问题描述:
Given a binary tree and a sum, find all root-to-leaf paths where each path's sum equals the given sum.
For example:
Given the below binary tree and sum = 22
,
5 / \ 4 8 / / \ 11 13 4 / \ / \ 7 2 5 1
return
[ [5,4,11,2], [5,8,4,5] ]
原问题链接:https://leetcode.com/problems/path-sum-ii/
问题分析
在这个问题的前一个问题里,其实已经讨论了path sum的判断和推导思路。我们要判断一棵树是否存在有从根到叶节点的路径所有元素的值等于目标元素,需要判断当前节点的值和目标值,然后递归的去推导它的左右子节点和它们的差值之间的比较。这样得到最终的结果。
而这里是要找出所有存在情况下的元素,我们需要遍历所有的情况。在问题的查找上,我们可以同样使用前面的递归情况,只是需要用一个List<List<Integer>>来保存最终的结果。同时也用一个List<Integer>来保存从根节点到某个节点的路径上的值。在每次递归的时候,我们构造一个新的拷贝传入到下一级的递归中。
详细的代码实现如下:
/** * Definition for a binary tree node. * public class TreeNode { * int val; * TreeNode left; * TreeNode right; * TreeNode(int x) { val = x; } * } */ public class Solution { public List<List<Integer>> pathSum(TreeNode root, int sum) { List<List<Integer>> result = new ArrayList<>(); pathSum(root, sum, result, new ArrayList<Integer>()); return result; } private void pathSum(TreeNode root, int sum, List<List<Integer>> result, List<Integer> list) { if(root == null) return; List<Integer> copy = new ArrayList<>(list); copy.add(root.val); if(root.val == sum && root.left == null && root.right == null) { result.add(copy); return; } pathSum(root.left, sum - root.val, result, copy); pathSum(root.right, sum - root.val, result, copy); } }
扫描二维码关注公众号,回复:
619785 查看本文章
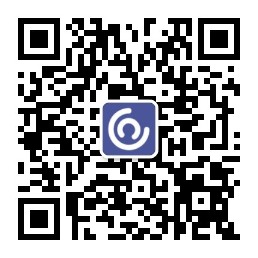