文章目录
(第二十一题)数组中出现次数超过一半的数字
2019年9月7日01:48:22
题目链接如下:
https://www.nowcoder.com/practice/e8a1b01a2df14cb2b228b30ee6a92163?tpId=13&tqId=11181&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间:2019年9月7日01:36:46
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日01:43:34
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
int MoreThanHalfNum_Solution(vector<int> numbers)
{
if (numbers.size() < 1)
throw"vector is empty!";
unordered_map<int, int>mymap;
for (int val : numbers)
{
mymap[val]++;
}
int size = numbers.size();
for (unordered_map<int, int>::iterator it = mymap.begin();
it != mymap.end(); ++it)
{
if (it->second > size / 2)
return it->first;
}
return 0;
}
};
int main()
{
Solution solution;
int Array[] = { 1,2,3,2,2,2,5,4,2 };
int Array1[] = { 1,2,3,4,5,8,5,4,2 };
vector<int>myvec(begin(Array), end(Array));
vector<int>myvec1(begin(Array1), end(Array1));
cout << solution.MoreThanHalfNum_Solution(myvec) << endl;
cout << solution.MoreThanHalfNum_Solution(myvec1) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日01:51:58
(第二十二题)最小的k个数
2019年9月7日02:09:50
题目链接如下:
https://www.nowcoder.com/practice/6a296eb82cf844ca8539b57c23e6e9bf?tpId=13&tqId=11182&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间:2019年9月7日01:59:11
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日02:03:47
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
vector<int> GetLeastNumbers_Solution(vector<int> input, int k)
{
vector<int>myvec;
int size = input.size();
if (size < 1 || k > size)
return myvec;
sort(input.begin(), input.end());
for (int i = 0; i < k; ++i)
{
myvec.push_back(input[i]);
}
return myvec;
}
};
int main()
{
Solution solution;
int Array[] = { 1,2,3,2,2,2,5,4,2 };
int Array1[] = { 4,5,1,6,2,7,3,8 };
vector<int>myvec(begin(Array), end(Array));
vector<int>myvec1(begin(Array1), end(Array1));
vector<int>result1 = solution.GetLeastNumbers_Solution(myvec, 8);
vector<int>result2 = solution.GetLeastNumbers_Solution(myvec1, 4);
for (int val : result1)
cout << val << " ";
cout << endl;
for (int val : result2)
cout << val << " ";
cout << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日02:12:08
注:实在是不能再写下去了,从昨天中午我10:30起床,11点开始学习。到现在只吃了一顿饭(半小时),其余时间全在刷 剑指offer,大概已经AC了22道题了。感觉很不错 但是 我的电脑已经跟了我7年时间了,一个老牌子的电脑(i3处理器),唉 对不起 我的电脑 跟着我宋某人 辛苦了。电脑 烫的现在都可以煎鸡蛋了,我去给它擦点水。
注:电脑发烫 360浏览器都不能正常工作了,哈哈哈哈哈 还好有个不常用的Chrome,睡了 每天再学吧!!!
(第二十三题)丑数
2019年9月7日10:59:42
题目链接如下:
https://www.nowcoder.com/practice/6aa9e04fc3794f68acf8778237ba065b?tpId=13&tqId=11186&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
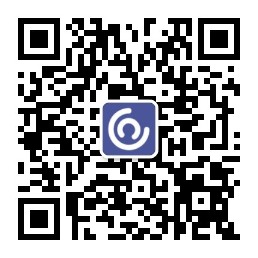
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日10:23:32
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日10:55:48
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
//首先除2,直到不能整除为止,然后除5到不能整除为止,然后除3直到不能整除为止。
//最终判断剩余的数字是否为1,如果是1则为丑数,否则不是丑数。
//也就是说 丑数=丑数*丑数
//第一个丑数 为1
//前20个丑数为:1, 2, 3, 4, 5, 6, 8, 9, 10, 12, 15, 16, 18, 20, 24, 25, 27, 30, 32, 36。
//于是判断第index个丑数 就是找上面的序列中的数字
/*
于是算法思想:每在myvec里面放入一个丑数,那么就又可以衍生出三个丑数
可以将以上序列(a1=1除外)可以分成3类,必定满足:
包含2的有序丑数序列:2*a1, 2*a2, 2*a3 .....
包含3的有序丑数序列:3*a1, 3*a2, 3*a3 .....
