1.MainActivity
public class MainActivity extends AppCompatActivity implements View.OnClickListener,DataCall, CompoundButton.OnCheckedChangeListener {
private EditText login_phone;
private EditText login_pwd;
private Button login;
Presenter presenter=new Presenter(MainActivity.this);
private SharedPreferences share;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
login_phone = findViewById(R.id.login_phone);
login_pwd = findViewById(R.id.login_pwd);
login = findViewById(R.id.login);
findViewById(R.id.login_reg).setOnClickListener(this);
findViewById(R.id.login_qq_btn).setOnClickListener(this);
login.setOnClickListener(this);
//获取复选框
CheckBox remPasCheck = findViewById(R.id.login_rem_pas);
//点击发生改变 记住密码
remPasCheck.setOnCheckedChangeListener(this);
//getSharedPreferences
share = getSharedPreferences("config", Context.MODE_PRIVATE);
//初始化为false 未选中状态
boolean remPas = share.getBoolean("login_rem_pas", false);
remPasCheck.setChecked(remPas);//还原记住密码状态
if (remPas){//如果选择了记住密码,则还原数据
login_phone.setText(share.getString("login_phone",""));
login_pwd.setText(share.getString("login_pwd",""));
}
}
@Override
public void onClick(View v) {
if(v.getId()==R.id.login) {
final String phone = login_phone.getText().toString();
final String pwd = login_pwd.getText().toString();
if (!StringUtil.isMobileNO(phone)){
Toast.makeText(this,"请输入正确手机号",Toast.LENGTH_LONG).show();
return;
}
if (pwd.length()<6){
Toast.makeText(this,"密码最少6位",Toast.LENGTH_LONG).show();
return;
}
//记住密码 初始化状态false
boolean remPas = share.getBoolean("login_rem_pas", false);
if (remPas){
SharedPreferences.Editor edit = share.edit();
edit.putString("login_phone",phone);
edit.putString("login_pwd",pwd);
edit.commit();
}
presenter.login(phone, pwd);
//跳转页面
Intent intent=new Intent(MainActivity.this,Main2Activity.class);
startActivity(intent);
finish();
}else if (v.getId()==R.id.login_reg){//点击注册按钮
Intent intent = new Intent(this,RegActivity.class);
startActivity(intent);
}else if(v.getId()==R.id.login_qq_btn){
if(Build.VERSION.SDK_INT>=22){//QQ需要申请写入权限
String[] mPermissionList = new String[]{Manifest.permission.WRITE_EXTERNAL_STORAGE,Manifest.permission.ACCESS_FINE_LOCATION,Manifest.permission.CALL_PHONE,Manifest.permission.READ_LOGS,Manifest.permission.READ_PHONE_STATE, Manifest.permission.READ_EXTERNAL_STORAGE,Manifest.permission.SET_DEBUG_APP,Manifest.permission.SYSTEM_ALERT_WINDOW,Manifest.permission.GET_ACCOUNTS,Manifest.permission.WRITE_APN_SETTINGS};
ActivityCompat.requestPermissions(this,mPermissionList,123);
}else{
UMShareAPI.get(this).getPlatformInfo(this, SHARE_MEDIA.QQ, authListener);
}
}
}
@Override
public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) {
if (buttonView.getId()==R.id.login_rem_pas){
SharedPreferences.Editor edit = share.edit();
if (!isChecked){//记录密码
edit.remove(“login_phone”);//移除手机号
edit.remove(“login_pwd”);//移除密码
}
edit.putBoolean(“login_rem_pas”,isChecked);
edit.commit();
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode==123){
UMShareAPI.get(this).getPlatformInfo(this, SHARE_MEDIA.QQ, authListener);
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
UMShareAPI.get(this).onActivityResult(requestCode, resultCode, data);
}
2.布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<EditText
android:id="@+id/login_phone"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入手机号"
android:padding="10dp" />
<EditText
android:id="@+id/login_pwd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入密码" />
<CheckBox
android:id="@+id/login_rem_pas"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="记住密码" />
<Button
