主要方面
创建jdbc步骤
- 加载驱动Class.forname()
- 获得连接Connection
- 获得Statement(PreparedStatement)对象,执行SQL语句
- 返回ResultSet结果,封装实体对象
- 关闭结果
Statement和PreparedStatement区别
- PreparedStatement可以防止参数注入,Statement不能防止
- PreparedStatement对象防止sql注入的方式是把用户非法输入的单引号用\反斜杠做了转义,从而达到了防止sql注入的目的
测试代码
package com;
import java.sql.*;
public class App
{
public static void main( String[] args ) throws Exception{
query_Statement();
query_PreparedStatement();
}
public static void query_Statement() throws Exception{
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/study?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8&allowMultiQueries=true&useSSL=false", "root", "123456");
Statement statement = connection.createStatement();
String sql = "select * from test_jbdc where id =1";
ResultSet rs = statement.executeQuery(sql);
while (rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
String address = rs.getString("address");
int age = rs.getInt("age");
System.out.println("id:"+id);
System.out.println("name:"+name);
System.out.println("address:"+address);
System.out.println("age:"+age);
}
rs.close();
statement.close();
connection.close();
}
public static void query_PreparedStatement() throws Exception{
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection("jdbc:mysql://127.0.0.1:3306/study?serverTimezone=GMT%2B8&useUnicode=true&characterEncoding=utf-8&allowMultiQueries=true&useSSL=false", "root", "123456");
String sql = "select * from test_jbdc where id =?";
PreparedStatement psmt = connection.prepareStatement(sql);
psmt.setInt(1,1);
ResultSet rs = psmt.executeQuery();
while (rs.next()){
int id = rs.getInt("id");
String name = rs.getString("name");
String address = rs.getString("address");
int age = rs.getInt("age");
System.out.println("id:"+id);
System.out.println("name:"+name);
System.out.println("address:"+address);
System.out.println("age:"+age);
}
rs.close();
psmt.close();
connection.close();
}
}
测试结果
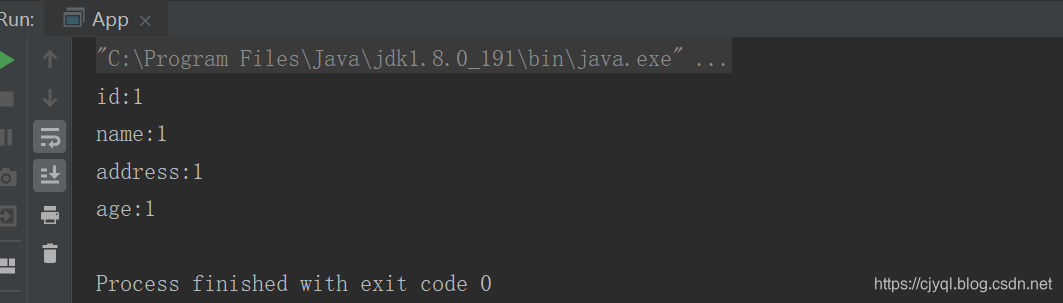