包含5的有序丑数序列:5*a1, 5*a2, 5*a3 .....
以上3个序列的个数总数和为n个,而且已知a1 = 1了,将以
上三个序列合并成一个有序序列即可。
程序中t2,t3,t5实际就是合并过程中三个序列中带排序的字段索引
核心在于根据已知求到结果,再把这个结果当成已知,求下一个丑数。直到目标结果出现
注:合并结果 要求:有序的序列
如:
a1=1,a2的来源就是2*a1 3*a1 5*a1中的最小值2
a2=2,a3的来源就是2*a2 3*a1 5*a1中的最小值3
a3=3,a4的来源就是2*a2 3*a2 5*a1中的最小值4
a4=4,a5的来源就是2*a3 3*a2 5*a1中的最小值5
等等,这最终就是一个有序序列
*/
class Solution {
public:
int GetUglyNumber_Solution(int index)
{
vector<int>myvec;
if (index < 7)
return index;
myvec.push_back(1);//1是第一个丑数
int indexof_2 = 0, indexof_3 = 0, indexof_5 = 0;
for (int i = 1; i < index; ++i)
{
myvec.push_back(min(2 * myvec[indexof_2],
min(3 * myvec[indexof_3], 5 * myvec[indexof_5])));
if (myvec[i] == 2 * myvec[indexof_2])
indexof_2++;
if (myvec[i] == 3 * myvec[indexof_3])
indexof_3++;
if (myvec[i] == 5 * myvec[indexof_5])
indexof_5++;
}
return myvec[index - 1];
}
};
int main()
{
Solution solution;
cout << "第20个丑数:" << solution.GetUglyNumber_Solution(20) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日11:02:04
(第二十四题)第一个只出现一次的字符
2019年9月7日11:40:02
题目链接如下:
https://www.nowcoder.com/practice/1c82e8cf713b4bbeb2a5b31cf5b0417c?tpId=13&tqId=11187&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日11:03:35
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日11:39:11
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
int FirstNotRepeatingChar(string str)
{
int size = str.size();
if (size == 0)
return -1;
unordered_map<char, int>mymap;
for (int i = 0; i < size; ++i)
{
mymap[str[i]]++;
}
#if 0
unordered_map<char, int>::iterator it = mymap.begin();
for (; it != mymap.end(); ++it)
{
if (it->second == 1)
break;
}
if (it == mymap.end())
return -1;
for (int i = 0; i < size; ++i)
{
if (str[i] == it->first)
return i;
}
#endif
for (int i = 0; i < size; ++i)
{
if (mymap[str[i]] == 1)
return i;
}
return -1;
}
};
int main()
{
Solution solution;
string mystr("bAbcddeaa");
string mystr1("gg");
cout << "第一个只出现一次的字符的位置:" << solution.FirstNotRepeatingChar(mystr) << endl;
cout << "第一个只出现一次的字符的位置:" << solution.FirstNotRepeatingChar(mystr1) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日11:41:47
这道本来6分钟就可以做出来的,偏偏花了36分钟。而且还是犯的还是特么昨天中午的那个错误。我在代码里面 将那块给注释起来了,大家也可以考虑一下 为什么不可以? 如果你不会 你好好的去面壁思过吧。
(第二十五题)数组中的逆序对
2019年9月7日15:19:38
注:写这个题,先去参考我的博客:
DSA之十大排序算法第五种:Merge Sort
题目链接如下:
https://www.nowcoder.com/practice/96bd6684e04a44eb80e6a68efc0ec6c5?tpId=13&tqId=11188&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日11:45:33
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间:
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
void devide_and_conquer(vector<int>&src,int low,int high,long *count_num)
{
if (low >= high)
return;
int mid = low + (high - low) / 2;
devide_and_conquer(src, low, mid, count_num);
devide_and_conquer(src, mid + 1, high, count_num);
int i = low, j = mid + 1, k = 0;//k是临时数组的下标
vector<int>temp_vec(high - low + 1);
while (i <= mid && j <= high)
{
if (src[i] > src[j])
{
//现在这个元素在j的位置上,它前面有mid+1-i个数 比它大
//在移动数据之后 当前的这个小数 的逆序就不存在了
//src[i]>a[j]了,那么这一次,
//从src[i]开始到src[mid]必定都是大于这个src[j]的,
//因为此时分治的两边已经是各自有序了
//这是最重要的一步:
*count_num = (mid - i + 1 + *count_num) % 1000000007;
temp_vec[k++] = src[j++];
}
else
{
temp_vec[k++] = src[i++];
}
}
while (i <= mid)
{
temp_vec[k++] = src[i++];
}
while (j <= high)
{
temp_vec[k++] = src[j++];
}
//接下来就是把排序过的数据 拷贝回去
k = 0;
for (int i=low;i<=high;++i)
{
src[i] = temp_vec[k++];
}
}
