目录
applicationContext_service.xml
applicationContext_transaction.xml
这里需要maven的基础:https://blog.csdn.net/qq_40323256/article/details/90168191
ssm的基础:https://blog.csdn.net/qq_40323256/article/details/89791744
bootstrap的基础:https://blog.csdn.net/qq_40323256/article/details/91490905
MySQL的基础:https://blog.csdn.net/qq_40323256/article/details/82905734
注意:此项目和这个项目都能实现同样的功能,不同点就是这个项目并没有使用maven,所有的jar包都是自己导入的。这个项目是dynamic web project,那个项目是maven project
一,运行效果
先来看一下最终运行效果
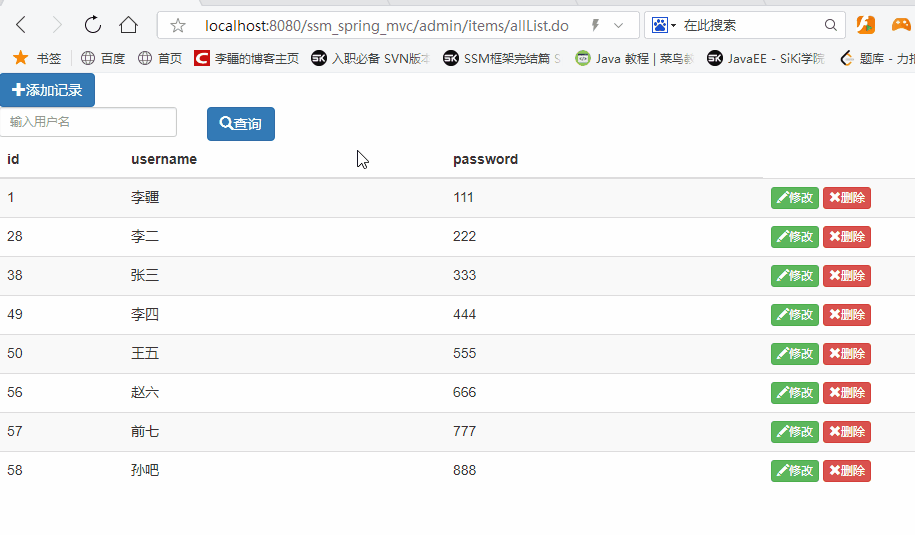
二,新建dynamic web project
先看下结构
注意:jar包记得build path
三,详细代码
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1">
<display-name>ssm_spring_mvc</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<!-- 静态资源放行 -->
<servlet-mapping>
<servlet-name>default</servlet-name>
<url-pattern>*.css</url-pattern>
<url-pattern>*.ttf</url-pattern>
<url-pattern>*.woff</url-pattern>
<url-pattern>*.js</url-pattern>
<url-pattern>*.png</url-pattern>
<url-pattern>*.jpg</url-pattern>
<url-pattern>*.gif</url-pattern>
</servlet-mapping>
<!-- 过滤器 解决表单post提交乱码问题 -->
<filter>
<filter-name>encoding</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>utf-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encoding</filter-name>
<!-- 拦截全部 /* -->
<url-pattern>/*</url-pattern>
</filter-mapping>
<!-- 配置springmvc前端控制器 和读取配置文件 -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<!-- 读取配置文件 -->
<param-name>contextConfigLocation</param-name>
<param-value>classpath:config/springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<!-- / -->
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- 监听器 加载spring的配置文件 -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:config/applicationContext_*.xml</param-value>
</context-param>
</web-app>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.3.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- 开启注解扫描 -->
<context:component-scan base-package="com.lijiang.controller"/>
<!-- 开启注解驱动 -->
<mvc:annotation-driven/>
<!-- 配置视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/jsp/"/>
<property name="suffix" value=".jsp"/>
</bean>
<!-- 图片上传 -->
<bean name="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver"/>
</beans>
applicationContext_mapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- 读取配置文件 数据库 -->
<context:property-placeholder location="classpath:config/db.properties" ignore-unresolvable="true"/>
<!-- 配置数据源 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driverClass}"/>
<property name="jdbcUrl" value="${jdbc.jdbcUrl}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<!-- 配置mybatis -->
<bean name="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.lijiang.bean"/>
</bean>
<!-- mapper工厂 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.lijiang.mapper"/>
</bean>
</beans>
applicationContext_service.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- 读取配置文件 数据库 -->
<context:property-placeholder location="classpath:config/db.properties" ignore-unresolvable="true"/>
<!-- 配置数据源 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driverClass}"/>
<property name="jdbcUrl" value="${jdbc.jdbcUrl}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<!-- 配置mybatis -->
<bean name="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.lijiang.bean"/>
</bean>
<!-- mapper工厂 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.lijiang.mapper"/>
</bean>
</beans>
applicationContext_transaction.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.3.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<!-- 读取配置文件 数据库 -->
<context:property-placeholder location="classpath:config/db.properties" ignore-unresolvable="true"/>
<!-- 配置数据源 -->
<bean name="dataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="driverClass" value="${jdbc.driverClass}"/>
<property name="jdbcUrl" value="${jdbc.jdbcUrl}"/>
<property name="user" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<!-- 配置mybatis -->
<bean name="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.lijiang.bean"/>
</bean>
<!-- mapper工厂 -->
<bean class="org.mybatis.spring.mapper.MapperScannerConfigurer">
<property name="basePackage" value="com.lijiang.mapper"/>
</bean>
</beans>
db.properties
jdbc.driverClass=com.mysql.jdbc.Driver
jdbc.jdbcUrl=jdbc:mysql://localhost:3306/ssm
jdbc.username=root
jdbc.password=root
ItemInfo.java
package com.lijiang.bean;
public class ItemInfo {
