首先,实战源码:包括编译好的文件:https://download.csdn.net/download/qq_25490573/11829029
不bb,上启动界面
首先,下载opencv库,在。pro中添加库文件 主要添加的是这两个 路径需要改成自己的
INCLUDEPATH += \
D:\opencv\opencv\build\include \
D:\opencv\opencv\build\include\opencv \
D:\opencv\opencv\build\include\opencv2
LIBS += \
D:/opencv/opencv/build/x64/vc14/lib/opencv_world341.lib \
#-------------------------------------------------
#
# Project created by QtCreator 2019-09-26T14:12:50
#
#-------------------------------------------------
QT += core gui
greaterThan(QT_MAJOR_VERSION, 4): QT += widgets
TARGET = WDMovicePlayer
TEMPLATE = app
# The following define makes your compiler emit warnings if you use
# any feature of Qt which as been marked as deprecated (the exact warnings
# depend on your compiler). Please consult the documentation of the
# deprecated API in order to know how to port your code away from it.
DEFINES += QT_DEPRECATED_WARNINGS
# You can also make your code fail to compile if you use deprecated APIs.
# In order to do so, uncomment the following line.
# You can also select to disable deprecated APIs only up to a certain version of Qt.
#DEFINES += QT_DISABLE_DEPRECATED_BEFORE=0x060000 # disables all the APIs deprecated before Qt 6.0.0
SOURCES += \
main.cpp \
mainwindow.cpp
HEADERS += \
mainwindow.h
FORMS += \
mainwindow.ui
INCLUDEPATH += \
D:\opencv\opencv\build\include \
D:\opencv\opencv\build\include\opencv \
D:\opencv\opencv\build\include\opencv2
LIBS += \
D:/opencv/opencv/build/x64/vc14/lib/opencv_world341.lib \
User32.LIB
RESOURCES += \
recourse.qrc
头文件声明如下:
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
#include "QPainter"
#include "QImage"
#include "QPixmap"
#include "QDebug"
#include <QColor>
#include "QWindow"
#include "Windows.h"
#include "QThread"
#include <QTimer>
#include "QPushButton"
#include "QDesktopWidget"
#include "QFileDialog"
#include "opencv2/core/core.hpp"
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
#include <opencv2/core/core.hpp>
using namespace cv;
namespace Ui {
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
explicit MainWindow(QWidget *parent = 0);
~MainWindow();
QImage MatToQImage(const cv::Mat& mat);
void resizeEvent(QResizeEvent *event);
void paintEvent(QPaintEvent *event); //绘图事件
void onBtnClicked(QString mvpath); //播放事件
private slots:
void onTimeout();//定时器循环
void onBtnFastClicked();
void onBtnSlowClicked();
private:
Ui::MainWindow *ui;
QRect WindowsRect;
VideoCapture capture; //用来读取视频结构
QTimer timer;//视频播放的定时器
QTimer StatueTimer;//提示定时器
int beishu;//调节播放速率
int delay;//帧延迟时间
long MovieProgress =0,MovieAllPro = 0,MoviceNowPro =0;
QString filepath;
void AddEventButton(); //添加自己的按钮事件
void ShowText2Status(QString value,int time = 3000);//状态栏显示文字
};
#endif // MAINWINDOW_H
。cpp文件如下
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include "windows.h";
MainWindow::MainWindow(QWidget *parent) :
QMainWindow(parent),
ui(new Ui::MainWindow)
, beishu(1)
,delay(0)
{
ui->setupUi(this);
this->setWindowFlag(Qt::FramelessWindowHint);
WindowsRect = QApplication::desktop()->screenGeometry();
// this->resize(WindowsRect.width()-600,WindowsRect.height()-400);
this->resize(1440,900);
this->move(200,100);
ui->progressBar->setValue(0);
this->statusBar()->hide();
ui->openfile->move(this->width()/2-ui->openfile->width()/2,this->height()/2-ui->openfile->height()/2);
AddEventButton();
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::onBtnFastClicked()
{
beishu = beishu * 2;
timer.stop();
timer.start(delay / beishu);
}
void MainWindow::onBtnSlowClicked()
{
beishu = beishu / 2;
timer.stop();
timer.start(delay / beishu);
}
void MainWindow::onBtnClicked(QString mvpath)
{
capture.open(mvpath.toStdString());
if (!capture.isOpened())
ShowText2Status("未检测到视频");
ui->moviename->setText(mvpath);
ui->openfile->hide();
MovieAllPro = capture.get(CV_CAP_PROP_FRAME_COUNT);//获取整个帧数
ShowText2Status(QString("整个视频共%1帧\n").arg(MovieAllPro));
// ui->MovieWindow->resize(QSize(capture.get(CV_CAP_PROP_FRAME_WIDTH), capture.get(CV_CAP_PROP_FRAME_HEIGHT)));
//设置开始帧()
long frameToStart = 0;
capture.set(CV_CAP_PROP_POS_FRAMES, frameToStart);
ShowText2Status(QString("从第%1帧开始读").arg(frameToStart));
//获取帧率
double rate = capture.get(CV_CAP_PROP_FPS);
ShowText2Status(QString("帧率为:%1").arg(rate));
delay = 1000 / rate;
