版权声明:本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。
文件上传:就是将文件提交到服务器
文件上传:
浏览器端的要求:
1.表单的提交方法必须是post
2.必须有一个文件上传组件 <input type="file" name="">
3.必须设置表单的enctype=multipart/form-data
服务器端接收的要求:
servlet3.0中
1.需要在Servlet中添加注解
@multipartConfig (用来支持文件上传)
2.接收普通上传组件(除了文件上传组件):request.getParameter()
3.接收文件上传组件:request.getPart(name属性的值);
扫描二维码关注公众号,回复:
7659679 查看本文章
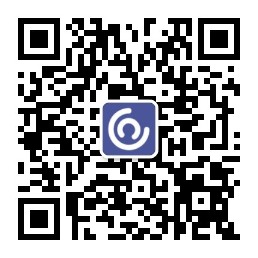
getName():获取的是name属性的值
4.获取文件名:
part.getHeader("content-disposition"):获取头信息 然后截取
文件上传应该注意的问题
名字重复的时候会覆盖,需要添加随机名称
在数据库中提供两个字段,
一个字段用来存放文件的真是名称
另一个字段用来存放 文件存放的路径
随机名称:
uuid
时间戳
文件安全:
重要的文件放在 web-inf 或者 meta-inf 或者 服务器创建一个路径
不是很重要的文件 放在项目下
文件存放目录
方式1:按照日期
方式2:按照用户,给每个用户创建一个文件夹来存放东西
方式3:按照文件个数
方式4:随机目录
代码:
浏览器端:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<form action="/webDay1701/upload2" method="post" enctype="multipart/form-data">
用户名:<input name = "username"><br>
文件:<input type="file" name="f"><br>
<input type="submit">
</form>
</body>
</html>
生成随机名称的工具类
package com.itheima.utils;
import java.util.UUID;
public class UploadUtils {
/**
* 获取随机名称
* @param realName 真实名称
* @return uuid
*/
public static String getUUIDName(String realName){
//realname 可能是 1.jpg 也可能是 1
//获取后缀名
int index = realName.lastIndexOf(".");
if(index==-1){
return UUID.randomUUID().toString().replace("-", "").toUpperCase();
}else{
return UUID.randomUUID().toString().replace("-", "").toUpperCase()+realName.substring(index);
}
//return null;
}
/**
* 获取文件真实名称
* @param name
* @return
*/
public static String getRealName(String name){
// c:/upload/1.jpg 1.jpg
//获取最后一个"/"
int index = name.lastIndexOf("\\");
return name.substring(index+1);
}
/**
* 获取文件目录
* @param name 文件名称
* @return 目录
*/
public static String getDir(String name){
int i = name.hashCode();
String hex = Integer.toHexString(i);
int j=hex.length();
for(int k=0;k<8-j;k++){
hex="0"+hex;
}
// System.out.println(hex);
return "/"+hex.charAt(0)+"/"+hex.charAt(1);
}
public static void main(String[] args) {
String s="0";
String s1="1.jpg";
//System.out.println(getUUIDName(s));
//System.out.println(getUUIDName(s1));
//System.out.println(getRealName(s));
//System.out.println(getRealName(s1));
System.out.println(getDir(s));
System.out.println(getDir(s1));
//getDir(s);
//getDir(s1);
}
}
servlet实现
package com.itheima.web.servlet;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
import org.apache.commons.io.IOUtils;
import com.itheima.utils.UploadUtils;
@WebServlet("/upload2")
@MultipartConfig
public class Upload2Servlet extends HttpServlet {
private static final long serialVersionUID = 1L;
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//0.设置编码
request.setCharacterEncoding("utf-8");
//获取普通的上传组件 username
String username = request.getParameter("username");
System.out.println(username);
//获取文件上传组件
Part part = request.getPart("f");
//获取文件名称
String sss = part.getHeader("content-dispositon");
String realName = sss.substring(sss.indexOf("filename=")+10, sss.length()-1);
System.out.println("文件的名称"+realName);
//获取随机名称
String uuidName = UploadUtils.getUUIDName(realName);
System.out.println("文件随机名称"+uuidName);
//获取文件存放的目录
String dir = UploadUtils.getDir(uuidName);
String realPath = this.getServletContext().getRealPath("/upload"+dir);
File file = new File(realPath);
if(!file.exists())
{
file.mkdirs();
}
System.out.println("文件的目录"+realPath);
//获取文件 对拷流
InputStream is = part.getInputStream();
FileOutputStream os = new FileOutputStream(new File(file,uuidName));
IOUtils.copy(is,os);
is.close();
os.close();
//删除临时文件
part.delete();
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}