springmvc的使用
package org.base.controller;
import org.base.pojo.Department;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class DeptController {
/**
* 映射的url http://localhost:端口号/项目名/test.do
* 跳转到 (prefix)test.jsp(suffix)页面
*/
@RequestMapping("/test")
public String test(){
System.out.println("test");
return "test";
}
/**
* 如果方法无返回值,则跳转到 testP.jsp页面,即映射名
* 对于方法中的参数,可以对应url中的参数自动赋值
* 这里注意,如果url中的参数和方法定义的不一致,例如,此处的age=1.5,那么会报400错误
*/
@RequestMapping("/testP")
public void testParam(String name,Integer age){
System.out.println("name:"+name+",age:"+age);
}
/**
* 也可以显式声明url参数
*/
@RequestMapping("/testP1")
public void testParam1(@RequestParam("name")String name,Integer age){
System.out.println("name:"+name+",age:"+age);
}
/**
* 自动注入bean属性,注意,url中形式是 departmentId=12,不是 对象.属性 形式
*/
@RequestMapping("/testP2")
public void testParam2(Department dept){
System.out.println("dept:"+dept);
}
/**
* ResponseBody注解:此方法返回值直接写入 response的body中,默认是json
* 注意要导入 jackson.jar
*/
@RequestMapping("/test2")
@ResponseBody
public Department test2(){
Department dept = new Department(12, "abc", 31, 5);
return dept;
}
//@RequestMapping 注解的方法支持很多参数: HttpServletRequest/Response,HttpSession,Writer
// 不支持对象.属性传值方法,如果重名,/**
* restful 风格的传参方式
*/
@RequestMapping("/test3/{str}")
public void test3(@PathVariable("str")String str){
System.out.println("str:"+str);
}
/**
* date 日期类型的注入
*/
@RequestMapping("/test4")
public void test4(@DateTimeFormat(iso=ISO.DATE)Date d){
if(d!=null)
System.out.println(
new SimpleDateFormat("yyyy-MM-dd HH:mm:ss").format(d));
}
}
@ResponseBody 可以将该方法返回的值 写入到 response的body中去(String,json,xml)。
@RequestMapping 也可以用于整个类
@Controller
@RequestMapping("/emp/*")
public class EmpController {
@RequestMapping("test1") // 这里匹配的url就是/emp/test1.do
public void test1(String s){
System.out.println("s:"+s);
}
}
spring 配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="
http://www.springframework.org/schema/beans
"
xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance
"
xmlns:tx="
http://www.springframework.org/schema/tx
"
xmlns:aop="
http://www.springframework.org/schema/aop
"
xmlns:context="
http://www.springframework.org/schema/context
"
xmlns:jee="
http://www.springframework.org/schema/jee
"
xmlns:mvc="
http://www.springframework.org/schema/mvc
"
xmlns:p="
http://www.springframework.org/schema/p
"
xsi:schemaLocation="
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-2.5.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/jee
http://www.springframework.org/schema/jee/spring-jee-2.5.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
">
<!-- 加载配置文件 -->
<context:property-placeholder location="classpath:config/jdbc.properties" />
<context:component-scan base-package="org.base" />
<!-- 开启基于注解的mvc配置 -->
<mvc:annotation-driven>
<mvc:message-converters>
<bean class="org.springframework.http.converter.json.MappingJacksonHttpMessageConverter">
<!-- 解决"IE下返回json提示下载"问题 -->
<property name="supportedMediaTypes" value="text/plain;charset=UTF-8"></property>
</bean>
</mvc:message-converters>
</mvc:annotation-driven>
<!-- 视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:prefix="/WEB-INF/" p:suffix=".jsp"></bean>
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="
http://java.sun.com/xml/ns/javaee
"
xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance
"
xsi:schemaLocation="
http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd
">
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<!-- spring mvc -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:config/spring.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>
@ResponseBody 可以将该方法返回的值 写入到 response的body中去(String,json,xml)。
@RequestMapping 也可以用于整个类
@Controller
@RequestMapping("/emp/*")
public class EmpController {
@RequestMapping("test1") // 这里匹配的url就是/emp/test1.do
public void test1(String s){
System.out.println("s:"+s);
}
}
spring 配置文件
扫描二维码关注公众号,回复:
8859083 查看本文章
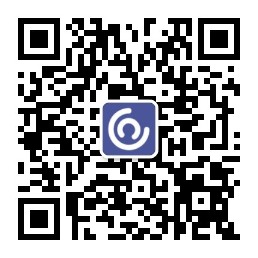
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="
http://www.springframework.org/schema/beans
"
xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance
"
xmlns:tx="
http://www.springframework.org/schema/tx
"
xmlns:aop="
http://www.springframework.org/schema/aop
"
xmlns:context="
http://www.springframework.org/schema/context
"
xmlns:jee="
http://www.springframework.org/schema/jee
"
xmlns:mvc="
http://www.springframework.org/schema/mvc
"
xmlns:p="
http://www.springframework.org/schema/p
"
xsi:schemaLocation="
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx-2.5.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop-2.5.xsd
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-2.5.xsd
http://www.springframework.org/schema/jee
http://www.springframework.org/schema/jee/spring-jee-2.5.xsd
http://www.springframework.org/schema/mvc
http://www.springframework.org/schema/mvc/spring-mvc.xsd
">
<!-- 加载配置文件 -->
<context:property-placeholder location="classpath:config/jdbc.properties" />
<context:component-scan base-package="org.base" />
<!-- 开启基于注解的mvc配置 -->
<mvc:annotation-driven>
<mvc:message-converters>
<bean class="org.springframework.http.converter.json.MappingJacksonHttpMessageConverter">
<!-- 解决"IE下返回json提示下载"问题 -->
<property name="supportedMediaTypes" value="text/plain;charset=UTF-8"></property>
</bean>
</mvc:message-converters>
</mvc:annotation-driven>
<!-- 视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"
p:prefix="/WEB-INF/" p:suffix=".jsp"></bean>
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5"
xmlns="
http://java.sun.com/xml/ns/javaee
"
xmlns:xsi="
http://www.w3.org/2001/XMLSchema-instance
"
xsi:schemaLocation="
http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd
">
<welcome-file-list>
<welcome-file>index.jsp</welcome-file>
</welcome-file-list>
<!-- spring mvc -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:config/spring.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.do</url-pattern>
</servlet-mapping>
</web-app>