timer.start(delay / beishu);
}
void MainWindow::onTimeout()
{
Mat frame;
//读取下一帧
if (!capture.read(frame))
{
ShowText2Status(QString("视频播放完成"));
if(MovieProgress>0){
MovieProgress = 0;
MovieAllPro = 0;
MoviceNowPro = 0;
ui->progressBar->setValue(0);
ui->MovieWindow->setPixmap(QPixmap(":/image/beijing,jpg"));
ui->openfile->show();
ui->moviename->setText("WDMovicePlayer");
}
return;
}
QImage image=MatToQImage(frame);
ui->MovieWindow->setScaledContents(true);
QSize qs = ui->MovieWindow->rect().size();
ui->MovieWindow->setPixmap(QPixmap::fromImage(image).scaled(qs));
ui->MovieWindow->repaint();
//这里加滤波程序
MoviceNowPro = capture.get(CV_CAP_PROP_POS_FRAMES);
MovieProgress = (MoviceNowPro*100/MovieAllPro);
ui->progressBar->setValue((int)MovieProgress);
// ShowText2Status(QString("正在读取第:第 %1 帧\n").arg(totalFrameNumber));
}
void MainWindow::paintEvent(QPaintEvent *event){
if(MovieProgress == 0){
QPainter painter(this);
QPixmap map(":/image/beijing.jpg");
painter.drawPixmap(0,0,this->width(),this->height(),QPixmap("://image/beijing.jpg"));
}
}
void MainWindow::resizeEvent(QResizeEvent *event){
ui->MovieWindow->resize(this->width(),this->height());
int height = ui->statusBar->isSizeGripEnabled()?ui->statusBar->size().height():0;
ui->ManageWidget->resize(this->width(),this->height()-height);
ui->openfile->move(this->width()/2-ui->openfile->width()/2,this->height()/2-ui->openfile->height()/2);
}
void MainWindow::AddEventButton(){
connect(ui->close,&QPushButton::clicked,[=](){
// HWND hWnd = ::FindWindow(L"Shell_TrayWnd",NULL);
// ::SetWindowPos(hWnd,0,0,0,0,0,SWP_SHOWWINDOW);
this->close();
});
connect(ui->maxwindow,&QPushButton::clicked,[=](){
// HWND hWnd = ::FindWindow(L"Shell_TrayWnd",NULL);
// ::SetWindowPos(hWnd,0,0,0,0,0,SWP_HIDEWINDOW);
this->showMaximized();
});
connect(ui->FullWindow,&QPushButton::clicked,[=](){
this->showFullScreen();
});
connect(ui->minwindow,&QPushButton::clicked,[=](){
this->showMinimized();
});
connect(ui->pushButton,&QPushButton::clicked,[=](){
static bool playerstats = true;
if(playerstats){
timer.stop();
}
else{
timer.start();
}
playerstats = !playerstats;
});
connect(ui->pushButton_fast, SIGNAL(clicked()), this, SLOT(onBtnFastClicked()));
connect(ui->pushButton_slow, SIGNAL(clicked()), this, SLOT(onBtnSlowClicked()));
connect(&timer, SIGNAL(timeout()), this, SLOT(onTimeout()));
connect(&StatueTimer,&QTimer::timeout,[=]{
ui->statusBar->hide();
});
connect(ui->openfile,&QPushButton::clicked,[=](){
filepath = QFileDialog::getOpenFileName(this,"WDMovicePlayer","E:/QT/test/WDMovicePlayer/","(*.mp4)");
ShowText2Status(filepath);
onBtnClicked(filepath);
});
}
QImage MainWindow::MatToQImage(const cv::Mat& mat)
{
// 8-bits unsigned, NO. OF CHANNELS = 1
if (mat.type() == CV_8UC1)
{
QImage image(mat.cols, mat.rows, QImage::Format_Indexed8);
// Set the color table (used to translate colour indexes to qRgb values)
image.setColorCount(256);
for (int i = 0; i < 256; i++)
{
image.setColor(i, qRgb(i, i, i));
}
// Copy input Mat
uchar *pSrc = mat.data;
for (int row = 0; row < mat.rows; row++)
{
uchar *pDest = image.scanLine(row);
memcpy(pDest, pSrc, mat.cols);
pSrc += mat.step;
}
return image;
}
// 8-bits unsigned, NO. OF CHANNELS = 3
else if (mat.type() == CV_8UC3)
{
// Copy input Mat
const uchar *pSrc = (const uchar*)mat.data;
// Create QImage with same dimensions as input Mat
QImage image(pSrc, mat.cols, mat.rows, mat.step, QImage::Format_RGB888);
return image.rgbSwapped();
}
else if (mat.type() == CV_8UC4)
{
// Copy input Mat
const uchar *pSrc = (const uchar*)mat.data;
// Create QImage with same dimensions as input Mat
QImage image(pSrc, mat.cols, mat.rows, mat.step, QImage::Format_ARGB32);
return image.copy();
}
else
{
return QImage();
}
}
void MainWindow::ShowText2Status(QString value, int time){
if(value.isEmpty()){
return;
}
ui->statusBar->show();
StatueTimer.stop();
StatueTimer.start(time);
ui->statusBar->showMessage(value,time);
}
界面布局如下:很low,懒得摆了,直接代码对齐,stylesheet随便调的,有想法的可以改一改
。ui文件,知道你也懒,已经备好了
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>MainWindow</class>
<widget class="QMainWindow" name="MainWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>801</width>
<height>584</height>
</rect>
</property>
<property name="windowTitle">
<string>MainWindow</string>