int InversePairs(vector<int> &data)
{
int size = data.size();
if (size <= 1)
return 0;
long count_num = 0;
devide_and_conquer(data, 0, size - 1, &count_num);
return (int)count_num % 1000000007;
}
};
int main()
{
Solution solution;
int Array[] = { 1,2,3,4,5,6,7,0 };
//int Array1[] = { 1,2,3,4,5,6,7,8 };
int Array1[] = { 4,5,2,1 };
vector<int>myvec(begin(Array), end(Array));
vector<int>myvec1(begin(Array1), end(Array1));
cout << "逆序对个数:" << solution.InversePairs(myvec) << endl;
cout << "逆序对个数:" << solution.InversePairs(myvec1) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日15:23:07
(第二十六题)数字在排序数组中出现的次数
2019年9月7日15:30:56
题目链接如下:
https://www.nowcoder.com/practice/70610bf967994b22bb1c26f9ae901fa2?tpId=13&tqId=11190&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日15:26:06
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日15:29:20
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
int GetNumberOfK(vector<int> &data, int k)
{
int size = data.size();
if (size == 0)
return 0;
unordered_map<int, int>mymap;
for (int val : data)
{
mymap[val]++;
}
auto it = mymap.find(k);
if (it != mymap.end())
{
return it->second;
}
else
return 0;
}
};
int main()
{
Solution solution;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日15:32:38
(第二十七题)两个链表的第一个公共节点
2019年9月7日16:34:52
题目链接如下:
https://www.nowcoder.com/practice/6ab1d9a29e88450685099d45c9e31e46?tpId=13&tqId=11189&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日15:32:38
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日16:33:43
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <unordered_map>
#include <time.h>
#include <algorithm>
using namespace std;
struct ListNode {
int val;
struct ListNode *next;
ListNode(int x) :val(x), next(nullptr) {}
};
class Solution {
public:
/*
公共的节点,说明公共节点的值和next都是一样的,所以一定会有公共的尾部。
意思就是:让长的先走两个链表的长度差,然后再一起走。
*/
ListNode* FindFirstCommonNode(ListNode* pHead1, ListNode* pHead2)
{
if (pHead1 == nullptr || pHead2 == nullptr)
return nullptr;
ListNode* this_first_p = nullptr;
vector<int>myvec1; ListNode* p1 = pHead1;
vector<int>myvec2; ListNode* p2 = pHead2;
while (p1 != nullptr)
{
myvec1.push_back(p1->val);
p1 = p1->next;
}
while (p2 != nullptr)
{
myvec2.push_back(p2->val);
p2 = p2->next;
}
int size1 = myvec1.size();
int size2 = myvec2.size();
p1 = pHead1;
p2 = pHead2;
if (size1 <= size2)
{
int i = 0;
for (; i < size2 - size1; ++i)//先走几步
{
p2 = p2->next;
}
for (; i < size2; ++i)
{
if (p1->val == p2->val && p1->next == p2->next)
{
return p1;
}
p1 = p1->next;
p2 = p2->next;
}
return nullptr;
}
else
{
int i = 0;
for (; i < size1 - size2; ++i)//先走几步
{
p1 = p1->next;
}
for (; i < size1; ++i)
{
if (p1->val == p2->val && p1->next == p2->next)
{
return p1;
}
p1 = p1->next;
p2 = p2->next;
}
return nullptr;
}
}
};
int main()
{
Solution solution;
int Array1[] = { 8,7,3,5,6,5,4,3,2 };
int Array2[] = { 7,5,4,3,2 };
ListNode* phead1 = new ListNode(8);//把长链做好
ListNode* cur_p = phead1;
for (int i = 1; i < 9; ++i)
{
ListNode* p = new ListNode(Array1[i]);
cur_p->next = p;
cur_p = cur_p->next;
}
ListNode* phead2 = new ListNode(7);//做短链
cur_p = phead1;
for (int i = 0; i < 5; ++i)
{
cur_p = cur_p->next;
}
phead2->next = cur_p;
cur_p = solution.FindFirstCommonNode(phead1, phead2);
if (cur_p != nullptr)
{
cout << "两个链表的公共节点的val:" << cur_p->val << endl;
}
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日16:37:22
(第二十八题)把数组排成最小的数
