基于外键关联的单向一对多关联是一种很少见的情况,并不推荐使用。
基于连接表的单向一对多关联 应该优先被采用。请注意,通过指定unique="true",我们可以把多样性从多对多改变为一对多。
摘自Hibernate Reference
Product与Category是多对一的关系,Product对象维护着对Category对象的参考,如果由Category对象维护对多个Product对象的管理,就是一对多单向关联。
多对一就是从Product端来看,多个产品属于一个分类,每个产品都需要维护各自的Category对象。
而一对多就是从Category端来看,一个Category下有多个分类,Category需要维护多个Product信息。
嘿,不好意思,各位看官,因为恢复系统把之前做的物理数据模型图弄丢了,本来打算一并附上的,现在搞不到了,呵呵。
SQL脚本:(附件中可下载)
-- MySQL dump 10.13 Distrib 5.1.55, for Win32 (ia32) -- -- Host: localhost Database: hibernate_demo -- ------------------------------------------------------ -- Server version 5.1.55-community /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8 */; /*!40103 SET @OLD_TIME_ZONE=@@TIME_ZONE */; /*!40103 SET TIME_ZONE='+00:00' */; /*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */; /*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */; /*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */; /*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */; -- -- Table structure for table `category` -- DROP TABLE IF EXISTS `category`; /*!40101 SET @saved_cs_client = @@character_set_client */; /*!40101 SET character_set_client = utf8 */; CREATE TABLE `category` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(30) NOT NULL, `description` varchar(30) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM AUTO_INCREMENT=9 DEFAULT CHARSET=latin1; /*!40101 SET character_set_client = @saved_cs_client */; -- -- Dumping data for table `category` -- LOCK TABLES `category` WRITE; /*!40000 ALTER TABLE `category` DISABLE KEYS */; INSERT INTO `category` VALUES (1,'fruit2','fruit category2'); /*!40000 ALTER TABLE `category` ENABLE KEYS */; UNLOCK TABLES; -- -- Table structure for table `product` -- DROP TABLE IF EXISTS `product`; /*!40101 SET @saved_cs_client = @@character_set_client */; /*!40101 SET character_set_client = utf8 */; CREATE TABLE `product` ( `id` int(11) NOT NULL, `name` varchar(30) NOT NULL, `price` int(11) NOT NULL, `description` varchar(30) NOT NULL, `category_id` int(11) DEFAULT NULL, PRIMARY KEY (`id`), KEY `category_id` (`category_id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1; /*!40101 SET character_set_client = @saved_cs_client */; -- -- Dumping data for table `product` -- LOCK TABLES `product` WRITE; /*!40000 ALTER TABLE `product` DISABLE KEYS */; /*!40000 ALTER TABLE `product` ENABLE KEYS */; UNLOCK TABLES; /*!40103 SET TIME_ZONE=@OLD_TIME_ZONE */; /*!40101 SET SQL_MODE=@OLD_SQL_MODE */; /*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */; /*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */; /*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */; -- Dump completed on 2012-08-16 22:20:47
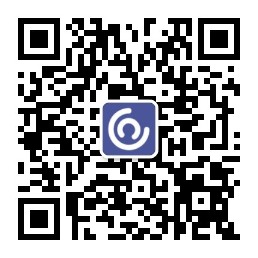
//Category.java
package com.zyp.examples; import java.util.HashSet; import java.util.Set; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.JoinColumn; import javax.persistence.OneToMany; import javax.persistence.Table; import javax.persistence.CascadeType; import org.hibernate.annotations.GenericGenerator; /** * Category entity. @author MyEclipse Persistence Tools */ @Entity @Table(name="category") public class Category implements java.io.Serializable { // Fields private static final long serialVersionUID = 411764056858459654L; @Id @GenericGenerator(name="incrementGenerator", strategy="increment") @GeneratedValue(generator="incrementGenerator", strategy=GenerationType.IDENTITY) @Column(name="id") private Integer id; @Column(name="name", nullable=false) private String name; @Column(name="description", nullable=false) private String description; @OneToMany(targetEntity=Product.class, cascade=CascadeType.ALL) @JoinColumn(name="category_id") private Set<Product> products = new HashSet<Product>(); // Constructors /** default constructor */ public Category() { } /** full constructor */ public Category(String name, String description) { this.name = name; this.description = description; } // Property accessors public Integer getId() { return this.id; } public void setId(Integer id) { this.id = id; } public String getName() { return this.name; } public void setName(String name) { this.name = name; } public String getDescription() { return this.description; } public void setDescription(String description) { this.description = description; } public Set<Product> getProducts() { return products; } public void setProducts(Set<Product> products) { this.products = products; } }
//Product.java