//id
private Integer id;
//username
private String username;
private String password;
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public ItemInfo(Integer id, String username, String password) {
super();
this.id = id;
this.username = username;
this.password = password;
}
}
ItemController.java
package com.lijiang.controller;
import java.util.ArrayList;
import java.util.List;
import org.apache.ibatis.annotations.Param;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.servlet.ModelAndView;
import com.lijiang.bean.ItemInfo;
import com.lijiang.service.ItemService;
@Controller
@RequestMapping("/admin/items")
public class ItemController {
@Autowired
private ItemService itemService;
// 显示所有列表
@RequestMapping("allList.do")
public String selectAll(Model model,ItemInfo itemInfo){
List<ItemInfo> itemList=itemService.selectAll(itemInfo);
model.addAttribute("itemList", itemList);
return "item_list";
}
// 删除
@RequestMapping("delete.do")
public String delete(Integer id){
itemService.deleteById(id);
return "forward:allList.do";
}
// 添加
@RequestMapping("save.do")
public String save(ItemInfo itemInfo){
itemService.saveItem(itemInfo);
return "forward:allList.do";
}
// 修改
@RequestMapping("update.do")
public String update(ItemInfo itemInfo){
itemService.updateItem(itemInfo);
return "forward:allList.do";
}
// 编辑 回显数据
@RequestMapping("edit.do")
@ResponseBody
public ItemInfo edit(Integer id) {
return itemService.selectItemInfoById(id);
}
}
ItemMapper.java
package com.lijiang.mapper;
import java.util.List;
import com.lijiang.bean.ItemInfo;
public interface ItemMapper {
List<ItemInfo> selectAll(ItemInfo itemInfo);
void deleteById(Integer id);
void saveItem(ItemInfo itemInfo);
void updateItem(ItemInfo itemInfo);
ItemInfo selectItemInfoById(Integer id);
}
ItemMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.lijiang.mapper.ItemMapper">
<!-- List<ItemInfo> selectAll(ItemInfo itemInfo); -->
<select id="selectAll" resultType="ItemInfo" parameterType="ItemInfo">
select * from user
<where>
<if test="username != null and username != ''">
and username LIKE "%"#{username}"%"
</if>
</where>
</select>
<!-- void deleteById(Integer id); -->
<delete id="deleteById" parameterType="Integer">
delete from user where id=#{id}
</delete>
<!-- void saveItem(ItemInfo itemInfo); -->
<insert id="saveItem" parameterType="ItemInfo">
insert into user values(#{id},#{username}, #{password});
</insert>
<!-- void updateItem(ItemInfo itemInfo); -->
<update id="updateItem" parameterType="ItemInfo">
update user
<set>
<if test="username != null and username != ''">username = #{username},</if>
<if test="password != null and password != ''">password = #{password}</if>
</set>
where id = #{id}
</update>
<!-- ItemInfo selectItemInfoById(Integer id); -->
<select id="selectItemInfoById" parameterType="Integer" resultType="ItemInfo">
select * from user where id=#{id}
</select>
</mapper>
ItemService.java
package com.lijiang.service;
import java.util.List;
import com.lijiang.bean.ItemInfo;
public interface ItemService {
List<ItemInfo> selectAll(ItemInfo itemInfo);
void deleteById(Integer id);
void saveItem(ItemInfo itemInfo);
void updateItem(ItemInfo itemInfo);
ItemInfo selectItemInfoById(Integer id);
}
ItemServicelmpl.java
package com.lijiang.service;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.lijiang.bean.ItemInfo;
import com.lijiang.mapper.ItemMapper;
@Service
public class ItemServicelmpl implements ItemService {
@Autowired
private ItemMapper itemMapper;
@Override
public List<ItemInfo> selectAll(ItemInfo itemInfo) {
return itemMapper.selectAll(itemInfo);
}
@Override
public void deleteById(Integer id) {
itemMapper.deleteById(id);
}
@Override
public void saveItem(ItemInfo itemInfo) {
itemMapper.saveItem(itemInfo);
}
@Override
public void updateItem(ItemInfo itemInfo) {
itemMapper.updateItem(itemInfo);
}
@Override
public ItemInfo selectItemInfoById(Integer id) {
return itemMapper.selectItemInfoById(id);
}
}
item_list.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<script src="${pageContext.request.contextPath }/js/jquery-3.3.1.min.js"></script>
<link href="${pageContext.request.contextPath }/css/bootstrap.min.css" rel="stylesheet">
<script src="${pageContext.request.contextPath }/js/bootstrap.min.js"></script>
</head>
<body>
<table class="table table-hover table table-striped">
