stream对象
Stream
IntStream
LongStream
DoubleStream
创建
常用的三种方式:
- 使用list对象:
- list.stream() − 为集合创建串行流。
- list.parallelStream() − 为集合创建并行流。
- Arrays: Arrays.stream( T[] array) − 为数组创建流(可以创建IntStream,LongStream,DoubleStrem)。
- Stream: Stream.of(T... values) − 为一组同类型的数据创建流。
demo:
/**
* 集合接口有两个方法来生成流:
* 按照流的类型可分为串行流和并行流
* stream() − 为集合创建串行流。
* parallelStream() − 为集合创建并行流。
*/
private static Stream<String> createStreamFromCollection() {
List<String> list = Arrays.asList("abc", "", "bc", "efg", "abcd","", "jkl");
return list.stream();
}
/**
* 使用Stream.of()创建流
* @return
*/
private static Stream<String> createStreamFromValues() {
return Stream.of("hello", "alex", "wangwenjun", "world", "stream");
}
/**
* 使用Arrays.stream()创建流
* 可以生成IntStream,LongStream,DoubleStream
* @return
*/
private static Stream<String> createStreamFromArrays() {
String[] strings = {"hello", "alex", "wangwenjun", "world", "stream"};
return Arrays.stream(strings);
}
操作
filter : 过滤, 过滤掉不符合条件的数据。
map :转换流类型, 返回一个流,该流包含将给定函数应用于该流元素的结果。
比如一个Stream
limit : 分页。
扫描二维码关注公众号,回复:
8093875 查看本文章
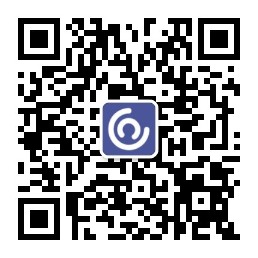
collect : 将流收集成一个数组。
List<Dish> menu = Arrays.asList(
new Dish("pork", false, 800, "肉类"),
new Dish("beef", false, 700, "肉类"),
new Dish("chicken", false, 400, "肉类"),
new Dish("french fries", true, 530, "鱼肉类"),
new Dish("rice", true, 350, "鱼肉类"),
new Dish("season fruit", true, 120, "鱼肉类"),
new Dish("pizza", true, 550, "鱼肉类"),
new Dish("prawns", false, 300, "其他"),
new Dish("salmon", false, 450, "其他"));
//创建菜单流
List<Map<String,Integer>> result = menu.stream().filter(d -> {
// 过滤出卡路里大于300的值
System.out.println("filtering->" + d.getName());
return d.getCalories() > 300;
})
.map(d -> {
Map<String, Integer> map = new HashMap<>();
map.put(d.getName(),d.getCalories());
return map;
})
.limit(3)
.collect(toList());
System.out.println(result);
其他操作
List<Integer> list = Arrays.asList(1, 2, 3, 4, 5, 6, 6, 7, 7, 1);
List<Integer> result = list.stream().filter(i -> i % 2 == 0).collect(toList());
System.out.println("i -> i % 2 的值 : "+result);
result = list.stream().distinct().collect(toList());
System.out.println("去重 : "+result);
result = list.stream().skip(2).collect(toList());//跳过前两个数
System.out.println("跳过 : "+result);
result = list.stream().limit(4).collect(toList());//取前4个值
System.out.println("分页 : "+result);
System.out.println("循环方式一 : ");
list.forEach(i -> System.out.println(i));
System.out.println("循环方式二 : ");
list.forEach((Integer i) -> System.out.println(i));
System.out.println("循环方式三 : ");
list.forEach(System.out::println);
for (int i : list) {
System.out.println(i);
}
**** 码字不易如果对你有帮助请给个关注****
**** 爱技术爱生活 QQ群: 894109590****