package com.zyp.examples; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.GenerationType; import javax.persistence.Id; import javax.persistence.Table; import org.hibernate.annotations.GenericGenerator; /** * Product entity. @author MyEclipse Persistence Tools */ @Entity @Table(name="product") public class Product implements java.io.Serializable { // Fields private static final long serialVersionUID = -3046977533702585304L; @Id @GenericGenerator(name="incrementGenerator", strategy="increment") @GeneratedValue(generator="incrementGenerator", strategy=GenerationType.IDENTITY) @Column(name="id") private Integer id; @Column(name="name", nullable=false) private String name; @Column(name="price", nullable=false) private Integer price; @Column(name="description",nullable=false) private String description; // Constructors /** default constructor */ public Product() { } // Property accessors public Integer getId() { return this.id; } public void setId(Integer id) { this.id = id; } public String getName() { return this.name; } public void setName(String name) { this.name = name; } public Integer getPrice() { return this.price; } public void setPrice(Integer price) { this.price = price; } public String getDescription() { return this.description; } public void setDescription(String description) { this.description = description; } }
//hibernate.cfg.xml
这个就不弄了,都一样
//HibernateUtil.java
也不弄了
//HibernateTest.java
package com.zyp.examples; import java.util.HashSet; import java.util.Set; import org.hibernate.Session; import org.hibernate.Transaction; public class HibernateTest { public static void main(String[] args) { //同时保存分类和产品 addAll(); //根据已有分类保存产品 //先添加分类 // addCategory(); // addProduct(); //级联删除 // deleteByCategory(); } public static void deleteByCategory() { Session session = HibernateUtil.getSessionFactory().openSession(); Transaction tx = session.beginTransaction(); Category cat = (Category) session.load(Category.class, new Integer(1)); session.delete(cat); tx.commit(); session.close(); HibernateUtil.shutdown(); } public static void addAll() { Product product = new Product(); product.setDescription("apple2"); product.setName("apple2"); product.setPrice(10); Product product1 = new Product(); product1.setDescription("orige2"); product1.setName("orige2"); product1.setPrice(20); Category category = new Category(); category.setDescription("fruit category2"); category.setName("fruit2"); Set<Product> products = new HashSet<Product>(); products.add(product); products.add(product1); category.setProducts(products); Session session = HibernateUtil.getSessionFactory().openSession(); Transaction tx = session.beginTransaction(); session.save(category); //启用级联操作 // session.save(product); // session.save(product1); tx.commit(); session.close(); HibernateUtil.shutdown(); } public static void addCategory() { Category category = new Category(); category.setName("fruit"); category.setDescription("fruit category"); Session session = HibernateUtil.getSessionFactory().openSession(); Transaction tx = session.beginTransaction(); session.save(category); tx.commit(); HibernateUtil.shutdown(); } public static void addProduct() { Product product = new Product(); product.setDescription("banana"); product.setName("banana"); product.setPrice(10); Product product1 = new Product(); product1.setDescription("tom"); product1.setName("tom"); product1.setPrice(20); Session session = HibernateUtil.getSessionFactory().openSession(); Transaction tx = session.beginTransaction(); Category category = (Category)session.load(Category.class, new Integer(1)); Set<Product> products = new HashSet<Product>(); products.add(product); products.add(product1); category.setProducts(products); session.save(category); //启用级联操作 // session.save(product); // session.save(product1); tx.commit(); session.close(); HibernateUtil.shutdown(); } }
=================== 悲剧的分界线 =========================
中间出了一个错误,困扰了我一个晚上,本来昨晚就应该发布这个文章的。哎,找了这么大一个互联网,就没一篇帖子给出我想要的答案,我晕,难道你们只知道双向关联,不知道单向关联么。。。。
仔细一想,之前的xml配置的时候貌似隐隐的出现了这样的错误,马上解决了。看起来,学习注解的时候,参考一下xml的配置还是有必要的。
Hibernate:
insert
into
product
(description, name, price, id)
values
(?, ?, ?, ?)
22:16:41,718 DEBUG AbstractBatcher:66 - Executing batch size: 2
22:16:41,718 DEBUG AbstractBatcher:418 - about to close PreparedStatement (open PreparedStatements: 1, globally: 1)
22:16:41,718 DEBUG JDBCExceptionReporter:92 - Could not execute JDBC batch update [insert into product (description, name, price, id) values (?, ?, ?, ?)]
