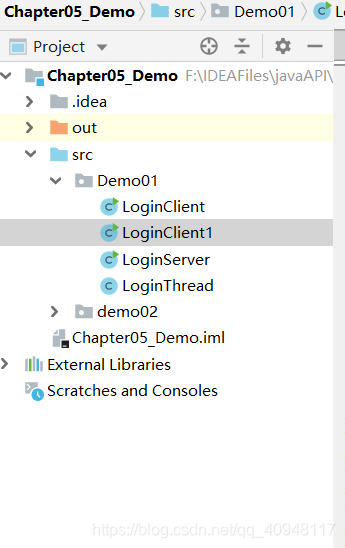
这是一个简单利用线程实现客户端和服务端的数据交互
package Demo01;
import jdk.internal.util.xml.impl.Input;
import java.io.*;
import java.net.Socket;
import java.util.Scanner;
/**
* 客户端
*/
public class LoginClient {
public static void main(String[] args) {
while (true) {
Socket socket = null;
OutputStream os = null;
InputStream is = null;
BufferedReader br = null;
try {
//建立连接,指定服务器及端口
socket = new Socket("localhost", 8800);
//信息
Scanner input = new Scanner(System.in);
System.out.println("请输入:");
String str = input.next();
//得到输出流
os = socket.getOutputStream();
//写
os.write(str.getBytes());
socket.shutdownOutput(); //让流半关闭
//接收服务端响应
//得到输入流
is = socket.getInputStream();
//输出信息
br = new BufferedReader(new InputStreamReader(is));
String reply = br.readLine();
while (reply != null) {
System.out.println("我是客户端,服务端响应信息为:" + reply);
reply = br.readLine();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (os != null) {
os.close();
}
if (socket != null) {
socket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
package Demo01;
import jdk.internal.util.xml.impl.Input;
import java.io.*;
import java.net.Socket;
import java.util.Scanner;
/**
* 客户端
*/
public class LoginClient1 {
public static void main(String[] args) {
while (true) {
Socket socket = null;
OutputStream os = null;
InputStream is = null;
BufferedReader br = null;
try {
//建立连接,指定服务器及端口
socket = new Socket("localhost", 8800);
//信息
Scanner input = new Scanner(System.in);
System.out.println("请输入:");
String str = input.next();
//得到输出流
os = socket.getOutputStream();
//写
os.write(str.getBytes());
socket.shutdownOutput(); //让流半关闭
//接收服务端响应
//得到输入流
is = socket.getInputStream();
//输出信息
br = new BufferedReader(new InputStreamReader(is));
String reply = br.readLine();
while (reply != null) {
System.out.println("我是客户端,服务端响应信息为:" + reply);
reply = br.readLine();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (os != null) {
os.close();
}
if (socket != null) {
socket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
package Demo01;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
/**
* 服务端
*/
public class LoginServer {
public static void main(String[] args) {
try {
ServerSocket serverSocket = new ServerSocket(8800);
while (true) {
Socket socket=serverSocket.accept();
//建一个服务端口
LoginThread loginThread=new LoginThread(socket);
loginThread.start();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
package Demo01;
import java.io.*;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.Scanner;
public class LoginThread extends Thread {
Socket socket = null;
public LoginThread(Socket socket) {
this.socket = socket;
}
public void run (){
InputStream is = null;
BufferedReader br = null;
OutputStream os = null;
try {
//得到输入流
is = socket.getInputStream();
//输出信息
br = new BufferedReader(new InputStreamReader(is));
String info = br.readLine();
while (info != null) {
System.out.println("我是服务端,客户端登录信息为:" + info);
info = br.readLine();
}
//给客户端响应
Scanner input = new Scanner(System.in);
System.out.println("请输入:");
String reply = input.next();
//得到输出流
os = socket.getOutputStream();
//写
os.write(reply.getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (br != null) {
br.close();
}
if (is != null) {
is.close();
}
if (os != null) {
os.close();
}
if (socket != null) {
socket.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}