1、基于日期时间的断言工厂
基于日期时间的断言工厂主要是通过日期时间对请求进行断言,判断请求时间是否符合配置的时间,实现类主要有三种,分别如下:
AfterRoutePredicateFactory
:接收一个日期参数判断请求时间是否在配置时间之后;BeforeRoutePredicateFactory
:接收一个日期参数,判断请求日期是否在指定日期之前;BetweenRoutePredicateFactory
:接收两个日期参数,判断请求日期是否在这两个指定日期之间;
2、AfterRoutePredicateFactory
org.springframework.cloud.gateway.handler.predicate.AfterRoutePredicateFactory
,用于匹配请求时间在指定时间之后的请求,其UML关系图如下(其他实现类的UML类似):
其源码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
public class AfterRoutePredicateFactory extends AbstractRoutePredicateFactory<AfterRoutePredicateFactory.Config> { /** * DateTime key. */ public static final String DATETIME_KEY = "datetime"; public AfterRoutePredicateFactory() { super(Config.class); } @Override public List<String> shortcutFieldOrder() { return Collections.singletonList(DATETIME_KEY); } @Override public Predicate<ServerWebExchange> apply(Config config) { // 获取配置的时间 ZonedDateTime datetime = config.getDatetime(); return exchange -> { // 当前请求时间 final ZonedDateTime now = ZonedDateTime.now(); // 判断当前请求时间是否在配置时间之后 return now.isAfter(datetime); }; } public static class Config { @NotNull private ZonedDateTime datetime; public ZonedDateTime getDatetime() { return datetime; } public void setDatetime(ZonedDateTime datetime) { this.datetime = datetime; } } } |
2.1 yml配置示例
application.yml
1 2 3 4 5 6 7 8 |
spring: cloud: gateway: routes: - id: after_route uri: http://example.org predicates: - After=2019-01-20T17:42:47.789-07:00[America/Denver] |
3、BeforeRoutePredicateFactory
org.springframework.cloud.gateway.handler.predicate.BeforeRoutePredicateFactory
,用于匹配请求时间在指定时间之前的请求,其源码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
public class BeforeRoutePredicateFactory extends AbstractRoutePredicateFactory<BeforeRoutePredicateFactory.Config> { /** * DateTime key. */ public static final String DATETIME_KEY = "datetime"; public BeforeRoutePredicateFactory() { super(Config.class); } @Override public List<String> shortcutFieldOrder() { return Collections.singletonList(DATETIME_KEY); } @Override public Predicate<ServerWebExchange> apply(Config config) { // 获取配置时间 ZonedDateTime datetime = config.getDatetime(); return exchange -> { // 当前请求时间 final ZonedDateTime now = ZonedDateTime.now(); // 判断当前请求时间是否在配置时间之前 return now.isBefore(datetime); }; } public static class Config { private ZonedDateTime datetime; public ZonedDateTime getDatetime() { return datetime; } public void setDatetime(ZonedDateTime datetime) { this.datetime = datetime; } } } |
3.1 yml配置示例
application.yml
1 2 3 4 5 6 7 8 |
spring: cloud: gateway: routes: - id: before_route uri: http://example.org predicates: - Before=2019-01-20T17:42:47.789-07:00[America/Denver] |
4、BetweenRoutePredicateFactory
org.springframework.cloud.gateway.handler.predicate.BetweenRoutePredicateFactory
,用于匹配请求时间在指定时间之间的请求,其源码如下:
扫描二维码关注公众号,回复:
8590659 查看本文章
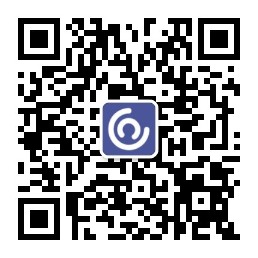
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 |
public class BetweenRoutePredicateFactory extends AbstractRoutePredicateFactory<BetweenRoutePredicateFactory.Config> { /** * DateTime 1 key. 开始时间 */ public static final String DATETIME1_KEY = "datetime1"; /** * DateTime 2 key. 结束时间 */ public static final String DATETIME2_KEY = "datetime2"; public BetweenRoutePredicateFactory() { super(Config.class); } @Override public List<String> shortcutFieldOrder() { return Arrays.asList(DATETIME1_KEY, DATETIME2_KEY); } @Override public Predicate<ServerWebExchange> apply(Config config) { // 获取配置的开始时间 ZonedDateTime datetime1 = config.datetime1; // 获取配置的结束时间 ZonedDateTime datetime2 = config.datetime2; // 断言结束时间大于开始时间 Assert.isTrue(datetime1.isBefore(datetime2), config.datetime1 + " must be before " + config.datetime2); return exchange -> { // 当前请求时间 final ZonedDateTime now = ZonedDateTime.now(); // 判断当前请求时间大于开始时间,并且小于结束时间 return now.isAfter(datetime1) && now.isBefore(datetime2); }; } @Validated public static class Config { @NotNull private ZonedDateTime datetime1; @NotNull private ZonedDateTime datetime2; public ZonedDateTime getDatetime1() { return datetime1; } public Config setDatetime1(ZonedDateTime datetime1) { this.datetime1 = datetime1; return this; } public ZonedDateTime getDatetime2() { return datetime2; } public Config setDatetime2(ZonedDateTime datetime2) { this.datetime2 = datetime2; return this; } } } |
4.1 yml配置示例
application.yml
1 2 3 4 5 6 7 8 |
spring: cloud: gateway: routes: - id: between_route uri: http://example.org predicates: - Between=2019-01-20T17:42:47.789-07:00[America/Denver], 2019-01-21T17:42:47.789-07:00[America/Denver] |