URL详解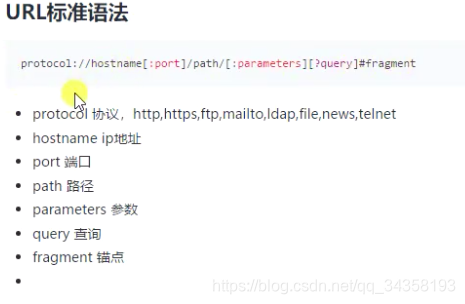
URL匹配
./website/website/urls.py
from django.urls import path, include
from book import urls
urlpatterns = [
# path('admin/', admin.site.urls),
path('index/', include(urls)),
# path('', view s.index),
]
./website/book/urls.py
from django.urls import path, include
from . import views
from news import urls
urlpatterns = [
path('', views.index),
path('web/', views.web),
]
./website/book/views.py
from django.shortcuts import render
from django.http import HttpResponse
# Create your views here.
def index(request):
html = '<h1 style="color:red;">Hello World</h1>'
return HttpResponse(html)
def web(request):
html = '<h1">Django Web</h1>'
return HttpResponse(html)
http://127.0.0.1:8080/index
显示:hello word,
因为./website/website/urls.py中的path为‘index’而./website/book/urls.py中path第一个地址为空。
http://127.0.0.1:8080/index/web
显示Django web,
因为./website/book/urls.py中path第二个地址为‘web/’
再次启动这个项目则在manage.py所在的路径下在终端执行:
python manage.py runserver
URL路径转换器
初次尝试路径转换器—默认str
./website/website/urls.py
from django.urls import path, include
from book import urls
urlpatterns = [
path('', include(urls)),
]
./website/book/urls.py
from django.urls import path, include
from . import views
urlpatterns = [
path('<str:html>', views.index, name='news'),
#浏览器输入:http://127.0.0.1:8000/str,页面则显示对应的str
]
./website/book/views.py
from django.http import HttpResponse
def index(request,html):
return HttpResponse('<h1>{}</h1>'.format(html))
int
./website/book/urls.py
urlpatterns = [
path('<int:page>',views.page,name='page'),
path('<int:numa>/<int:numb>',views.sum,name='sum'),
]
./website/book/views.py
def page(request,page):
return HttpResponse('<h1>{}</h1>'.format(page))
#浏览器输入:http://127.0.0.1:8000/2,页面则显示对应的2。
def sum(request,numa,numb):
return HttpResponse('<h1>{}</h1>'.format(numa+numb))
#浏览器输入:http://127.0.0.1:8000/2/6,页面则显示对应的6。
slug
./website/book/urls.py
path('<slug:slugStr>',views.slugStr,name='slugStr'),
./website/book/views.py
def slugStr(request,slugStr):
return HttpResponse('<h1>{}</h1>'.format(slugStr))
#浏览器输入:http://127.0.0.1:8000/swe-123;lkl-=,页面则显示对应的swe-123;lkl-=
uuid
./website/book/urls.py
path('<uuid:uu>/<int:numb>',views.uu,name='uu'),
./website/book/views.py
def uu(request,uu):
return HttpResponse('<h1>{}</h1>'.format(uu))
#浏览器输入:http://127.0.0.1:8000/uuid码,页面则显示对应的uuid码。如:5acba24c-abdd-4ee1-b991-a90d3406d2a8
path (和str有不同)
./website/book/urls.py
path('<path:home>',views.home,name='home'),
./website/book/views.py
def home(request,home):
return HttpResponse('<h1>{}</h1>'.format(home))
#浏览器输入:http://127.0.0.1:8000/html/index.html,页面则显示对应的html/index.html
和str不同:如果没有path
则在浏览器输入http://127.0.0.1:8000/html/html.index会报错,如图:
URL-include
在上面初试路径转换器的时候已经使用了。
第一种:
include(module,namespace=None)
第二种:
include(pattern_list)
第三种:
include((pattern_list,app_namespace),namespace=None)
module – 表示一个模型文件
namespace—实例命名空间
pattern_list—必须是一个可迭代的path()或re_path()清单
app_namespace—app命名空间
./websites/news/urls.py
extractpatterns = [
path('', views.index,name='index'),
path('index/', views.index,name='index'),
path('home/', views.index,name='index'),
]
urlpatterns = [
#第一种最常用
path('', views.index,name='index'),
path('index/', include(extractpatterns),name='index'),
path('index/', include((extractpatterns, 'newsapp')),name='index'),
]
URL-命名空间
./websites/news/urls.py
urlpatterns = [
path('', views.index,name='index'),
path('news/', views.news,name='news'),
path('video/', views.video,name='video'),
#将video修改成movies,只是显示链接地址变了,实际上链接指向的页面没有变。
]
./websites/news/views.py
def index(request):
return render(request,'index.html')
def news(request):
return HttpResponse('我是新闻的页面首页')
def video(request):
return HttpResponse('我是视频的页面首页')
./websites/news/template/index.html
<p><a href={% url 'index' %}>index</a></p>
<p><a href={% url 'news' %}>news</a></p>
<p><a href={% url 'video' %}>video</a></p>
URL-获取查询关键字
参考B站视频:https://www.bilibili.com/video/av48618712/?p=20