numpy API:
flip:
flip(m, 0) is equivalent to flipud(m).
flip(m, 1) is equivalent to fliplr(m).
flip(m, n) corresponds to m[...,::-1,...]
with ::-1
at position n.
flip(m) corresponds to m[::-1,::-1,...,::-1]
with ::-1
at all positions.
flip(m, (0, 1)) corresponds to m[::-1,::-1,...]
with ::-1
at position 0 and position 1.
>>> A = np.arange(8).reshape((2,2,2)) >>> A array([[[0, 1], [2, 3]], [[4, 5], [6, 7]]]) >>> flip(A, 0) array([[[4, 5], [6, 7]], [[0, 1], [2, 3]]]) >>> flip(A, 1) array([[[2, 3], [0, 1]], [[6, 7], [4, 5]]]) >>> np.flip(A) array([[[7, 6], [5, 4]], [[3, 2], [1, 0]]]) >>> np.flip(A, (0, 2)) array([[[5, 4], [7, 6]], [[1, 0], [3, 2]]]) >>> A = np.random.randn(3,4,5) >>> np.all(flip(A,2) == A[:,:,::-1,...]) True
flipud: (==flip(m, 1) )
Flip array in the up/down direction.
Flip the entries in each column in the up/down direction. Rows are preserved, but appear in a different order than before.
Equivalent to m[::-1,...]
. Does not require the array to be two-dimensional.
>>> A = np.diag([1.0, 2, 3]) >>> A array([[ 1., 0., 0.], [ 0., 2., 0.], [ 0., 0., 3.]]) >>> np.flipud(A) array([[ 0., 0., 3.], [ 0., 2., 0.], [ 1., 0., 0.]]) >>> >>> A = np.random.randn(2,3,5) >>> np.all(np.flipud(A) == A[::-1,...]) True >>> >>> np.flipud([1,2]) array([2, 1])
扫描二维码关注公众号,回复:
6706807 查看本文章
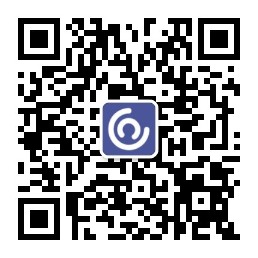
fliplr: (==flip(m, 0))
Equivalent to m[:,::-1]. Requires the array to be at least 2-D.
Flip array in the left/right direction.
rot90
Rotate array counterclockwise.
>>> A = np.diag([1.,2.,3.]) >>> A array([[ 1., 0., 0.], [ 0., 2., 0.], [ 0., 0., 3.]]) >>> np.fliplr(A) array([[ 0., 0., 1.], [ 0., 2., 0.], [ 3., 0., 0.]]) >>> >>> A = np.random.randn(2,3,5) >>> np.all(np.fliplr(A) == A[:,::-1,...]) True