导入numpy并查看版本
import numpy as np
np.__version__
什么是numpy?
Numpy即Numeric Python,python经过扩展可以支持数组和矩阵类型,并且具有大量的函数可以计算这些数组和矩阵。这些数组一般是多维的,而这个扩展的程序包就是numpy。
【注】是数据分析和机器学习中最基本的工具,后面许多API和工具都是建立在他得基础上,如:pandas、scipy、matplotlib等
一、创建ndarray
numpy中最基础数据结构就是ndarray:即数组
1. 使用np.array()由python list创建
data = [1,2,3]
nd = np.array(data)
nd
data
type(nd),type(data)
nd.dtype # 数组中的元素的类型
nd2 = np.array([1,2,3,4.5,True,"1234"])
nd2
nd2.dtype
注意:1、数组中所有的元素类型相同 2、如果通过列表来创建的时候,列表中元素不一样,会被统一成某种类型(优先级:str>float>int)
# 注意:图片也可在numpy里面用数组来表示
# 引入一张图片
import matplotlib.pyplot as plt # 这个是用于处理数据可视化的一个工具
girl = plt.imread("./source/girl.jpg")
girl # 彩色图片是一个三维的数组
girl.shape # 数据的形状
plt.imshow(girl)
plt.show()
boy = np.array([[0.21,0.14,0.12343,0.14],
[0.21,0.14,0.12343,0.14],
[0.21,0.14,0.12343,0.14],
])
plt.imshow(boy,cmap="gray")
plt.show()
g = girl[:200,:300]
plt.imshow(g)
plt.show()
2. 使用np的routines函数创建
1)np.ones(shape,dtype=None,order='C')
# 参数shape数据的形状传一个元组,元组的第0个元素代表第0维中的元素个数,依次类推
np.ones((3,2,4,5,6,7,8))
ones
ones = np.ones((168,59,3))
plt.imshow(ones)
plt.show()
ones[:,:,1:] = 0 # 数组是可以切片赋值的
ones[:,:,0] = 0.3
ones
plt.imshow(ones)
plt.show()
2)np.zeros(shape,dtype="float",order="C")
np.zeros((2,3),dtype="int32")
3)np.full(shape,fill_value,dtype=None)
np.full((3,4),12,dtype="float")
4)np.eye(N,M,k=0,dtype='float')
np.eye(4)
5)np.linspace(start,stop,num=50)
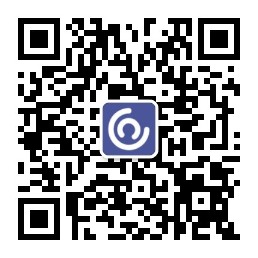
np.linspace(0,10,10)
np.logspace(1,100,10)
6)np.arange([start,]stop,[step,]dtype=None) "[]"中是可选项
np.arange(0,100,5)
7)np.random.randint(low,high=None,size=None,dtype='I')
np.random.randint(0,10,size=(10,5)) # low下限 high上限 size形状(元组)
8)np.random.randn(d0,d1,...,dn) 从第一维度到第n维度生成一个数组,数组中的数字符合标准正态分布
np.random.randn(5,6,10) # N(0,1)
9)np.random.normal(loc=0.0,scale=1.0,size=None)
np.random.normal(450,100,size=(90,10))
10)np.random.random(size=None)
np.random.random(size=10) # 生成0-1之间的浮点数
用随机数生成图片
boy = np.random.random((667,480,3))
plt.imshow(boy,cmap="gray")
plt.show()
二、ndarray的属性
数组的常用属性:
维度 ndim, 大小 size, 形状 shape, 元素类型 dtype, 每项大小 itemsize, 数据 data
tigger = plt.imread("./source/tigger.jpg")
tigger
tigger.ndim
girl.ndim
nd
nd.size
tigger.shape
nd.dtype
tigger.dtype
nd.itemsize
tigger.itemsize
nd.data
tigger.data
三、ndarray的基本操作
1、索引
l = [1,3,4,6,7,8,0]
