假如有以下代码:
1 using UnityEngine; 2 using UnityEditor; 3 4 public class LugsTest : MonoBehaviour 5 { 6 [SerializeField] 7 bool isEnabled; 8 9 [SerializeField] 10 string name; 11 }
将这个脚本直接挂到 GameObject 上的效果是:
这个是显而易见的答案。如果现在有一个需求,只在 Inspector 中显示代码中的部分变量,该如何做呢?这个就是这里要实现的内容。
额外多出两个脚本(其实多出一个就可以,只是这里想总结一套架构清晰的逻辑):
CustomInspector、LugsTestEditor
以下分别是两个脚本的内容:
1 using UnityEngine; 2 using UnityEditor; 3 4 public class CustomInspector : Editor 5 { 6 protected void DrawPropertyField(string filedName) 7 { 8 DrawPropertyField(filedName, true, true); 9 } 10 11 protected void DrawPropertyField(string fieldName, bool isValid, bool isValidWarning) 12 { 13 WrapWithValidationColor(() => 14 { 15 SerializedProperty property = serializedObject.FindProperty(fieldName); 16 EditorGUILayout.PropertyField(property); 17 }, isValid, isValidWarning); 18 } 19 20 protected void WrapWithValidationColor(System.Action method, bool isValid, bool isValidWarning) 21 { 22 Color colorBackup = GUI.color; 23 if (isValid == false) 24 { 25 GUI.color = Color.red; 26 } 27 else if (isValidWarning == false) 28 { 29 GUI.color = Color.yellow; 30 } 31 method.Invoke(); 32 GUI.color = colorBackup; 33 } 34 }
对以上脚本中第 31 行内容不理解的可以阅读: https://www.cnblogs.com/luguoshuai/p/10940879.html
1 using UnityEditor; 2 using UnityEngine; 3 4 5 [CustomEditor(typeof(LugsTest))] 6 public class LugsTestEditor : CustomInspector 7 { 8 public override void OnInspectorGUI() 9 { 10 DrawPropertyField("isEnabled"); 11 12 if (GUI.changed) 13 { 14 serializedObject.ApplyModifiedProperties(); 15 } 16 } 17 }
在工程中加入以上脚本后的结果是怎么样的呢?
扫描二维码关注公众号,回复:
6296688 查看本文章
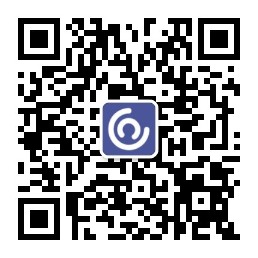
这个时候隐藏了 name 字段,如果同时隐藏定义的两个字段,该如何?只需修改 LugsTestEditor 中的内容即可。
1 using UnityEditor; 2 using UnityEngine; 3 4 5 [CustomEditor(typeof(LugsTest))] 6 public class LugsTestEditor : CustomInspector 7 { 8 public override void OnInspectorGUI() 9 { 10 //DrawPropertyField("isEnabled"); 11 //DrawPropertyField("name"); 12 13 if (GUI.changed) 14 { 15 serializedObject.ApplyModifiedProperties(); 16 } 17 } 18 }