jedis 基本操作
jedis
因为jedis 对实际开发已经没有任何意义了,基本上都是用 spring data redis 的 api。
spring data redis
spring-data-reids 提供了spring应用中通过简单的配置访问redis服务,对redis底层开发包进行了高度封装
redisTemplate 提供了redis各种操作,异常处理序列化
- 连接池自动管理,提供了一个高度封装的 redisTemplate 类
- 针对jedis 客户端中大量api 进行了归类封装,将同一类型操作封装为operation 接口。
和springboot整合
后续整理
redisTemplate 使用
和 redisTemplate 一样的还有StringRedisTemplate,StringRedisTemplate 是继承 redisTemplate ,但两者的数据是不共通的
。
redisTemplate 常用的操作
- bound***Ops
- opsFor***
- delete
主要开发中用于数据的存取,和修改,参考以下
redis 的集中基本数据类型使用
@Test
public void testString(){
// 实例化一个对象
BoundValueOperations<String, Serializable> value = redisTemplate.boundValueOps("name");
// set 添加
value.set("lisi");
// get 查找
Serializable name = value.get();
System.out.println("当前的姓名"+ name);
// append (测试下来,并没有往value 后面添加值,所以自己也不确定是否用错)
value.append(" is a bad man"); // 返回值是 Integer 类型
Serializable newName = value.get();
System.out.println("当前键:"+ value.getKey() + ", 当前姓名:" + newName); // 当前键:name, 当前姓名:lisi
// set 添加超时时间
value.set("wangwu",6,TimeUnit.HOURS);
System.out.println("被修改的值:"+value.get()); // 被修改的值:wangwu
//Set the bound key to hold the string value if the bound key is absent.
//如果绑定键不存在,则设置绑定键来保存字符串值
System.out.println("绑定键是否存在:"+value.setIfAbsent("haha")); // false
//Set the bound key to hold the string value if key is present.
//如果键存在,则设置绑定键来保存字符串值。
System.out.println("绑定键是否存在:"+value.setIfPresent("zhangsan"));
// System.out.println("绑定键是否存在:"+value.setIfPresent("wangwu"));
System.out.println("现在的值:"+value.get()); //zhangsan
}
// Hash 类型操作
@Test
public void testHash(){
// 实例化一个 Hash 对象
BoundHashOperations<String, Object, Object> person = redisTemplate.boundHashOps("person");
//设置值
Map map = new HashMap();
map.put("name","lisi");
map.put("age",3);
map.put("sex","man");
person.putAll(map);
//获取
Map<Object, Object> entries = person.entries();
System.out.println(entries);
// 单个key
Object name = person.get("name");
System.out.println("name:"+name);
//hasKey 是否存在
System.out.println(person.hasKey("name"));
// 所有key
System.out.println(person.keys());
// 获取大于1 的key
List list = new ArrayList();
list.add("name");
list.add("sex");
List personValuelist = person.multiGet(list);
System.out.println(personValuelist);
// 判断key是否存在,不存在则添加
person.putIfAbsent("loveGirl","haha");
// values 获取所有值
System.out.println(person.values());//[haha, 3, lisi, man]
}
//测试List
@Test
public void testList(){
redisTemplate.delete("fruits");
BoundListOperations<String, Serializable> fruits = redisTemplate.boundListOps("fruits");
fruits.leftPush("apple");
fruits.leftPush("banana");
System.out.println(fruits.getOperations()); // org.springframework.data.redis.core.RedisTemplate@4e212f46
// 返回整个数组
System.out.println("返回所有数组:"+fruits.range(0,-1));
//左边移掉一个
System.out.println( fruits.leftPop()); // 返回移掉的值
// key 存在就添加
System.out.println(fruits.leftPushIfPresent("apple"));
System.out.println(fruits.leftPushIfPresent("banana"));
System.out.println("返回所有数组:"+fruits.range(0,-1)); //返回所有数组:[banana, apple, apple]
// 移除 2 个 apple
fruits.remove(2,"apple");
System.out.println("返回所有数组:"+fruits.range(0,-1)); // 返回所有数组:[banana, apple]
}
// set 值不可重复
@Test
public void testSet(){
redisTemplate.delete("fruits");
redisTemplate.delete("sweetfruits");
