1.NSArray中:
- (void)makeObjectsPerformSelector:(SEL)aSelector; - (void)makeObjectsPerformSelector:(SEL)aSelector withObject:(id)argument;
应用场景:在tableview中,在一个NSArray中保存了每个cell的数据下载处理对象,当数据量比较大的时候,程序可能会收到 memoryWarring,这时候,如果程序继续扩大内存使用,程序将会被操作系统“闪退”。因此在收到memoryWarring的时候需要终止或暂停所有数据下载处理对象的任务。可以使用如下代码片段:
- (void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // terminate all pending download connections NSArray *allDownloads = [self.imageDownloadsInProgress allValues]; [allDownloads makeObjectsPerformSelector:@selector(cancelDownload)]; }
2.NSOperationQueue线程池:
NSOperationQueue中添加isa NSOperation的类实例,简化了多线程程序的开发。NSOperation的子类只需要覆盖父类的main方法即可。
a.继承NSOperation,实现自己的线程类
//code
@interface MyTask: NSOperation @end @implementation MyTask -(void)main { //custom code } @end
b.将MyTask子类添加到operationQueue中
//code
扫描二维码关注公众号,回复:
759563 查看本文章
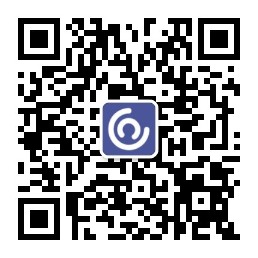
NSOperationQueue *queue = [[NSOperationQueue alloc] init]; int index = 0; MyTask *task1 = [[MyTask alloc] initWithTaskId:index++ andSleepTime:2.0f]; [queue addOperation:task1]; [task1 release]; MyTask *task2 = [[MyTask alloc] initWithTaskId:index++ andSleepTime:2.0f]; [queue addOperation:task2]; [task2 release]; [queue release];
c.如果某个线程执行的任务依赖于其它线程的执行结果的时候,可以自定义线程依赖关系
//code
NSOperationQueue *queue = [[NSOperationQueue alloc] init]; int index = 3; MyTask *task3 = [[MyTask alloc] initWithTaskId:index++ andSleepTime:3.0f] ; [queue addOperation:task3]; [task3 release]; MyTask *task4 = [[MyTask alloc] initWithTaskId:index++ andSleepTime:1.0f]; [task4 addDependency:[[queue operations] lastObject]]; [queue addOperation:task4]; [task4 release]; [queue release];
这样,虽然task3执行3s,task4执行1s,但是由于task4依赖于task3,因此task4在task3执行完成后才开始执行。一个 task可以设置多个这样的依赖关系。
3.去掉UISearchBar中的UISearchBarIconClear
//code
for (UIView *subview in searchBar.subviews) { if ([subview conformsToProtocol:@protocol(UITextInputTraits)]) { [(UITextField *)subview setClearButtonMode:UITextFieldViewModeNever]; } }
4.去掉UISearchDisplayController显示的“No Results”
//code
for( UIView *subview in self.searchDisplayController.searchResultsTableView.subviews ) { if ([subview isKindOfClass:[UILabel class]]) { UILabel *lbl = (UILabel*)subview; lbl.text = @""; } }