一、@Target
如果一个Annotation 没有明确地指明定义的位置,则可以在任意的位置使用,例如之
前所讲解的全部的 Annotation 因为没有指定应用位置,所以可以在任意位置上进行定义。
@MyDefaultAnnotationReflect (key = "J1", value = "J2")
public class SimpleBean {
//使用自定义的Annotation并设置两个属性内容
@MyDefaultAnnotationReflect (key = "J3", value = "J4")
public String toString() {
//覆写toString()方法
return "Hello World" ;
}
}
以上定义的 MyDefaultAnnotationReflect 可以在方法上声明,也可以在类上声明;
而如果希望一个自定义的Annotation 只能在指定的位置上出现,例如,只能在类上或只能在方法中声明,则必须使用@Target
注释。
@Target 明确地指出了一个Annotation的使用位置,此注释的定义如下:
@Documented
@Retention(value = RetentionPolicy.RUNTIME)
@Target(value = ElementType.ANNOTATION_TYPE)
public @interface Target {
public abstract ElementType[] value
}
下面定义一个 Annotation,此 Annotation 只能用在类上,不能用在方法中:
定义一个 Annotation 可以出现在类、方法上:
@Target({ElementType.TYPE,ElementType.METHOD}) //此 Annotation 只能用在类上及方法上
@Retention(value = RetentionPolicy.RUNTIME)//此 Annotation 在类执行时依然有效
@interface MyTargetAnnotation{
public String key() default "J1";
public String value() default "J2";
}
二、@Documented 注释
任何一个自定义的 Annotation 实际上都是通过 @Documented 进行注释的,在生成
javadoc 时可以通过 @Documented 将一些文档的说明信息写入。@Documented 的使用格式:
@Documented
[public] @interface Annotation名称{
数据类型 变量名称();
}
使用完整格式定义 Annotation:
@Documented
@interface MyDocumentedAnnotation{
public String key();
public String value();
}
使用 @Documented 注释后,此 Annotation 在生成 java doc 时就可以加入类或方法的一些说明信息,这样可以便于用户了解类或方法的作用
对定义的方法进行 javadoc 注释:
@MyDocumentedAnnotation(key="J1",value="J2")
public class SimpleBeanDocumented{
//此方法在对象输出时调用,返回对象的信息
@MyDocumentedAnnotation(key="J3",value="J4")
public String toString(){
return "Hello World !!!";
}
}
在命令行中执行:
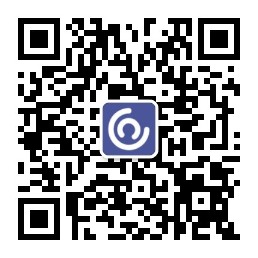
javadoc -d doc SimpleBeanDocumented.java
打开 index.html 可以查看之前编写好的 java doc
三、@Inherited 注释
@Inherited 用于标注一个父类的注释是否可以被子类所继承,如果一个 Annotation 需要被其子类所继承,则在声明时直接使用 @Inherited 注释即可
Inherited 的 Annotation 定义如下:
@Documented
@Retention(value = RetentionPolicy.RUNTIME)
@Target(value = ElementType.ANNOTATION_TYPE)
public @interface Inherited{
}
定义一个 Annotation,观察 Inherited 的作用:
import java.lang.annotation.*;
@Documented
@Inherited//此 Annotation 可以被继承
@Retention(value = RetentionPolicy.RUNTIME)
@interface MyInheritedAnnotation{
public String name();
}
//定义 Person 类
@MyInheritedAnnotation(name = "Java")
class Person{
}
class Student extends Person{//继承 Person类
}
public class Test{
public static void main(String[] args) throws Exception {
Class<?> c = null;
c = Class.forName("Student");
Annotation ann[] = c.getAnnotations();
for (Annotation a:ann){//输出全部的 Annotation
System.out.println(a);
}
if (c.isAnnotationPresent(MyInheritedAnnotation.class)){
MyInheritedAnnotation mda = null;//声明自定义的 Annotation
// 取得自定义的 Annotation,此 Annotation是从父类继承而来
mda = c.getAnnotation(MyInheritedAnnotation.class);
String name = mda.name();//得到自定义的 Annotation 指定变量内容
System.out.println("name = " + name);
}
}
}
这里如果在声明 Annotation 时,没有加上 @Inherited 注释,则此 Annotation 是无法被继承的