BoundSetOperations<String, Serializable> fruits = redisTemplate.boundSetOps("fruits");
BoundSetOperations<String, Serializable> sweetfruits = redisTemplate.boundSetOps("sweetfruits");
// 添加
fruits.add("apple","pineapple");
fruits.add("banana");
fruits.add("apple");
sweetfruits.add("apple");
// 获取
// Set<Serializable> members = fruits.members();
// System.out.println("fruits 的集合:"+members);// [pineapple, banana, apple]
//比较不同 diff(Collection<K> keys) , diff(K key) 返回比较中sweetfruits没有的值而fruits 中有的值
// System.out.println("fruits 的 diff :"+ fruits.diff( "sweetfruits")); // [pineapple, banana]
sweetfruits.add("kiwi fruit");
// System.out.println("sweetfruits 的集合:"+ sweetfruits.members());// [kiwi fruit, apple]
//diffAndStore(Collection<K> keys, K destKey)
BoundSetOperations<String, Serializable> difffruits = redisTemplate.boundSetOps("difffruits");
fruits.diffAndStore("fruits","sweetfruits");
redisTemplate.opsForSet().members("sweetfruits").forEach(v -> System.out.println("比较不同set并存储:" + v));
System.out.println("difffruits 的集合:" + difffruits.members());
System.out.println("sweetfruits 的集合:" + sweetfruits.members());// [] , 会把后面的函数清空
System.out.println("fruits 的集合:" + fruits.members());
}
注解式使用
开启基于注解的缓存 @EnableCaching
一些缓存注解
@Cacheable
// key 生存策略,参考下图
@Cacheable(cacheNames = "test", key ="#id" )
public Person get(Long id){
// ...
}
上述就已经完成了spring本地化的缓存
扫描二维码关注公众号,回复:
8988041 查看本文章
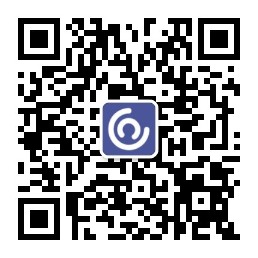
自定义生成key 策略的实现
@Cacheable(cacheNames = "test", keyGenerator ="myKeyGenerate" )
@Configuration
public class MyCacheConfig {
@Bean("myKeyGenerate")
public KeyGenerator myKeyGenerate(){
return new KeyGenerator() {
@Override
public Object generate(Object o, Method method, Object... objects) {
return method.getName() +"["+ objects[0].toString()+"]";
}
};
}
}
缓存中用到的spel表达式
@CachePut
修改了数据库的某个数据,同时更新缓存
@CachePut(cacheNames = "test", key ="#test.id")
@Override
public Test update(Test test) {
testMapper.updateById(test);
return test;
}
@CacheEvict
删除缓存中的数据
@CacheEvict(cacheNames = "test",key = "#id") // beforeInvocation 无论是否有异常,都会清除缓存
@Override
public void del(Long id) {
System.out.println("将要删除的:"+id);
}
@Caching
复合注解(实际开发中,暂时没用到过)
@Target({ElementType.TYPE, ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Inherited
@Documented
public @interface Caching {
Cacheable[] cacheable() default {};
CachePut[] put() default {};
CacheEvict[] evict() default {};
}
@CacheConfig
抽取缓存公共的配置
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface CacheConfig {
String[] cacheNames() default {};
String keyGenerator() default "";
String cacheManager() default "";
String cacheResolver() default "";
}
结合redis 使用
加入redis 后,发现spring 自动使用了redis 缓存了。
springboot2.0+
实现注解存放redis中序列化和反序列化
//缓存管理器
@Bean
public CacheManager cacheManager(RedisConnectionFactory redisConnectionFactory) {
RedisCacheConfiguration config = RedisCacheConfiguration.defaultCacheConfig()
.entryTtl(Duration.ZERO)
.serializeKeysWith(RedisSerializationContext.SerializationPair.fromSerializer(keySerializer()))
.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(valueSerializer()))
.disableCachingNullValues();
RedisCacheManager redisCacheManager = RedisCacheManager.builder(redisConnectionFactory)
.cacheDefaults(config)
.transactionAware()
.build();
return redisCacheManager;
}