using System;
using System.Threading;
using System.Threading.Tasks;
namespace AsyncAwaitDemo
{
public delegate Task MyDelegate();
class Program
{
static async void Call()
{
int x = await Task.Run<int>(() => { Thread.Sleep(3500); return 100; });
Console.WriteLine("From Call():" + x);
}
static void Main(string[] args)
{
Call();
LongCallWithCallback((i) =>
{
Console.WriteLine("Execution done." + i);
});
CallAsyncTask(async () =>
{
int x = 0;
await Task.Run(() =>
{
Thread.Sleep(3000);
x = 100;
});
Console.WriteLine("Got value:" + x);
});
while (true)
{
Console.WriteLine("Main thread working...");
Thread.Sleep(500);
}
}
private static void CallAsyncTask(MyDelegate myDelegate)
{
myDelegate();
}
private static MyDelegate GetATask()
{
return LongCallToReturn;
}
public static Task<int> LongCallToReturn()
{
return Task.Run<int>(() =>
{
Thread.Sleep(3000);
return 100;
});
}
public static async void LongCallWithCallback(Action<int> afterExecution)
{
int x = await Task.Run<int>(() =>
{
Thread.Sleep(5000);
return 100;
});
// Call back.
afterExecution(x);
}
}
}
using System;
using System.Threading;
using System.Threading.Tasks;
namespace Async2Await
{
class Program
{
static void Main(string[] args)
{
ProcessAsync();
while (true)
{
Thread.Sleep(1000);
Console.WriteLine("Main working...");
}
}
private static async void ProcessAsync()
{
await Task.Run(() =>
{
Thread.Sleep(5000);
Console.WriteLine("p1...");
});
await Task.Run(() =>
{
Thread.Sleep(5000);
Console.WriteLine("p2...");
});
Console.WriteLine("End of ProcessAsync()");
}
}
}
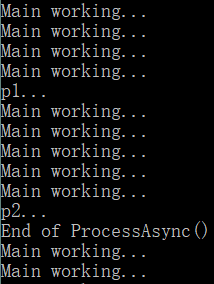