android:id="@+id/login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="登录" />
<Button
android:id="@+id/login_qq_btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="QQ登录"/>
<Button
android:id="@+id/login_reg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="注册"/>
</LinearLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".RegActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="手机号"/>
<EditText
android:id="@+id/reg_mobile"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="请输入手机号"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="密码"/>
<EditText
android:id="@+id/reg_pas"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:hint="请输入密码"/>
</LinearLayout>
<Button
android:id="@+id/reg_btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="注册"/>
</LinearLayout>
3.RegActivity注册
package com.example.day08mvp;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.EditText;
import android.widget.Toast;
import com.example.day08mvp.bean.Result;
import com.example.day08mvp.core.DataCall;
import com.example.day08mvp.presenter.Presenter;
import com.example.day08mvp.presenter.RegisterPresenter;
import com.example.day08mvp.util.StringUtil;
public class RegActivity extends AppCompatActivity implements View.OnClickListener,DataCall {
private EditText mMobile,mPas;
private RegisterPresenter presenter=new RegisterPresenter(this);
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_reg);
mMobile = findViewById(R.id.reg_mobile);
mPas = findViewById(R.id.reg_pas);
findViewById(R.id.reg_btn).setOnClickListener(this);
}
@Override
public void onClick(View v) {
String mobile = mMobile.getText().toString();
String pas = mPas.getText().toString();
if (!StringUtil.isMobileNO(mobile)){
Toast.makeText(this,"请输入正确手机号",Toast.LENGTH_LONG).show();
return;
}
if (pas.length()<6){
Toast.makeText(this,"密码最少6位",Toast.LENGTH_LONG).show();
return;
}
presenter.login(mobile,pas);
}
@Override
public void callback(Result data) {
Toast.makeText(this,"注册成功,请登录",Toast.LENGTH_LONG).show();
finish();
}
}
4.在presenter里新建一个RegisterPresenter用于注册
package com.example.day08mvp.presenter;
import android.os.Handler;
import android.os.Message;
import com.example.day08mvp.bean.Result;
import com.example.day08mvp.core.DataCall;
import com.example.day08mvp.model.Model;
public class RegisterPresenter {
Model model=new Model();
private DataCall call;
public RegisterPresenter(DataCall dataCall){
call=dataCall;
}
private Handler handler=new Handler(){
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
Result result= (Result) msg.obj;
// Toast.makeText(MainActivity.this,result.getCode()+result.getMsg(),Toast.LENGTH_LONG).show();
call.callback(result);//接口的回调页面
}
};
public void login(final String phone, final String pwd) {
new Thread(){
@Override
public void run() {
super.run();
// String strjson="http://www.zhaoapi.cn/user/login?mobile="+phone+"&password="+pwd;
Result result = model.login(phone, pwd);
Message message=handler.obtainMessage();
message.obj=result;
handler.sendMessage(message);
}
}.start();
}
}
5.手机号正则表达式
package com.example.day08mvp.util;
import android.text.TextUtils;
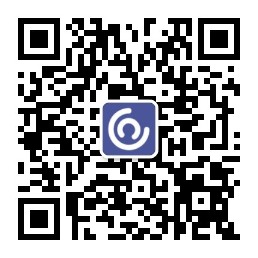
public class StringUtil {
public static boolean isMobileNO(String mobiles) {
/*
移动:134、135、136、137、138、139、150、151、147(TD)、157(TD)、158、159、178、187、188
联通:130、131、132、152、155、156、176、185、186
电信:133、153、177、180、189、(1349卫通)
总结起来就是第一位必定为1,第二位必定为3或5或8,其他位置的可以为0-9
*/
String telRegex = “[1][34578]\d{9}”;//"[1]“代表第1位为数字1,”[4578]“代表第二位可以为3、4、5、7、8中的一个,”\d{9}"代表后面是可以是0~9的数字,有9位。
if (TextUtils.isEmpty(mobiles)) {
return false;
} else {
return mobiles.matches(telRegex);
}
}
}