java.sql.BatchUpdateException: Field 'category_id' doesn't have a default value
at com.mysql.jdbc.PreparedStatement.executeBatchSerially(PreparedStatement.java:2007)
at com.mysql.jdbc.PreparedStatement.executeBatch(PreparedStatement.java:1443)
at org.hibernate.jdbc.BatchingBatcher.doExecuteBatch(BatchingBatcher.java:70)
at org.hibernate.jdbc.AbstractBatcher.executeBatch(AbstractBatcher.java:268)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:266)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:167)
at org.hibernate.event.def.AbstractFlushingEventListener.performExecutions(AbstractFlushingEventListener.java:321)
at org.hibernate.event.def.DefaultFlushEventListener.onFlush(DefaultFlushEventListener.java:50)
at org.hibernate.impl.SessionImpl.flush(SessionImpl.java:1028)
at org.hibernate.impl.SessionImpl.managedFlush(SessionImpl.java:366)
at org.hibernate.transaction.JDBCTransaction.commit(JDBCTransaction.java:137)
at com.zyp.examples.HibernateTest.addAll(HibernateTest.java:67)
at com.zyp.examples.HibernateTest.main(HibernateTest.java:14)
22:16:41,718 WARN JDBCExceptionReporter:100 - SQL Error: 1364, SQLState: HY000
22:16:41,718 ERROR JDBCExceptionReporter:101 - Field 'category_id' doesn't have a default value
22:16:41,718 ERROR AbstractFlushingEventListener:324 - Could not synchronize database state with session
org.hibernate.exception.GenericJDBCException: Could not execute JDBC batch update
at org.hibernate.exception.SQLStateConverter.handledNonSpecificException(SQLStateConverter.java:126)
at org.hibernate.exception.SQLStateConverter.convert(SQLStateConverter.java:114)
at org.hibernate.exception.JDBCExceptionHelper.convert(JDBCExceptionHelper.java:66)
at org.hibernate.jdbc.AbstractBatcher.executeBatch(AbstractBatcher.java:275)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:266)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:167)
at org.hibernate.event.def.AbstractFlushingEventListener.performExecutions(AbstractFlushingEventListener.java:321)
at org.hibernate.event.def.DefaultFlushEventListener.onFlush(DefaultFlushEventListener.java:50)
at org.hibernate.impl.SessionImpl.flush(SessionImpl.java:1028)
at org.hibernate.impl.SessionImpl.managedFlush(SessionImpl.java:366)
at org.hibernate.transaction.JDBCTransaction.commit(JDBCTransaction.java:137)
at com.zyp.examples.HibernateTest.addAll(HibernateTest.java:67)
at com.zyp.examples.HibernateTest.main(HibernateTest.java:14)
Caused by: java.sql.BatchUpdateException: Field 'category_id' doesn't have a default value
at com.mysql.jdbc.PreparedStatement.executeBatchSerially(PreparedStatement.java:2007)
at com.mysql.jdbc.PreparedStatement.executeBatch(PreparedStatement.java:1443)
at org.hibernate.jdbc.BatchingBatcher.doExecuteBatch(BatchingBatcher.java:70)
at org.hibernate.jdbc.AbstractBatcher.executeBatch(AbstractBatcher.java:268)
... 9 more
Exception in thread "main" org.hibernate.exception.GenericJDBCException: Could not execute JDBC batch update
at org.hibernate.exception.SQLStateConverter.handledNonSpecificException(SQLStateConverter.java:126)
at org.hibernate.exception.SQLStateConverter.convert(SQLStateConverter.java:114)
at org.hibernate.exception.JDBCExceptionHelper.convert(JDBCExceptionHelper.java:66)
at org.hibernate.jdbc.AbstractBatcher.executeBatch(AbstractBatcher.java:275)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:266)
at org.hibernate.engine.ActionQueue.executeActions(ActionQueue.java:167)
at org.hibernate.event.def.AbstractFlushingEventListener.performExecutions(AbstractFlushingEventListener.java:321)
at org.hibernate.event.def.DefaultFlushEventListener.onFlush(DefaultFlushEventListener.java:50)
at org.hibernate.impl.SessionImpl.flush(SessionImpl.java:1028)
at org.hibernate.impl.SessionImpl.managedFlush(SessionImpl.java:366)
at org.hibernate.transaction.JDBCTransaction.commit(JDBCTransaction.java:137)
at com.zyp.examples.HibernateTest.addAll(HibernateTest.java:67)
at com.zyp.examples.HibernateTest.main(HibernateTest.java:14)
Caused by: java.sql.BatchUpdateException: Field 'category_id' doesn't have a default value
at com.mysql.jdbc.PreparedStatement.executeBatchSerially(PreparedStatement.java:2007)
at com.mysql.jdbc.PreparedStatement.executeBatch(PreparedStatement.java:1443)
at org.hibernate.jdbc.BatchingBatcher.doExecuteBatch(BatchingBatcher.java:70)
at org.hibernate.jdbc.AbstractBatcher.executeBatch(AbstractBatcher.java:268)
... 9 more
这个问题和之前的一样,都是因为外键设置了非空导致,外键是可以为空的。
为什么呢?
我们不是通过外键关联来保存Product的么,先保存category,然后保存Product,我们在保存product的时候,没有设置主键值,而是从category的id获取,而此时的product 外键要求非空,因此报错。
mysql> alter table product
-> modify category_id int not null;
可以使用上面的语句来重现这个错误。