版权声明:本文为博主原创文章,未经博主允许不得转载。 https://blog.csdn.net/qq_36169781/article/details/88529685
1. Excel中xls文件转DataTable数据表
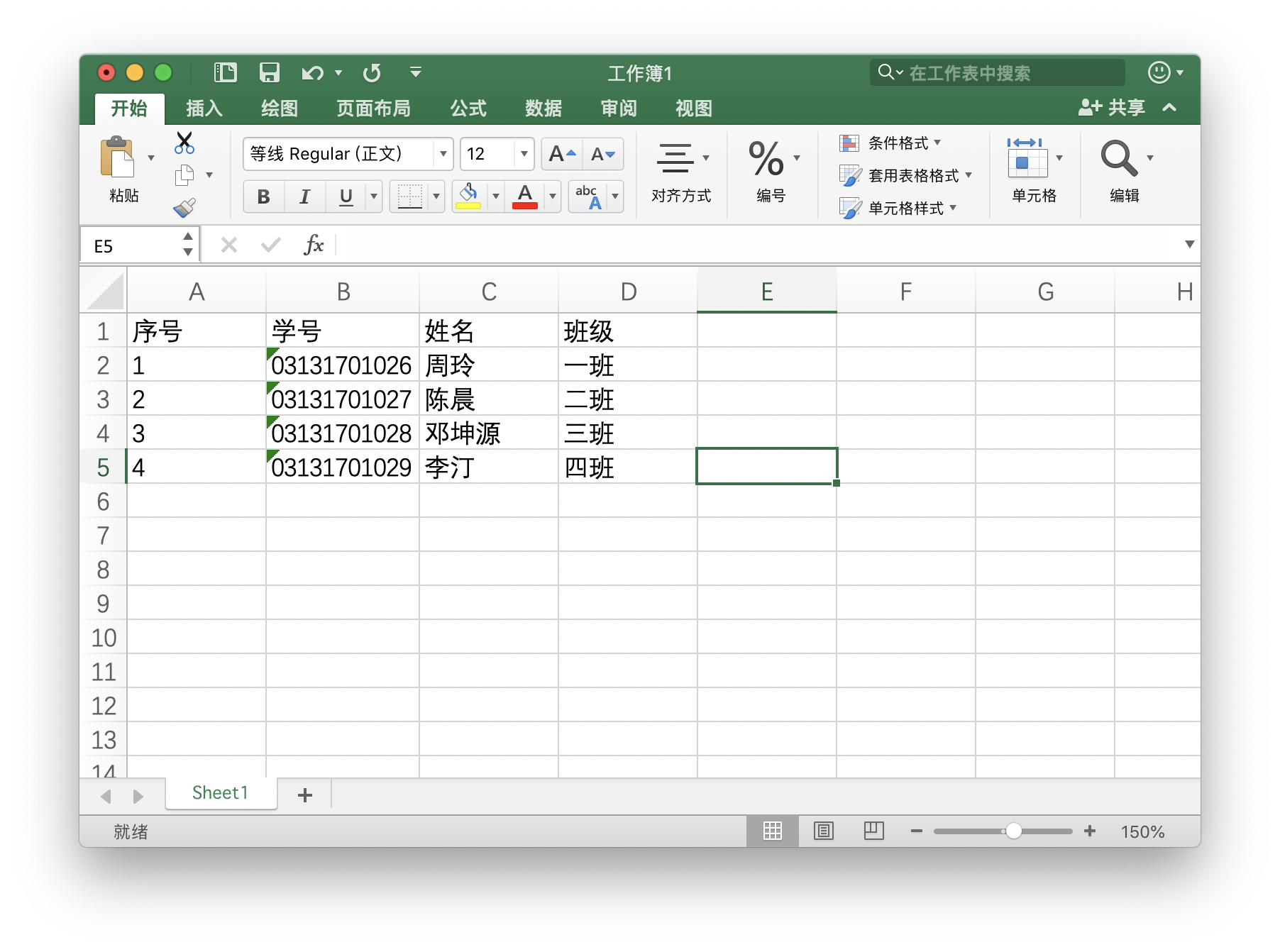
/// <summary>
/// 初始化数据绑定
/// <param name="filePath">Excel文件路径,应包含完整的文件名</param>
/// </summary>
public DataTable ExcleToDt(string filePath)
{
//配置连接字符串
OleDbConnection oleCon = new OleDbConnection("Provider=Microsoft.Jet.Oledb.4.0;Data Source='" + filePath + "';Extended Properties='Excel 8.0;HDR=Yes;IMEX=1;'");
//打开连接
oleCon.Open();
string Sql = "SELECT * FROM [Sheet1$] ";
try{
OleDbDataAdapter mycommand = new OleDbDataAdapter(Sql, oleCon);
}
catch(Exception ee){
//不支持的文件类型,在Oledb4.0中仅支持.xls,更高的Excel版本需要高版本Oledb支持
}
DataSet ds = new DataSet();//使用表格的Sheet1数据填充数据集
mycommand.Fill(ds, "[Sheet1$]");
oleCon.Close();//完成过程后记得关闭连接
return ds.Tables["[Sheet1$]"];
}
2.DataTable绑定GridView
使用Excel文件转换为DataTable数据表后作为GridView的数据源进行绑定
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
string filePath = "test/test.xls";//文件路径
DataTable dt = ExcleToDt(filePath);//转DataTable
//将转换的DataTable列名改为英文,方便绑定
dt.Columns.ColumnName[0]="s_studyno";
dt.Columns.ColumnName[1]="s_name";
dt.Columns.ColumnName[2]="s_class";
GridView1.DataSource = dt;//作为GridView数据源
GridView1.DataBind();
}
前台GridView绑定主要代码:
<asp:GridView ID="GridView1" runat="server" CssClass="gridview" Width="100%" OnRowDataBound="GridView1_RowDataBound" >
<Columns>
<asp:BoundField DataField="s_studyno" HeaderText="学生编号"></asp:BoundField>
<asp:BoundField DataField="s_name" HeaderText="姓名"></asp:BoundField>
<asp:BoundField DataField="s_class" HeaderText="行政班"></asp:BoundField>
</Columns>
</asp:GridView>
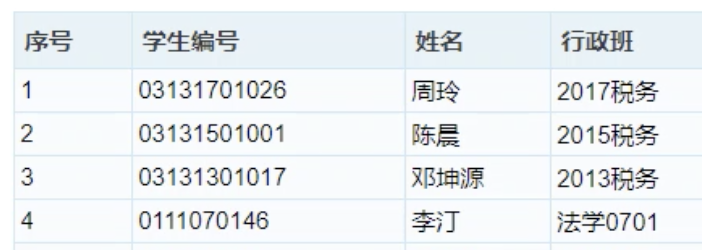
3.Excel上传+转DataTable+GridView实例
前端代码:
<form id="form1" runat="server">
<table cellspacing="0" cellpadding="0" width="95%" border="0" style="margin:10px">
<tr>
<td colspan="2" height="25" width="*">
