主要思路:将矩阵分圈,即每次都输出最外面一层的矩形,每一圈分为四个序列,每一圈的边界都基于其左上角坐标(tR,tC)和右下角坐标(dR,dC)。
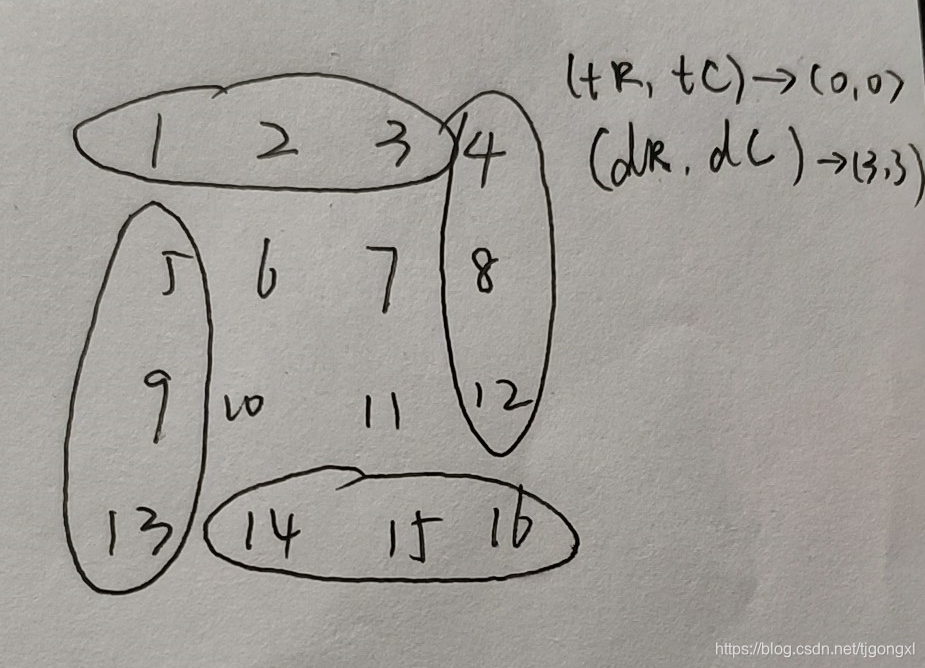
通过四个while循环,对边界条件的判断就可以打印完一圈,然后tR,tC加1,dR,dC减1。
到最后一圈可能会出现只剩一行或者只剩一列的情况,对应tR = dR 或 tC = dC,需要单独进行判断,而不需要四次循环打印。
class Solution {
public List<Integer> spiralOrder(int[][] matrix) {
List<Integer> res = new ArrayList<>();
int m = matrix.length;
if(m == 0) return res;
int n = matrix[0].length;
int tR = 0, tC = 0, dR = m - 1, dC = n - 1;
while(tR <= dR && tC <= dC) {
getElement(res, matrix, tR++, tC++, dR--, dC--);
}
return res;
}
public void getElement(List<Integer> res, int[][] matrix, int tR, int tC, int dR, int dC) {
if(tR == dR) { //只剩下一行
for(int i = tC; i <= dC; i++) {
res.add(matrix[tR][i]);
}
} else if(tC == dC) { //只剩下一列
for(int i = tR; i <= dR; i++) {
res.add(matrix[i][tC]);
}
} else {
int curR = tR;
int curC = tC;
while(curC != dC) { //左右
res.add(matrix[tR][curC]);
curC++;
}
while(curR != dR) { //上下
res.add(matrix[curR][dC]);
curR++;
}
while(curC != tC) { //右左
res.add(matrix[dR][curC]);
curC--;
}
while(curR != tR) { //下上
res.add(matrix[curR][tC]);
curR--;
}
}
}
}