Servlet类:
package org.servlet;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.util.Iterator;
import java.util.List;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import sun.net.TelnetOutputStream;
import sun.net.ftp.FtpClient;
public class FtpServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private FtpClient ftpClient;
public FtpServlet() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.getProperties().put("file.encoding", "GBK");
response.setContentType("text/html;charset=UTF-8");
request.setCharacterEncoding("utf-8");
TelnetOutputStream os = null;
FileInputStream is = null;
try{
boolean flag = connectServer(ip, userName, password,savePath);//调用FTP连接方法
os = ftpClient.put(“abc.txt”); //"abc.txt"是用ftp上传后的新文件名
File file_in = new File(“c:/temp/abc.txt”); //要上传的文件
if (file_in.length()==0) {
return ;
}
is = new FileInputStream(file_in);
byte[] bytes = new byte[1024];
int c;
while ((c = is.read(bytes)) != -1) {
os.write(bytes, 0, c); //开始写
}
}catch (FileNotFoundException e1) {
e1.printStackTrace();
}catch (IOException e1) {
e1.printStackTrace();
} catch (FileUploadException e) {
e.printStackTrace();
}finally {
if (is != null) {
is.close();
}
if (os != null) {
os.close();
}
if (ftpClient != null) {
ftpClient.closeServer();
}
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request,response);
}
//连接ftp服务器
private boolean connectServer(String ip, String user, String password,String path) throws IOException {
// server:FTP服务器的IP地址;user:登录FTP服务器的用户名;password:登录FTP服务器的用户名的口令;path:FTP服务器上的 路径
try{
ftpClient = new FtpClient();
ftpClient.openServer(ip);
ftpClient.login(user, password);
if (path.length()!= 0){ //path是ftp服务下主目录的子目录
ftpClient.cd(path);
}
ftpClient.binary(); //用2进制上传
return true;
}catch(IOException e){
e.printStackTrace();
return false;
}
}
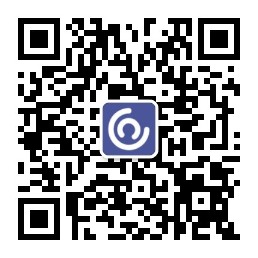
//FTP文件下载
public void download(){
connectServer(ip, userName, password,savePath);
String fileName = request.getParameter("filename");
try{
ftpIn = ftpClient.get(fileName); //fileName为FTP服务器上要下载的文件名
byte[] buf = new byte[204800];
int bufsize = 0;
ftpOut = new FileOutputStream(path+"/"+fileName); //存放在本地硬盘的物理位置
while ((bufsize = ftpIn.read(buf, 0, buf.length)) != -1) {
ftpOut.write(buf, 0, bufsize);
}
}catch(Exception e){
e.printStackTrace();
}finally{
if (ftpIn != null) {
ftpIn.close();
}
if (ftpOut != null) {
ftpOut.close();
}
if (ftpClient != null) {
ftpClient.closeServer();
}
}
}
}