l[-1]
nd = np.random.randint(0,4,size=4)
nd
nd[0]
lp = [[1,2,4],
[4,5,6]
]
lp
lp[0][1]
lp[0,1]
nd = np.random.randint(0,10,size=(6,6))
nd
nd[0]
nd[0][1]
nd[0,1] # 先访问第0个维度的第0个,再访问第1个维度中第1
nd[1,2,3] # 维度不足3个,不这样访问
nd[1,4] = 20000
nd
nd
nd[[1,1,1,1,1]]
nd[[1,4,5,2,0]] # 连续访问多个,可以把你要访问的那些下标写成一个列表
2、切片
nd = np.random.randint(0,10,size=(4,4))
nd
nd[0:5] # 区间左闭右开
l[0:10]
nd[1:]
nd[::-1]
nd
nd[:,0:2]
把girl调头
girl
girl2 = girl[::-3]
plt.imshow(girl2)
plt.show()
girl3 = girl[::2,::-2]
plt.imshow(girl3)
plt.show()
girl4 = girl[:,:,::1]
girl4[:,:,:2] = 0
plt.imshow(girl4)
plt.show()
3、变形
reshape()
resize()
tigger
tigger.shape
nd = np.random.randint(0,20,size=12)
nd (1*12)
nd.reshape((3,4))
nd
nd.reshape((4,4)) # 元素总数不一致,是不能相互转变的
nd.reshape((1,6))
nd.reshape((1,1,2,6))
nd.resize((3,4))
nd
【注意】
1、resize()和reshape()都是对数组进行变形,resize()是在原来数组上直接变形,reshape()生成一个新的数组
2、变形的时候,新数组的总元素个数要和原来的保持一致
拼图游戏
girl = plt.imread("./source/girl2.jpg")
plt.imshow(girl)
plt.show()
tigger[150:450,200:500] = girl
plt.imshow(tigger)
plt.show()
4、级联
nd1 = np.random.randint(0,100,size=(4,4))
nd2 = np.random.randint(0,100,size=(4,3))
print(nd1)
print(nd2)
级联:就是按照指定的维度把两个数组链接在一起
级联注意点:
1、参与级联的数组要构成一个列表(或元组)
2、维度必须一样
3、形状必须相符(如:二维数组axis=0时,列数必须一样,axis=1的时候行数必须一样)
4、级联的默认方向shape的第一个值所代表的那个维度(axis默认为0)
np.concatenate([nd1,nd2],axis=1)
# 参数一,参与级联的那些数组;参数二,级联的维度
nd3 = np.random.randint(0,100,size=(6,4))
nd3
np.concatenate([nd1,nd3])
hstack()和vstack()
hstack()把列数组改成一个行数组(把所有行上数组拼接成一个一行的数组)
vstack()把一个单行的数组拆成多行(每个行上只有一个数字)
nd3 = np.random.randint(0,10,size=(10,1))
nd3
np.hstack(nd3)
nd4 = np.random.randint(0,50,size=(4,3))
nd4
np.hstack(nd4)
np.vstack(nd4)
nd5 = np.random.randint(0,10,size=10)
nd5
np.vstack(nd5)
5、切分
split()
vsplit()
hsplit()
nd = np.random.randint(0,100,size=(5,6))
nd
np.hsplit(nd,[1,3]) # 代表在水平方向切分
# 参数一,被切的数组 参数二,切分点(列表,在切分点前切分)
np.vsplit(nd,[1,4]) # 纵向上切分 参数和横向类似
np.split(nd,[1,3],axis=0) # 切行
np.split(nd,(1,3),axis=1) # 切列
6、副本
nd = np.random.randint(0,100,size=6)
nd
nd1 = nd
nd1[0] = 1000
nd1
nd # 此时nd1和nd变量引用是同一个数组对象(nd和nd1中存储的地址都是一样的)
# 在某种意义上这也是一种浅拷贝
用copy()函数对数组对象创建副本
nd2 = nd.copy()
nd2
nd2[2] = 2000
nd2
nd
nd1
用列表创建数组的过程有木有创建副本
l = [1,2,3,4]
nd = np.array(l) # 把l拷贝了一份放在了数组nd中
nd[0] = 100
nd
l
四、ndarray的聚合操作
1、求和
nd = np.random.randint(0,10,size=(3,4))