<asp:MultiView ID="mvTable" runat="server">
<asp:View ID="View1" runat="server">
<table width="100%" border="0" cellspacing="1" cellpadding="1" bgcolor="B7CEE3">
<tr bgcolor="#F0F5F9">
<td height="20">
<table width="100%" border="0" cellspacing="0" cellpadding="0" height="35">
<tr bgcolor="#F0F5F9">
<td>
<asp:Label ID="label1" runat="server" Text="上传EXCEL:" style="float:left;margin-top:5px;margin-left:10px"></asp:Label>
<asp:FileUpload ID="fUExcel" runat="server" style="float:left"/>
<asp:Button ID="toUpload" runat="server" CssClass="layui-btn layui-btn-small layui-btn-danger" style="float:left;margin-left:10px" Text="上传" OnClick="toUpload_Click"></asp:Button>
</td>
</tr>
</table>
</td>
</tr>
</table>
<br />
<asp:GridView ID="GridView1" runat="server" CssClass="gridview" Width="100%" DataKeyNames="tblStudentID" AutoGenerateColumns="False"
OnRowDataBound="GridView1_RowDataBound" EnableModelValidation="True" OnRowCommand="GridView1_RowCommand" PageSize="20">
<Columns>
<asp:BoundField DataField="s_studyno" HeaderText="学生编号"></asp:BoundField>
<asp:BoundField DataField="s_name" HeaderText="姓名"></asp:BoundField>
<asp:BoundField DataField="s_class" HeaderText="行政班"></asp:BoundField>
</Columns>
</asp:GridView>
<webdiyer:AspNetPager ID="AspNetPager1" runat="server" CustomInfoText="" FirstPageText="首页"
InputBoxClass="input" LastPageText="尾页" NextPageText="下一页" OnPageChanged="AspNetPager1_PageChanged"
PrevPageText="上一页" ShowCustomInfoSection="Left" SubmitButtonClass="btn" ShowFirstLast="true"
ShowPrevNext="true" NumericButtonCount="5" ShowDisabledButtons="true" ShowPageIndexBox="Always"
AlwaysShow="True" CustomInfoSectionWidth="30%" Width="99%" Visible="true" SubmitButtonText="跳转"
CustomInfoHTML="总记录:%RecordCount%条 当前 %CurrentPageIndex% / %PageCount% 页" PageSize="15">
</webdiyer:AspNetPager>
</asp:View>
</asp:MultiView>