</property>
<property name="styleSheet">
<string notr="true"/>
</property>
<widget class="QWidget" name="centralWidget">
<widget class="QWidget" name="ManageWidget" native="true">
<property name="geometry">
<rect>
<x>9</x>
<y>9</y>
<width>641</width>
<height>281</height>
</rect>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgba(255, 255, 255,0);</string>
</property>
<layout class="QVBoxLayout" name="verticalLayout">
<property name="topMargin">
<number>0</number>
</property>
<property name="bottomMargin">
<number>0</number>
</property>
<item>
<widget class="QWidget" name="MyWindowTile" native="true">
<property name="minimumSize">
<size>
<width>0</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>16777215</width>
<height>30</height>
</size>
</property>
<layout class="QHBoxLayout" name="horizontalLayout_2">
<property name="spacing">
<number>0</number>
</property>
<property name="leftMargin">
<number>0</number>
</property>
<property name="topMargin">
<number>0</number>
</property>
<property name="rightMargin">
<number>0</number>
</property>
<property name="bottomMargin">
<number>0</number>
</property>
<item>
<spacer name="horizontalSpacer_2">
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
<property name="sizeType">
<enum>QSizePolicy::Fixed</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>10</width>
<height>20</height>
</size>
</property>
</spacer>
</item>
<item>
<widget class="QLabel" name="moviename">
<property name="minimumSize">
<size>
<width>0</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>16777215</width>
<height>30</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgba(248, 255, 254, 0);</string>
</property>
<property name="text">
<string>WDMoviePlayer</string>
</property>
</widget>
</item>
<item>
<spacer name="horizontalSpacer">
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>40</width>
<height>20</height>
</size>
</property>
</spacer>
</item>
<item>
<widget class="QWidget" name="widget" native="true">
<property name="minimumSize">
<size>
<width>100</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>100</width>
<height>30</height>
</size>
</property>
<layout class="QHBoxLayout" name="horizontalLayout">
<property name="spacing">
<number>0</number>
</property>
<property name="leftMargin">
<number>0</number>
</property>
<property name="topMargin">
<number>0</number>
</property>
<property name="rightMargin">
<number>0</number>
</property>
<property name="bottomMargin">
<number>0</number>
</property>
<item>
<widget class="QPushButton" name="minwindow">
<property name="minimumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgb(84, 255, 227);</string>
</property>
<property name="text">
<string>min</string>
</property>
</widget>
</item>
<item>
<widget class="QPushButton" name="maxwindow">
<property name="minimumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgb(84, 255, 227);</string>
</property>
<property name="text">
<string>max</string>
</property>
</widget>
</item>
<item>
<widget class="QPushButton" name="close">
<property name="minimumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>30</width>
<height>30</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">background-color: rgb(84, 255, 227);</string>
</property>
<property name="text">
<string>close</string>
</property>
</widget>
</item>
</layout>
</widget>
</item>
</layout>
</widget>
</item>
<item>
<spacer name="verticalSpacer">
<property name="orientation">
<enum>Qt::Vertical</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>20</width>
<height>40</height>
</size>
</property>
</spacer>
</item>
<item>
<widget class="QWidget" name="widget_2" native="true">
<layout class="QHBoxLayout">
<property name="spacing">
<number>0</number>
</property>
<property name="leftMargin">
<number>0</number>
</property>
<property name="topMargin">
<number>0</number>
</property>
<property name="rightMargin">
<number>0</number>
</property>
<property name="bottomMargin">
<number>0</number>
</property>
<item>
<spacer name="horizontalSpacer_5">
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
<property name="sizeType">
<enum>QSizePolicy::Fixed</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>60</width>
<height>20</height>
</size>
</property>
</spacer>
</item>
<item>