nd
np.sum(nd,axis=0) # 把行按照列标对应加起来
np.sum(nd,axis=1) # 把列按照对应行标加起来
np.sum(nd) # 把所有的元素加起来
nd.sum()
2、最值
nd
np.max(nd)
np.max(nd,axis=0) # 求每个列里面最大值
np.max(nd,axis=1) # 求每一行里面的最大值
np.argmax(nd)
np.argmin(nd)
np.argmax(nd,axis=0) # 在列上求最大值并返回最大值的行标
np.argmin(nd,axis=1) # 在每个行上求最小值返回列标
3、其他聚合操作
Function Name NaN-safe Version Description
np.sum np.nansum Compute sum of elements
np.prod np.nanprod Compute product of elements
np.mean np.nanmean Compute mean of elements
np.std np.nanstd Compute standard deviation
np.var np.nanvar Compute variance
np.min np.nanmin Find minimum value
np.max np.nanmax Find maximum value
np.argmin np.nanargmin Find index of minimum value
np.argmax np.nanargmax Find index of maximum value
np.median np.nanmedian Compute median of elements
np.percentile np.nanpercentile Compute rank-based statistics of elements
np.any N/A Evaluate whether any elements are true
np.all N/A Evaluate whether all elements are true
np.power 幂运算
nd = np.array([1,2,3,np.nan])
nd
np.nan + 100
np.nan*100
# nan表示缺失(默认是浮点型),缺失和任何数做运算结果都是缺失
nd.sum()
np.nansum(nd) # 以nan开头的聚合方法,可在聚合运算的直接把nan剔除
思考题:给定一个4维矩阵,如何得到最后两维的和?
nd = np.random.randint(0,10,size=(2,3,2,2))
nd
nd.sum(axis=-1)
nd.sum(axis=-2)
nd.sum(axis=(-1,-2))
思考题:如何根据第3列来对一个5*5矩阵排序?
nd = np.random.randint(0,20,size=(5,5))
nd
nd[[3,0,4,1,2]] #??
nd
sort_ind = np.argsort(nd[:,3]) # 排序并且返回排序以后的下标序列
sort_ind
nd[sort_ind]
思路
五、ndarray的矩阵操作
1. 基本矩阵操作
1)算术运算(即加减乘除)
nd = np.random.randint(0,10,size=(5,5))
nd
nd + 3 # 加运算,启动广播机制把缺失的地方补全
nd*2 # 乘法不需要广播机制
nd/2
2)矩阵积
nd1 = np.random.randint(0,5,size=(4,3))
nd2 = np.random.randint(0,5,size=(3,4))
print(nd1)
print(nd2)
np.dot(nd1,nd2)
2. 广播机制
ndarray的广播机制的两条规则:
- 1、为缺失维度补1
- 2、假定缺失的元素用已有值填充
m = np.random.randint(0,10,size=(2,3))
m
a = np.arange(3)
a
# 求m+a
m+a
a = np.arange(3).reshape((3,1))
a
b = np.arange(3)
b
a+b
0 0 0 0 1 2
1 1 1 + 0 1 2
2 2 2 0 1 2
a + 3
a = np.random.randint(0,5,size=(3,3))
a
b = np.random.randint(0,5,size=(2,2))
b
a + b # 如果行和列个数均不相同无法进行补全,不能相加
排序
nd = np.random.randint(0,100,size=(10))
nd
nd.sort() # 改变原来的数组
nd
np.sort(nd)# 不会改变原来的数组,而是生成一个新的数组
nd
nd1 = np.random.randint(0,100,size=(4,4))
nd1
np.sort(nd1,axis=1) # axis=1排的是列标 axis=0排的是行标
np.sort(nd1,axis=0)
# argsort()排的是下标
np.argsort(nd1,axis=0)