</td>
</tr>
</table>
</form>
后端代码:
protected void toUpload_Click(object sender, EventArgs e)
{
HttpPostedFile p = fUExcel.PostedFile;//获取fileUpload控件载入的文件
string filename = "";
if (p.FileName.Trim() != "")
{
//生成新的文件名
filename = DateTime.Now.ToString("yyyyMMddHHmm") + ran.Next(9999) + Path.GetFileName(p.FileName);
}
else
{
Response.Write("<script>window.alert('请先选择导入文件后,再执行导入!');</script>");
return;
}
//如果目标上传文件夹路径不存在则创建目录
if (!Directory.Exists(Server.MapPath(@"~/UpLoadFile/ImportStudentsCache" + "//")))//文件临时存放目录
Directory.CreateDirectory(Server.MapPath(@"~/UpLoadFile/ImportStudentsCache" + "//"));
//文件路径
string filePath = Server.MapPath(@"~/UpLoadFile/ImportStudentsCache" + "//" + filename);
//fileUpload控件保存文件
fUExcel.SaveAs(filePath);
if (filename.Contains("xls"))//Oledb.4.0仅支持.xls,更高的Excel版本需要高版本Oledb支持
{
//配置连接字符串
OleDbConnection oleCon = new OleDbConnection("Provider=Microsoft.Jet.Oledb.4.0;Data Source='" + filePath + "';Extended Properties='Excel 8.0;HDR=Yes;IMEX=1;'");
oleCon.Open();
string Sql = "SELECT * FROM [Sheet1$] ";
OleDbDataAdapter mycommand = new OleDbDataAdapter(Sql, oleCon);
//使用表格的Sheet1数据填充数据集
DataSet ds = new DataSet();
mycommand.Fill(ds, "[Sheet1$]");
oleCon.Close();//完成过程后记得关闭连接
int count = ds.Tables["[Sheet1$]"].Rows.Count;//返回Sheet1的行数
//如果项目中用到了导入模板文件,可以取消以下注视判断模板的有效性
// try
// {
// ds.Tables["[Sheet1$]"].Rows[0]["学号"].ToString().Trim();
// }
// catch (Exception eee)//不包含学号列(模板中学号列名被修改)
// {
// File.Delete(filePath);
// Response.Write("<script>window.alert('导入失败!请勿更改模板列名!');</script>");
// return;
// }
//读取完后删除导入的文件
try
{
File.Delete(filePath);
}
catch (Exception eee)
{
Response.Write("<script>window.alert('" + eee.Message.ToString() + "');</script>");
}
}
else
{
Response.Write("<script>window.alert('请检查您选择的文件是否为Excel文件!');</script>");
return;
}
PageInit();//页面功能主函数,包含数据库交互和数据绑定。
}