<thead>
<th>id</th>
<th>username</th>
<th>password</th>
</thead>
<tbody>
<c:forEach items="${itemList}" var="item">
<tr>
<td>${item.id}</td>
<td>${item.username}</td>
<td>${item.password}</td>
<td>
<button class="btn btn-success btn-xs" data-toggle="modal" data-target="#editLayer" onclick="editItems(${item.id})"><span class="glyphicon glyphicon-pencil"></span>修改</button>
<button class="btn btn-danger btn-xs" onclick="deleteItem(${item.id})"><span class="glyphicon glyphicon-remove"></span>删除</button>
</td>
</tr>
</c:forEach>
<!-- 记录添加弹出层 -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target="#addLayer"> <span class="glyphicon glyphicon-plus"></span>添加记录 </button>
<div class="modal fade" id="addLayer" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button data-dismiss="modal" class="close" type="button"><span aria-hidden="true">×</span><span class="sr-only">Close</span></button>
<h4 class="modal-title">添加记录</h4>
</div>
<div class="modal-body">
<form id="add_items_form" class="form-horizontal">
<input type="hidden" class="form-control" name="id"> <br>
<input type="text" class="form-control" placeholder="用户名" name="username"> <br>
<input type="password" class="form-control" placeholder="密码" name="password">
</form>
</div>
<div class="modal-footer">
<button data-dismiss="modal" class="btn btn-default" type="button">关闭</button>
<button class="btn btn-primary" type="button" onclick="addItem()">确认添加</button>
</div>
</div>
</div>
</div>
<!-- <div style="height:200px"></div> -->
<!-- 查询记录 -->
<form id="search_form" action="${pageContext.request.contextPath }/admin/items/allList.do" method="post">
<div class="row">
<div class="col-md-2">
<input type="text" id="search_item_name" class="form-control input-sm" placeholder="输入用户名" name="username" value="">
</div>
<div class="col-md-9">
<button id="search_btn" type="submit" class="btn btn-primary"><span class="glyphicon glyphicon-search"></span>查询</button>
</div>
</div>
</form>
<!-- 记录修改弹出层 -->
<div class="modal fade" id="editLayer" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button data-dismiss="modal" class="close" type="button"><span aria-hidden="true">×</span><span class="sr-only">Close</span></button>
<h4 class="modal-title">修改记录</h4>
</div>
<div class="modal-body">
<form id="edit_items_form" class="form-horizontal">
<input type="hidden" id="edit_item_id" class="form-control" name="id"> <br>
<input type="text" id="edit_item_username" class="form-control" placeholder="用户名" name="username"> <br>
<input type="password" id="edit_item_password" class="form-control" placeholder="密码" name="password">
</form>
</div>
<div class="modal-footer">
<button data-dismiss="modal" class="btn btn-default" type="button">关闭</button>
<button class="btn btn-primary" type="button" onclick="updateItem()">确认修改</button>
</div>
</div>
</div>
</div>
<!-- <div style="height:200px"></div> -->
</tbody>
</table>
</body>
<script type="text/javascript">
$(function(){
init();
})
function init(){
// alert("初始化界面...");
}
// 删除
function deleteItem(id){
if(confirm("确定要删除该记录吗?")){
$.post(
"${pageContext.request.contextPath }/admin/items/delete.do",
{"id":id},
function(){
alert("删除成功!");
window.location.reload();
}
);
}
}
//添加记录
function addItem(){
$.ajax({
type:"POST",
data:new FormData($("#add_items_form")[0]),
url:"${pageContext.request.contextPath }/admin/items/save.do",
cache:false,
contentType:false,
processData:false,
success:function(){
alert("记录添加成功!");
window.location.reload();
}
});
}
// “确认修改”按钮的点击事件
function updateItem(){
$.ajax({
type:"POST",
data:new FormData($("#edit_items_form")[0]),
url:"${pageContext.request.contextPath }/admin/items/update.do",
cache:false,
contentType:false,
processData:false,
success:function(){
alert("修改成功!");
window.location.reload();
}
});
}
//点击“修改”按钮,打开编辑窗口,回显数据
function editItems(id){
$("#edit_items_form")[0].reset();
$.ajax({
type:"POST",
data:{"id":id},
url:"${pageContext.request.contextPath }/admin/items/edit.do",
dataType:"json",
success:function(data){
$("#edit_item_id").val(data.id);
$("#edit_item_username").val(data.username);
$("#edit_item_password").val(data.password);
}
});
}
</script>
</html>
四,MySQL数据库文件
五,运行
右键项目-【run as】-【run on server】
六,完整项目下载
csdn下载:https://download.csdn.net/download/qq_40323256/11243041
GitHub下载:https://github.com/LiJiangJiangJiang/ssm_spring_mvc