这是第二次实操viper了,
年纪大了就多练练,才能记住。
http://go.coder55.com/article/6589
https://github.com/spf13/viper
package main import ( "github.com/spf13/pflag" "github.com/spf13/viper" "golang.org/x/net/context" "github.com/fsnotify/fsnotify" "fmt" ) func main() { //读取Command Line参数 pflag.String("hostAddress", "127.0.0.1", "Server running address") pflag.Int64("port", 8080, "Server running port") pflag.Parse() viper.BindPFlags(pflag.CommandLine) fmt.Printf("hostAddress: %s, port: %s \n", viper.GetString("hostAddress"), viper.GetString("port"), ) //读取yaml文件 v := viper.New() v.SetConfigName("linux_config") v.AddConfigPath("./") v.SetConfigType("yaml") if err := v.ReadInConfig(); err != nil { fmt.Printf("err: %s\n", err) } fmt.Printf(`TimeStamp:%s \n CompanyInfomation.Name:%s \n CompanyInfomation.Department:%s \n`, v.Get("TimeStamp"), v.Get("CompanyInfomation.Name"), v.Get("CompanyInfomation.Department"), ) //监听函数,可以免重启修改配置参数 ctx, _ := context.WithCancel(context.Background()) v.OnConfigChange(func(e fsnotify.Event) { fmt.Printf("config is change: %s\n", e.String()) fmt.Printf(`TimeStamp:%s \n CompanyInfomation.Name:%s \n CompanyInfomation.Department:%s \n`, v.Get("TimeStamp"), v.Get("CompanyInfomation.Name"), v.Get("CompanyInfomation.Department"), ) }) v.WatchConfig() <-ctx.Done() //反序列化 parseYaml(v) } type CompanyInfomation struct { Name string MarketCapitalization int64 EmployeeNum int64 Department []interface{} IsOpen bool } type YamlSetting struct { TimeStamp string Address string Postcode int64 CompanyInfomation CompanyInfomation } func parseYaml(v *viper.Viper) { var yamlObj YamlSetting if err := v.Unmarshal(&yamlObj); err != nil { fmt.Printf("err: %s\n", err) } fmt.Println(yamlObj) }
输出的样子
package main import ( "flag" "github.com/spf13/pflag" ) func main() { // using standard library "flag" package flag.Int("flagname", 1234, "help message for flagname") pflag.CommandLine.AddGoFlagSet(flag.CommandLine) pflag.Parse() viper.BindPFlags(pflag.CommandLine) i := viper.GetInt("flagname") // retrieve value from viper ... }
在Viper中使用pflag并不排除使用 标准库中使用标志包的其他包。pflag包可以通过导入这些标志来处理为标志包定义的标志。这是通过调用名为AddGoFlagSet()的pflag包提供的便利函数来实现的。
扫描二维码关注公众号,回复:
7699275 查看本文章
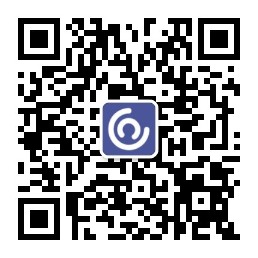