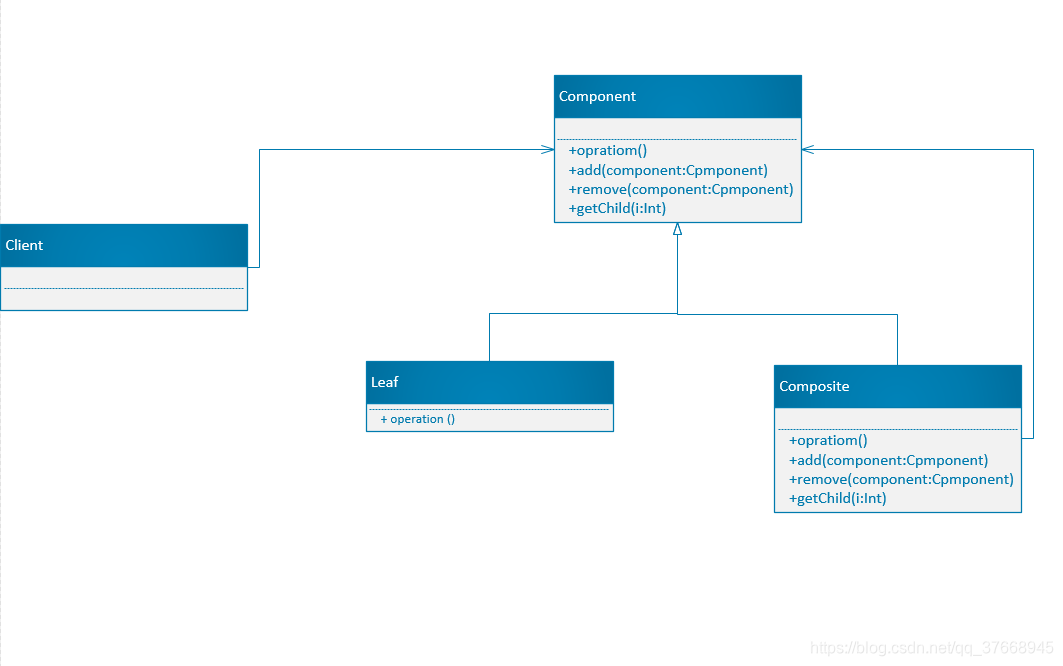
例子:学校作为根节点,学院做普通节点,专业就是叶子。
package com.component;
/**
* 组件类
* 机构组件,学院和系称为机构
* @Author zhaoxin
* @Email [email protected]
* @Description //TODO
* @Date 2019/4/8
**/
public abstract class OrganizationComponent {
public void add(OrganizationComponent organizationComponent){
throw new UnsupportedOperationException();
}
public void remove(OrganizationComponent organizationComponent){
throw new UnsupportedOperationException();
}
public String getName(){
throw new UnsupportedOperationException();
}
public String getDescription(){
throw new UnsupportedOperationException();
}
public void print(){
throw new UnsupportedOperationException();
}
}
package com.component;
import java.util.ArrayList;
import java.util.List;
/**
* @Author zhaoxin
* @Email [email protected]
* @Description 大学对象
* @Date 2019/4/8
**/
public class University extends OrganizationComponent {
String name;
String description;
List<OrganizationComponent> organizationComponents=new ArrayList<OrganizationComponent>();
public University (String name,String description){
this.name=name;
this.description=description;
}
@Override
public void add(OrganizationComponent organizationComponent) {
organizationComponents.add(organizationComponent);
}
@Override
public void remove(OrganizationComponent organizationComponent) {
organizationComponents.remove(organizationComponent);
}
@Override
public String getName() {
return name;
}
@Override
public String getDescription() {
return description;
}
@Override
public void print() {
System.out.println("-------" + getName() + "-----------");
// 大学下面有很多学院,把他们遍历出来
for(OrganizationComponent organizationComponent : organizationComponents){
organizationComponent.print();
}
}
}
package com.component;
import java.util.ArrayList;
import java.util.List;
/**
* @Author zhaoxin
* @Email [email protected]
* @Description 学院是一个机构
* @Date 2019/4/8
*
**/
public class College extends OrganizationComponent {
String name;
String description;
List<OrganizationComponent> organizationComponents = new ArrayList<OrganizationComponent>();
public College(String name, String description) {
this.name = name;
this.description = description;
}
// 重写机构组件的方法,其作为树有增加和删除方法
@Override
public void add(OrganizationComponent organizationComponent) {
organizationComponents.add(organizationComponent);
}
@Override
public void remove(OrganizationComponent organizationComponent) {
organizationComponents.remove(organizationComponent);
}
@Override
public String getName() {
return name;
}
@Override
public String getDescription() {
return description;
}
@Override
public void print() {
System.out.println("-------" + getName() + "-----------");
// 学院下面有很多专业,把他们遍历出来
for(OrganizationComponent organizationComponent : organizationComponents){
organizationComponent.print();
}
}
}
package com.component;
/**
* @Author zhaoxin
* @Email [email protected]
* @Description //TODO
* @Date 2019/4/8
**/
public class Department extends OrganizationComponent {
String name;
String description;
public Department(String name, String description) {
this.name = name;
this.description = description;
}
// 重写机构组件的方法,其作为叶子没有增加和删除方法
@Override
public String getName() {
return name;
}
@Override
public String getDescription() {
return description;
}
// 叶子只需要输出自己的信息
@Override
public void print() {
System.out.println(getName());
}
}
package com.component;
/**
* @Author zhaoxin
* @Email [email protected]
* @Description //输出信息,模拟客户调用
* @Date 2019/4/8
**/
public class OutputInfo {
OrganizationComponent allOrganization;
public OutputInfo(OrganizationComponent allOrganization){
this.allOrganization=allOrganization;
}
public void printOrganization(){
allOrganization.print();
}
}
package com.component;
import com.component.*;
/**
* @Author zhaoxin
* @Email [email protected]
* @Description //测试组合模式
* @Date 2019/4/8
**/
public class CompositeTest {
public static void main(String[] args) {
// 从大到小创建对象,学院和专业组合成为学校,先创建学校,它也是机构组件
OrganizationComponent university=new University("河南工程学院","全国最好的学校");
//创建学院
OrganizationComponent college1=new College("计算机学院","河南工程学院最好的院系");
OrganizationComponent college2=new College("人文社会科学学院","不知道干啥类");
//计算机学院专业
college1.add(new Department("软件工程","软工"));
college1.add(new Department("计算机科学与技术","机科"));
college1.add(new Department("大数据科学与分析","大数据"));
//人文社会科学学院专业
college2.add(new Department("1111","1111"));
college2.add(new Department("2222","2222"));
college2.add(new Department("3333","3333"));
// 学校有下面学院
university.add(college1);
university.add(college2);
// 输出学校机构信息
OutputInfo info = new OutputInfo(university);
info.printOrganization();
}
}