-------------------------------------资源来源于网络,仅供自学使用,如有侵权,联系我必删.
第一:
使用 C++ 标准库
C++ 标准库并不是 C++ 语言的一部分
C++ 标准库是由 C++ 语言编写而成的类库和函数的集合
C++ 标准库中定义的类和对象都位于 std 命名空间中
C++ 标准库的头文件都不带 .h 后缀
C++ 标准库涵盖了C C 库的功能
• C 库中 <name.h> 头文件对应 C++ 中的<cname>
C++ 标准库预定义了多数常用的数据结构,如:字符串,链表,队列,栈等
• <bitset>
• <deque>
• <list>
• <map>
• <queue>
• <set>
• <stack>
• <vector>
#include <cstdio>
using namespace std;//打开std命名空间
int main()
{
printf("Hello World!\n");
printf("Press any key to continue...");
getchar();
return 0;
}
#include <iostream>
using namespace std;
int main()
{
//cout 表示输出
//endl 表示换行
cout<<"Hello World"<<endl;
int x;
int y;
//cin 表示输入
cout<<"1st Parameter: ";
cin>>x;
cout<<"2nd Parameter: ";
cin>>y;
cout<<"Result: "<<x+y<<endl;
return 0;
}
第二:
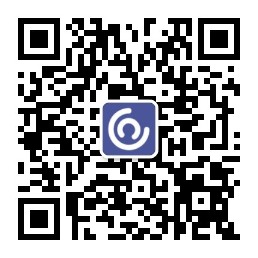
左移运算符 << 和 右移运算符 >> 在C 语言中只能用于整数运算,并且语义是确定不变的
操作符重载为操作符提供不同的语义
#include <cstdlib>
#include <iostream>
using namespace std;
struct Complex
{
int a;
int b;
};
int main(int argc, char *argv[])
{
Complex c1 = {1, 2};
Complex c2 = {3, 4};
Complex c3 = c1 + c2;//加法 不能用于二个结构体系相加
cout << "Press the enter key to continue ...";
cin.get();//C++ 库函数
return EXIT_SUCCESS;
}
可以定义一个 add 函数用于两个 Complex 的相加
#include <cstdlib>
#include <iostream>
using namespace std;
struct Complex
{
int a;
int b;
};
Complex add(const Complex& c1, const Complex& c2)//定义add函数 函数重载
{
Complex ret;
ret.a = c1.a + c2.a;
ret.b = c1.b + c2.b;
return ret;
}
int main(int argc, char *argv[])
{
Complex c1 = {1, 2};
Complex c2 = {3, 4};
Complex c3 = add(c1, c2);
cout<<"c3.a = "<<c3.a<<endl;
cout<<"c3.b = "<<c3.b<<endl;
cout << "Press the enter key to continue ...";
cin.get();
return EXIT_SUCCESS;
}
add 函数可以解决Complex 变量相加的问题,但是Complex 是现实世界中确实存在的复数,并且复数在数学中的地位和普通的实数相同
第三:
C++ 中操作符重载的本质
C++ 中通过 operator 关键字可以利用函数扩展操作符
operator的本质是通过函数重载实现操作符重载
#include <cstdlib>
#include <iostream>
using namespace std;
struct Complex
{
int a;
int b;
};
Complex add(const Complex& c1, const Complex& c2)
{
Complex ret;
ret.a = c1.a + c2.a;
ret.b = c1.b + c2.b;
return ret;
}
int main(int argc, char *argv[])
{
Complex c1 = {1, 2};
Complex c2 = {3, 4};
Complex c3 = add(c1, c2);
cout<<"c3.a = "<<c3.a<<endl;
cout<<"c3.b = "<<c3.b<<endl;
cout << "Press the enter key to continue ...";
cin.get();
return EXIT_SUCCESS;
}
用 operator 关键字扩展的操作符可以用于类吗?
第四:
C++ 中的类的 友元
private 声明使得类的成员不能被外界访问
但是通过 friend关键字可以例外的开放权限
#include <cstdlib>
#include <iostream>
using namespace std;
class Complex
{
int a;
int b;
public:
Complex(int a = 0, int b = 0)
{
this->a = a;
this->b = b;
}
int getA()
{
return a;
}
int getB()
{
return b;
}
friend Complex operator+ (const Complex& c1, const Complex& c2);//Complex函数 operator+操作符 友元
};
Complex operator+ (const Complex& c1, const Complex& c2)
{
Complex ret;
ret.a = c1.a + c2.a;
ret.b = c1.b + c2.b;
return ret;
}
int main(int argc, char *argv[])
{
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2;
cout << "Press the enter key to continue ...";
cin.get();
return EXIT_SUCCESS;
}
重载左移运算符
#include <cstdlib>
#include <iostream>
using namespace std;
class Complex
{
int a;
int b;
public:
Complex(int a = 0, int b = 0)
{
this->a = a;
this->b = b;
}
int getA()
{
return a;
}
int getB()
{
return b;
}
friend Complex operator+ (const Complex& c1, const Complex& c2);//声明友元
friend ostream& operator<< (ostream& out, const Complex& c);//重载左移操作符
};
ostream& operator<< (ostream& out, const Complex& c)//ostream&引用 支持C++库链式调用
{
out<<c.a<<" + "<<c.b<<"i";
return out;//返回out
}
Complex operator+ (const Complex& c1, const Complex& c2)
{
Complex ret;
ret.a = c1.a + c2.a;
ret.b = c1.b + c2.b;
return ret;
}
int main(int argc, char *argv[])
{
Complex c1(1, 2);
Complex c2(3, 4);
Complex c3 = c1 + c2;
cout<<c1<<endl;
cout<<c2<<endl;
cout<<c3<<endl;
cout << "Press the enter key to continue ...";
cin.get();
return EXIT_SUCCESS;
}
C++做了什么
C++ 是怎么改变左移运算符和右移运算符的语义的?
C++ 中的操作符重载可以改变左移和右移运算符的语义!
小结
操作符重载是 C++ 的强大特性之一
操作符重载的本质是通过函数扩展操作符的语义
operator 关键字是操作符重载的关键
friend 关键字可以对函数或类开发访问权限
操作符重载遵循函数重载的规则