<spacer name="horizontalSpacer_4">
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>40</width>
<height>20</height>
</size>
</property>
</spacer>
</item>
<item>
<widget class="QPushButton" name="pushButton_slow">
<property name="minimumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">QPushButton{
background-color: rgb(28, 115, 255);
border-radius:5px;
border:0px;
}
QPushButton:hover{
background-color: rgb(85, 170, 255);
border-radius:5px;
border:0px;
}
QPushButton:pressed{
background-color: rgb(85, 0, 255);
border-radius:5px;
border:0px;
}
</string>
</property>
<property name="text">
<string>减速</string>
</property>
</widget>
</item>
<item>
<widget class="QPushButton" name="pushButton">
<property name="minimumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">QPushButton{
background-color: rgb(28, 115, 255);
border-radius:5px;
border:0px;
}
QPushButton:hover{
background-color: rgb(85, 170, 255);
border-radius:5px;
border:0px;
}
QPushButton:pressed{
background-color: rgb(85, 0, 255);
border-radius:5px;
border:0px;
}
</string>
</property>
<property name="text">
<string>播放</string>
</property>
</widget>
</item>
<item>
<widget class="QPushButton" name="pushButton_fast">
<property name="minimumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">QPushButton{
background-color: rgb(28, 115, 255);
border-radius:5px;
border:0px;
}
QPushButton:hover{
background-color: rgb(85, 170, 255);
border-radius:5px;
border:0px;
}
QPushButton:pressed{
background-color: rgb(85, 0, 255);
border-radius:5px;
border:0px;
}
</string>
</property>
<property name="text">
<string>加快</string>
</property>
</widget>
</item>
<item>
<spacer name="horizontalSpacer_3">
<property name="orientation">
<enum>Qt::Horizontal</enum>
</property>
<property name="sizeHint" stdset="0">
<size>
<width>40</width>
<height>20</height>
</size>
</property>
</spacer>
</item>
<item>
<widget class="QPushButton" name="FullWindow">
<property name="minimumSize">
<size>
<width>60</width>
<height>22</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>60</width>
<height>20</height>
</size>
</property>
<property name="styleSheet">
<string notr="true">QPushButton{
background-color: rgb(28, 115, 255);
border-radius:5px;
border:0px;
}
QPushButton:hover{
background-color: rgb(85, 170, 255);
border-radius:5px;
border:0px;
}
QPushButton:pressed{
background-color: rgb(85, 0, 255);
border-radius:5px;
border:0px;
}
</string>
</property>
<property name="text">
<string>全屏</string>
</property>
</widget>
</item>
</layout>
</widget>
</item>
<item>
<widget class="QProgressBar" name="progressBar">
<property name="minimumSize">
<size>
<width>0</width>
<height>10</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>16777215</width>
<height>10</height>
</size>
</property>
<property name="value">
<number>30</number>
</property>
</widget>
</item>
</layout>
</widget>
<widget class="QLabel" name="MovieWindow">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>501</width>
<height>271</height>
</rect>
</property>
<property name="frameShape">
<enum>QFrame::NoFrame</enum>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QPushButton" name="openfile">
<property name="geometry">
<rect>
<x>240</x>
<y>350</y>
<width>300</width>
<height>60</height>
</rect>
</property>
<property name="minimumSize">
<size>
<width>300</width>
<height>60</height>
</size>
</property>
<property name="maximumSize">
<size>
<width>300</width>
<height>60</height>
</size>
</property>
<property name="font">
<font>
<family>华文行楷</family>
<pointsize>20</pointsize>
</font>
</property>
<property name="styleSheet">
<string notr="true">QPushButton{
background-color: rgb(73, 67, 255);
border-radius:15px;
border:0px;
}
QPushButton:hover{
background-color: rgb(85, 170, 255);
border-radius:15px;
border:0px;
}
QPushButton:pressed{
background-color: rgb(85, 0, 255);
border-radius:15px;
border:0px;
}
</string>
</property>
<property name="text">
<string>打开文件</string>
</property>
</widget>
<zorder>MovieWindow</zorder>
<zorder>ManageWidget</zorder>
<zorder>openfile</zorder>
</widget>
<widget class="QStatusBar" name="statusBar"/>
</widget>
<layoutdefault spacing="6" margin="11"/>
<resources/>
<connections/>
</ui>
最后运行起来,看一下效果
扫描二维码关注公众号,回复:
8876137 查看本文章
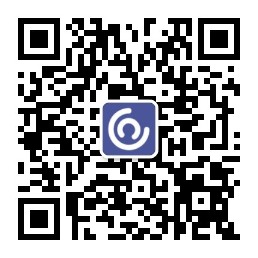