老套路先看效果图:
一个特别简单的字母排序列表如上图:
先看下有哪些属性:
<com.xiayiye.honorfirst.custom.CustomNumView
android:id="@+id/cn_view"
android:layout_width="40dp"
android:layout_height="match_parent"
android:layout_alignParentRight="true"
android:background="@android:color/holo_blue_bright"
app:num_text_center="false"
app:num_text_color="@android:color/white"
app:num_text_left="true"
app:num_text_right="true"
app:num_text_size="20sp" />
非常简单,就加了五个自定义属性分别是:文字字母居中,居左,居右,文字字母大小,颜色等。如何自定义的呢?
1.我们需要在value文件夹下面新建一个attrs文件,然后定义一些属性如下:
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="CustomNumView">
<attr name="num_text_color" format="reference|color" />
<attr name="num_text_size" format="dimension" />
<attr name="num_text_left" format="boolean" />
<attr name="num_text_right" format="boolean" />
<attr name="num_text_center" format="boolean" />
</declare-styleable>
</resources>
然后写个类继承view。实现相关的三四个构造方法。字母是通过onDraw方法画上去的,具体计算步骤就不讲解了
然后通过TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.CustomNumView);拿到自定义的xml文件里面的自己定义的相关属性
textColor = typedArray.getColor(R.styleable.CustomNumView_num_text_color, Color.WHITE);
float dimension = typedArray.getDimension(R.styleable.CustomNumView_num_text_size, 10);
isLeft = typedArray.getBoolean(R.styleable.CustomNumView_num_text_left, false);
isCenter = typedArray.getBoolean(R.styleable.CustomNumView_num_text_center, false);
isRight = typedArray.getBoolean(R.styleable.CustomNumView_num_text_right, false);
typedArray.recycle();
上面就是拿到的自定义属性的五个属性方法
然后根据拿到的方法进行相应的逻辑判断处理后在xml布局中就可以使用了
扫描二维码关注公众号,回复:
8510666 查看本文章
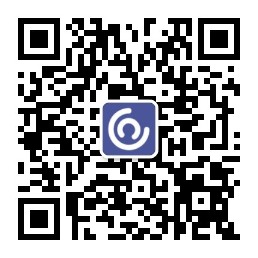
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
for (int i = 0; i < data.length; i++) {
String str = data[i];
float textWidth = paint.measureText(str);
float textHeight = bounds.height();
// float x = geZiWidth * 0.5f - textWidth * 0.5f;
float y = (geZiHeight * 0.5f + textHeight * 0.5f) + i * geZiHeight;
float x = 0;
if (isCenter) {
//xml中定义了居中就居中显示
x = geZiWidth * 0.5f - textWidth * 0.5f;
} else if (isLeft) {
//xml中定义了左边就左边显示
x = 0;
} else if (isRight) {
//xml中定义了右边就右边显示
x = geZiWidth - textWidth;
} else {
//默认居中
x = geZiWidth * 0.5f - textWidth * 0.5f;
}
paint.getTextBounds(str, 0, str.length(), bounds);
paint.setColor(selectNum == i ? Color.RED : textColor);
canvas.drawText(str, x, y, paint);
// canvas.drawText(str, geZiWidth * 0.5f - paint.measureText(str) * 0.5f, getHeight()/26*(i+1), paint);
}
}
咱们来看下最终的效果图:
源码已上传GitHub:点击浏览
字母列表view源码页面:点击浏览