Given a m * n
matrix mat
of integers, sort it diagonally in ascending order from the top-left to the bottom-right then return the sorted array.
Example 1:
Input: mat = [[3,3,1,1],[2,2,1,2],[1,1,1,2]] Output: [[1,1,1,1],[1,2,2,2],[1,2,3,3]]
Constraints:
m == mat.length
n == mat[i].length
1 <= m, n <= 100
1 <= mat[i][j] <= 100
class Solution { public int[][] diagonalSort(int[][] mat) { int m = mat.length, n = mat[0].length; Map<Integer, PriorityQueue<Integer>> map = new HashMap(); for(int i = 0; i < m; i++){ for(int j = 0; j < n; j++){ map.putIfAbsent(i-j, new PriorityQueue()); map.get(i - j).offer(mat[i][j]); } } for(int i = 0; i < m; i++){ for(int j = 0; j < n; j++){ mat[i][j] = map.get(i - j).poll(); } } return mat; } }
putIfAbsent,如果key不存在就put,存在就不用管
对角线有什么特性?
下表相减相同 -- i - j。
什么东西能自动排序?
PriorityQueue
扫描二维码关注公众号,回复:
8916174 查看本文章
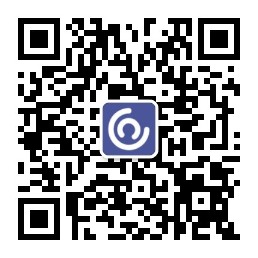