2019年9月7日17:09:49
题目链接如下:
https://www.nowcoder.com/practice/8fecd3f8ba334add803bf2a06af1b993?tpId=13&tqId=11185&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日16:40:06
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日17:05:09
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <set>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
/*
1.使用to_string() 可以将int 转化为string
2.给sort提供自定义的 比较方式
*/
//自定义compare_of_2int函数,将vector的数据排序
static bool compare_of_2int(int a, int b)
{
string str_a = to_string(a);
string str_b = to_string(b);
return (str_a + str_b) < (str_b + str_a);
}
string PrintMinNumber(vector<int> numbers)
{
string result;
int size = numbers.size();
if (size < 1)
{
return result;
}
//将vector里面的数据按照上面的方式排序
sort(numbers.begin(), numbers.end(), compare_of_2int);
for (int val : numbers)
{
result += to_string(val);
}
return result;
}
};
int main()
{
Solution solution;
int Array1[] = { 3,32,321 };
vector<int>myvec(begin(Array1), end(Array1));
cout << solution.PrintMinNumber(myvec) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日17:12:27
(第二十九题)整数中1出现的次数
2019年9月7日17:09:49
题目链接如下:
https://www.nowcoder.com/practice/bd7f978302044eee894445e244c7eee6?tpId=13&tqId=11184&tPage=2&rp=2&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日17:12:27
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日17:21:33
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <set>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
int NumberOf1Between1AndN_Solution(int n)
{
if (n < 1)
return 0;
int count = 0;
for (int i = 1; i <= n; ++i)
{
string str_i = to_string(i);
for (char c : str_i)
{
if (c == '1')
count++;
}
}
return count;
}
};
int main()
{
Solution solution;
cout << "1到n里面总共有:"
<< solution.NumberOf1Between1AndN_Solution(13) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日17:24:41
(第三十题)求1+2+3±—+n(有限制)
2019年9月7日17:55:14
题目链接如下:
https://www.nowcoder.com/practice/7a0da8fc483247ff8800059e12d7caf1?tpId=13&tqId=11200&tPage=3&rp=3&ru=/ta/coding-interviews&qru=/ta/coding-interviews/question-ranking
题目描述:
代码如下:
/**══════════════════════════════════╗
*作 者:songjinzhou ║
*CSND地址:https://blog.csdn.net/weixin_43949535 ║
**GitHub:https://github.com/TsinghuaLucky912/My_own_C-_study_and_blog║
*═══════════════════════════════════╣
*创建时间: 2019年9月7日17:30:59
*功能描述:
*
*
*═══════════════════════════════════╣
*结束时间: 2019年9月7日17:56:31
*═══════════════════════════════════╝
// .-~~~~~~~~~-._ _.-~~~~~~~~~-.
// __.' ~. .~ `.__
// .'// 西南\./联大 \\`.
// .'// | \\`.
// .'// .-~"""""""~~~~-._ | _,-~~~~"""""""~-. \\`.
// .'//.-" `-. | .-' "-.\\`.
// .'//______.============-.. \ | / ..-============.______\\`.
//.'______________________________\|/______________________________`.
*/
#include <iostream>
#include <vector>
#include <string>
#include <set>
#include <time.h>
#include <algorithm>
using namespace std;
class Solution {
public:
int Sum_Solution(int n)
{
int result = n;
//只能去使用 短路计算以退出递归
//但是缺点很明显:递归的层数不能太深 得小于3000
n && (result += Sum_Solution(n - 1));
return result;
}
};
/*
n=5 result=result+sun(4);-------5+10
n=4 result=result+sun(3);-------4+6
n=3 result=result+sun(2);-------3+3
n=2 result=result+sun(1);-------2+1
n=1 result=result+sun(0); -----1+0
n=0 result=result+sun(-1);----->0
*/
int main()
{
Solution solution;
cout << solution.Sum_Solution(5) << endl;
return 0;
}
/**
*备用注释:
*
*
*
*/
2019年9月7日17:57:19